
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
interp.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __INTERP_H__ 00010 #define __INTERP_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief VM Interpreter 00016 * 00017 * VM interpreter header. 00018 */ 00019 00020 00021 #include "thread.h" 00022 00023 00024 #define INTERP_LOOP_FOREVER 0 00025 #define INTERP_RETURN_ON_NO_THREADS 1 00026 00027 00028 /** Frame pointer ; currently for single thread */ 00029 #define PM_FP (gVmGlobal.pthread->pframe) 00030 /** Instruction pointer */ 00031 #define PM_IP (PM_FP->fo_ip) 00032 /** Argument stack pointer */ 00033 #define PM_SP (PM_FP->fo_sp) 00034 00035 /** top of stack */ 00036 #define TOS (*(PM_SP - 1)) 00037 /** one under TOS */ 00038 #define TOS1 (*(PM_SP - 2)) 00039 /** two under TOS */ 00040 #define TOS2 (*(PM_SP - 3)) 00041 /** three under TOS */ 00042 #define TOS3 (*(PM_SP - 4)) 00043 /** index into stack; 0 is top, 1 is next */ 00044 #define STACK(n) (*(PM_SP - ((n) + 1))) 00045 /** pops an obj from the stack */ 00046 #define PM_POP() (*(--PM_SP)) 00047 /** pushes an obj on the stack */ 00048 #define PM_PUSH(pobj) (*(PM_SP++) = (pobj)) 00049 /** gets the argument (S16) from the instruction stream */ 00050 #define GET_ARG() mem_getWord(PM_FP->fo_memspace, &PM_IP) 00051 00052 /** pushes an obj in the only stack slot of the native frame */ 00053 #define NATIVE_SET_TOS(pobj) (gVmGlobal.nativeframe.nf_stack = \ 00054 (pobj)) 00055 /** gets the nth local var from the native frame locals */ 00056 #define NATIVE_GET_LOCAL(n) (gVmGlobal.nativeframe.nf_locals[n]) 00057 /** gets a pointer to the frame that called this native fxn */ 00058 #define NATIVE_GET_PFRAME() (*ppframe) 00059 /** gets the number of args passed to the native fxn */ 00060 #define NATIVE_GET_NUM_ARGS() (gVmGlobal.nativeframe.nf_numlocals) 00061 00062 00063 /** 00064 * COMPARE_OP enum. 00065 * Used by the COMPARE_OP bytecode to determine 00066 * which type of compare to perform. 00067 * Must match those defined in Python. 00068 */ 00069 typedef enum PmCompare_e 00070 { 00071 COMP_LT = 0, /**< less than */ 00072 COMP_LE, /**< less than or equal */ 00073 COMP_EQ, /**< equal */ 00074 COMP_NE, /**< not equal */ 00075 COMP_GT, /**< greater than */ 00076 COMP_GE, /**< greater than or equal */ 00077 COMP_IN, /**< is in */ 00078 COMP_NOT_IN, /**< is not in */ 00079 COMP_IS, /**< is */ 00080 COMP_IS_NOT, /**< is not */ 00081 COMP_EXN_MATCH /**< do exceptions match */ 00082 } PmCompare_t, *pPmCompare_t; 00083 00084 /** 00085 * Byte code enumeration 00086 */ 00087 typedef enum PmBcode_e 00088 { 00089 #ifdef HAVE_PYTHON27 00090 STOP_CODE=0, /* 0x00 */ 00091 POP_TOP=1, /* 0x01 */ 00092 ROT_TWO=2, /* 0x02 */ 00093 ROT_THREE=3, /* 0x03 */ 00094 DUP_TOP=4, /* 0x04 */ 00095 ROT_FOUR=5, /* 0x05 */ 00096 UNUSED_06=6, 00097 UNUSED_07=7, 00098 UNUSED_08=8, 00099 NOP=9, /* 0x09 */ 00100 UNARY_POSITIVE=10, /* 0x0a */ 00101 UNARY_NEGATIVE=11, /* 0x0b */ 00102 UNARY_NOT=12, /* 0x0c */ 00103 UNARY_CONVERT=13, /* 0x0d */ 00104 UNUSED_0E=14, 00105 UNARY_INVERT=15, /* 0x0f */ 00106 UNUSED_10=16, 00107 UNUSED_11=17, 00108 UNUSED_12=18, 00109 BINARY_POWER=19, /* 0x13 */ 00110 BINARY_MULTIPLY=20, /* 0x14 */ 00111 BINARY_DIVIDE=21, /* 0x15 */ 00112 BINARY_MODULO=22, /* 0x16 */ 00113 BINARY_ADD=23, /* 0x17 */ 00114 BINARY_SUBTRACT=24, /* 0x18 */ 00115 BINARY_SUBSCR=25, /* 0x19 */ 00116 BINARY_FLOOR_DIVIDE=26, /* 0x1a */ 00117 BINARY_TRUE_DIVIDE=27, /* 0x1b */ 00118 INPLACE_FLOOR_DIVIDE=28, /* 0x1c */ 00119 INPLACE_TRUE_DIVIDE=29, /* 0x1d */ 00120 SLICE_0=30, /* 0x1e */ 00121 SLICE_1=31, /* 0x1f */ 00122 SLICE_2=32, /* 0x20 */ 00123 SLICE_3=33, /* 0x21 */ 00124 UNUSED_22=34, 00125 UNUSED_23=35, 00126 UNUSED_24=36, 00127 UNUSED_25=37, 00128 UNUSED_26=38, 00129 UNUSED_27=39, 00130 STORE_SLICE_0=40, /* 0x28 */ 00131 STORE_SLICE_1=41, /* 0x29 */ 00132 STORE_SLICE_2=42, /* 0x2a */ 00133 STORE_SLICE_3=43, /* 0x2b */ 00134 UNUSED_2C=44, 00135 UNUSED_2D=45, 00136 UNUSED_2E=46, 00137 UNUSED_2F=47, 00138 UNUSED_30=48, 00139 UNUSED_31=49, 00140 DELETE_SLICE_0=50, /* 0x32 */ 00141 DELETE_SLICE_1=51, /* 0x33 */ 00142 DELETE_SLICE_2=52, /* 0x34 */ 00143 DELETE_SLICE_3=53, /* 0x35 */ 00144 STORE_MAP=54, /* 0x36 */ 00145 INPLACE_ADD=55, /* 0x37 */ 00146 INPLACE_SUBTRACT=56, /* 0x38 */ 00147 INPLACE_MULTIPLY=57, /* 0x39 */ 00148 INPLACE_DIVIDE=58, /* 0x3a */ 00149 INPLACE_MODULO=59, /* 0x3b */ 00150 STORE_SUBSCR=60, /* 0x3c */ 00151 DELETE_SUBSCR=61, /* 0x3d */ 00152 BINARY_LSHIFT=62, /* 0x3e */ 00153 BINARY_RSHIFT=63, /* 0x3f */ 00154 BINARY_AND=64, /* 0x40 */ 00155 BINARY_XOR=65, /* 0x41 */ 00156 BINARY_OR=66, /* 0x42 */ 00157 INPLACE_POWER=67, /* 0x43 */ 00158 GET_ITER=68, /* 0x44 */ 00159 UNUSED_45=69, 00160 PRINT_EXPR=70, /* 0x46 */ 00161 PRINT_ITEM=71, /* 0x47 */ 00162 PRINT_NEWLINE=72, /* 0x48 */ 00163 PRINT_ITEM_TO=73, /* 0x49 */ 00164 PRINT_NEWLINE_TO=74, /* 0x4a */ 00165 INPLACE_LSHIFT=75, /* 0x4b */ 00166 INPLACE_RSHIFT=76, /* 0x4c */ 00167 INPLACE_AND=77, /* 0x4d */ 00168 INPLACE_XOR=78, /* 0x4e */ 00169 INPLACE_OR=79, /* 0x4f */ 00170 BREAK_LOOP=80, /* 0x50 */ 00171 WITH_CLEANUP=81, /* 0x51 */ 00172 LOAD_LOCALS=82, /* 0x52 */ 00173 RETURN_VALUE=83, /* 0x53 */ 00174 IMPORT_STAR=84, /* 0x54 */ 00175 EXEC_STMT=85, /* 0x55 */ 00176 YIELD_VALUE=86, /* 0x56 */ 00177 POP_BLOCK=87, /* 0x57 */ 00178 END_FINALLY=88, /* 0x58 */ 00179 BUILD_CLASS=89, /* 0x59 */ 00180 00181 /* Opcodes from here have an argument */ 00182 HAVE_ARGUMENT=90, /* 0x5a */ 00183 STORE_NAME=90, /* 0x5a */ 00184 DELETE_NAME=91, /* 0x5b */ 00185 UNPACK_SEQUENCE=92, /* 0x5c */ 00186 FOR_ITER=93, /* 0x5d */ 00187 LIST_APPEND=94, /* 0x5e */ 00188 STORE_ATTR=95, /* 0x5f */ 00189 DELETE_ATTR=96, /* 0x60 */ 00190 STORE_GLOBAL=97, /* 0x61 */ 00191 DELETE_GLOBAL=98, /* 0x62 */ 00192 DUP_TOPX=99, /* 0x63 */ 00193 LOAD_CONST=100, /* 0x64 */ 00194 LOAD_NAME=101, /* 0x65 */ 00195 BUILD_TUPLE=102, /* 0x66 */ 00196 BUILD_LIST=103, /* 0x67 */ 00197 BUILD_SET=104, /* 0x68 */ 00198 BUILD_MAP=105, /* 0x69 */ 00199 LOAD_ATTR=106, /* 0x6a */ 00200 COMPARE_OP=107, /* 0x6b */ 00201 IMPORT_NAME=108, /* 0x6c */ 00202 IMPORT_FROM=109, /* 0x6d */ 00203 JUMP_FORWARD=110, /* 0x6e */ 00204 JUMP_IF_FALSE_OR_POP=111, /* 0x6f */ 00205 JUMP_IF_TRUE_OR_POP=112, /* 0x70 */ 00206 JUMP_ABSOLUTE=113, /* 0x71 */ 00207 POP_JUMP_IF_FALSE=114, /* 0x72 */ 00208 POP_JUMP_IF_TRUE=115, /* 0x73 */ 00209 LOAD_GLOBAL=116, /* 0x74 */ 00210 UNUSED_75=117, 00211 UNUSED_76=118, 00212 CONTINUE_LOOP=119, /* 0x77 */ 00213 SETUP_LOOP=120, /* 0x78 */ 00214 SETUP_EXCEPT=121, /* 0x79 */ 00215 SETUP_FINALLY=122, /* 0x7a */ 00216 UNUSED_7B=123, 00217 LOAD_FAST=124, /* 0x7c */ 00218 STORE_FAST=125, /* 0x7d */ 00219 DELETE_FAST=126, /* 0x7e */ 00220 UNUSED_7F=127, 00221 UNUSED_80=128, 00222 UNUSED_81=129, 00223 RAISE_VARARGS=130, /* 0x82 */ 00224 CALL_FUNCTION=131, /* 0x83 */ 00225 MAKE_FUNCTION=132, /* 0x84 */ 00226 BUILD_SLICE=133, /* 0x85 */ 00227 MAKE_CLOSURE=134, /* 0x86 */ 00228 LOAD_CLOSURE=135, /* 0x87 */ 00229 LOAD_DEREF=136, /* 0x88 */ 00230 STORE_DEREF=137, /* 0x89 */ 00231 UNUSED_8A=138, 00232 UNUSED_8B=139, 00233 CALL_FUNCTION_VAR=140, /* 0x8c */ 00234 CALL_FUNCTION_KW=141, /* 0x8d */ 00235 CALL_FUNCTION_VAR_KW=142, /* 0x8e */ 00236 SETUP_WITH=143, /* 0x8f */ 00237 UNUSED_90=144, 00238 EXTENDED_ARG=145, /* 0x91 */ 00239 SET_ADD=146, /* 0x92 */ 00240 MAP_ADD=147, /* 0x93 */ 00241 UNUSED_94=148, 00242 UNUSED_95=149, 00243 UNUSED_96=150, 00244 UNUSED_97=151, 00245 UNUSED_98=152, 00246 UNUSED_99=153, 00247 UNUSED_9A=154, 00248 UNUSED_9B=155, 00249 UNUSED_9C=156, 00250 UNUSED_9D=157, 00251 UNUSED_9E=158, 00252 UNUSED_9F=159, 00253 UNUSED_A0=160, 00254 UNUSED_A1=161, 00255 UNUSED_A2=162, 00256 UNUSED_A3=163, 00257 UNUSED_A4=164, 00258 UNUSED_A5=165, 00259 UNUSED_A6=166, 00260 UNUSED_A7=167, 00261 UNUSED_A8=168, 00262 UNUSED_A9=169, 00263 UNUSED_AA=170, 00264 UNUSED_AB=171, 00265 UNUSED_AC=172, 00266 UNUSED_AD=173, 00267 UNUSED_AE=174, 00268 UNUSED_AF=175, 00269 UNUSED_B0=176, 00270 UNUSED_B1=177, 00271 UNUSED_B2=178, 00272 UNUSED_B3=179, 00273 UNUSED_B4=180, 00274 UNUSED_B5=181, 00275 UNUSED_B6=182, 00276 UNUSED_B7=183, 00277 UNUSED_B8=184, 00278 UNUSED_B9=185, 00279 UNUSED_BA=186, 00280 UNUSED_BB=187, 00281 UNUSED_BC=188, 00282 UNUSED_BD=189, 00283 UNUSED_BE=190, 00284 UNUSED_BF=191, 00285 UNUSED_C0=192, 00286 UNUSED_C1=193, 00287 UNUSED_C2=194, 00288 UNUSED_C3=195, 00289 UNUSED_C4=196, 00290 UNUSED_C5=197, 00291 UNUSED_C6=198, 00292 UNUSED_C7=199, 00293 UNUSED_C8=200, 00294 UNUSED_C9=201, 00295 UNUSED_CA=202, 00296 UNUSED_CB=203, 00297 UNUSED_CC=204, 00298 UNUSED_CD=205, 00299 UNUSED_CE=206, 00300 UNUSED_CF=207, 00301 UNUSED_D0=208, 00302 UNUSED_D1=209, 00303 UNUSED_D2=210, 00304 UNUSED_D3=211, 00305 UNUSED_D4=212, 00306 UNUSED_D5=213, 00307 UNUSED_D6=214, 00308 UNUSED_D7=215, 00309 UNUSED_D8=216, 00310 UNUSED_D9=217, 00311 UNUSED_DA=218, 00312 UNUSED_DB=219, 00313 UNUSED_DC=220, 00314 UNUSED_DD=221, 00315 UNUSED_DE=222, 00316 UNUSED_DF=223, 00317 UNUSED_E0=224, 00318 UNUSED_E1=225, 00319 UNUSED_E2=226, 00320 UNUSED_E3=227, 00321 UNUSED_E4=228, 00322 UNUSED_E5=229, 00323 UNUSED_E6=230, 00324 UNUSED_E7=231, 00325 UNUSED_E8=232, 00326 UNUSED_E9=233, 00327 UNUSED_EA=234, 00328 UNUSED_EB=235, 00329 UNUSED_EC=236, 00330 UNUSED_ED=237, 00331 UNUSED_EE=238, 00332 UNUSED_EF=239, 00333 UNUSED_F0=240, 00334 UNUSED_F1=241, 00335 UNUSED_F2=242, 00336 UNUSED_F3=243, 00337 UNUSED_F4=244, 00338 UNUSED_F5=245, 00339 UNUSED_F6=246, 00340 UNUSED_F7=247, 00341 UNUSED_F8=248, 00342 UNUSED_F9=249, 00343 UNUSED_FA=250, 00344 UNUSED_FB=251, 00345 UNUSED_FC=252, 00346 UNUSED_FD=253, 00347 UNUSED_FE=254, 00348 UNUSED_FF=255, 00349 #else /* HAVE_PYTHON27 */ 00350 /* 00351 * Python source to create this list: 00352 * import dis 00353 * o = dis.opname 00354 * for i in range(256): 00355 * if o[i][0] != '<': 00356 * print "\t%s," % o[i] 00357 * else: 00358 * print "\tUNUSED_%02X," % i 00359 */ 00360 STOP_CODE = 0, /* 0x00 */ 00361 POP_TOP, 00362 ROT_TWO, 00363 ROT_THREE, 00364 DUP_TOP, 00365 ROT_FOUR, 00366 UNUSED_06, 00367 UNUSED_07, 00368 UNUSED_08, 00369 NOP, 00370 UNARY_POSITIVE, /* d010 */ 00371 UNARY_NEGATIVE, 00372 UNARY_NOT, 00373 UNARY_CONVERT, 00374 UNUSED_0E, 00375 UNARY_INVERT, 00376 UNUSED_10, /* 0x10 */ 00377 UNUSED_11, 00378 LIST_APPEND, 00379 BINARY_POWER, 00380 BINARY_MULTIPLY, /* d020 */ 00381 BINARY_DIVIDE, 00382 BINARY_MODULO, 00383 BINARY_ADD, 00384 BINARY_SUBTRACT, 00385 BINARY_SUBSCR, 00386 BINARY_FLOOR_DIVIDE, 00387 BINARY_TRUE_DIVIDE, 00388 INPLACE_FLOOR_DIVIDE, 00389 INPLACE_TRUE_DIVIDE, 00390 SLICE_0, /* d030 */ 00391 SLICE_1, 00392 SLICE_2, /* 0x20 */ 00393 SLICE_3, 00394 UNUSED_22, 00395 UNUSED_23, 00396 UNUSED_24, 00397 UNUSED_25, 00398 UNUSED_26, 00399 UNUSED_27, 00400 STORE_SLICE_0, /* d040 */ 00401 STORE_SLICE_1, 00402 STORE_SLICE_2, 00403 STORE_SLICE_3, 00404 UNUSED_2C, 00405 UNUSED_2D, 00406 UNUSED_2E, 00407 UNUSED_2F, 00408 UNUSED_30, /* 0x30 */ 00409 UNUSED_31, 00410 DELETE_SLICE_0, /* d050 */ 00411 DELETE_SLICE_1, 00412 DELETE_SLICE_2, 00413 DELETE_SLICE_3, 00414 STORE_MAP, 00415 INPLACE_ADD, 00416 INPLACE_SUBTRACT, 00417 INPLACE_MULTIPLY, 00418 INPLACE_DIVIDE, 00419 INPLACE_MODULO, 00420 STORE_SUBSCR, /* d060 */ 00421 DELETE_SUBSCR, 00422 BINARY_LSHIFT, 00423 BINARY_RSHIFT, 00424 BINARY_AND, /* 0x40 */ 00425 BINARY_XOR, 00426 BINARY_OR, 00427 INPLACE_POWER, 00428 GET_ITER, 00429 UNUSED_45, 00430 PRINT_EXPR, /* d070 */ 00431 PRINT_ITEM, 00432 PRINT_NEWLINE, 00433 PRINT_ITEM_TO, 00434 PRINT_NEWLINE_TO, 00435 INPLACE_LSHIFT, 00436 INPLACE_RSHIFT, 00437 INPLACE_AND, 00438 INPLACE_XOR, 00439 INPLACE_OR, 00440 BREAK_LOOP, /* 0x50 *//* d080 */ 00441 WITH_CLEANUP, 00442 LOAD_LOCALS, 00443 RETURN_VALUE, 00444 IMPORT_STAR, 00445 EXEC_STMT, 00446 YIELD_VALUE, 00447 POP_BLOCK, 00448 END_FINALLY, 00449 BUILD_CLASS, 00450 00451 /* Opcodes from here have an argument */ 00452 HAVE_ARGUMENT = 90, /* d090 */ 00453 STORE_NAME = 90, 00454 DELETE_NAME, 00455 UNPACK_SEQUENCE, 00456 FOR_ITER, 00457 UNUSED_5E, 00458 STORE_ATTR, 00459 DELETE_ATTR, /* 0x60 */ 00460 STORE_GLOBAL, 00461 DELETE_GLOBAL, 00462 DUP_TOPX, 00463 LOAD_CONST, /* d100 */ 00464 LOAD_NAME, 00465 BUILD_TUPLE, 00466 BUILD_LIST, 00467 BUILD_MAP, 00468 LOAD_ATTR, 00469 COMPARE_OP, 00470 IMPORT_NAME, 00471 IMPORT_FROM, 00472 UNUSED_6D, 00473 JUMP_FORWARD, /* d110 */ 00474 JUMP_IF_FALSE, 00475 JUMP_IF_TRUE, /* 0x70 */ 00476 JUMP_ABSOLUTE, 00477 UNUSED_72, 00478 UNUSED_73, 00479 LOAD_GLOBAL, 00480 UNUSED_75, 00481 UNUSED_76, 00482 CONTINUE_LOOP, 00483 SETUP_LOOP, /* d120 */ 00484 SETUP_EXCEPT, 00485 SETUP_FINALLY, 00486 UNUSED_7B, 00487 LOAD_FAST, 00488 STORE_FAST, 00489 DELETE_FAST, 00490 UNUSED_79, 00491 UNUSED_80, /* 0x80 */ 00492 UNUSED_81, 00493 RAISE_VARARGS, /* d130 */ 00494 CALL_FUNCTION, 00495 MAKE_FUNCTION, 00496 BUILD_SLICE, 00497 MAKE_CLOSURE, 00498 LOAD_CLOSURE, 00499 LOAD_DEREF, 00500 STORE_DEREF, 00501 UNUSED_8A, 00502 UNUSED_8B, 00503 CALL_FUNCTION_VAR, /* d140 */ 00504 CALL_FUNCTION_KW, 00505 CALL_FUNCTION_VAR_KW, 00506 EXTENDED_ARG, 00507 00508 UNUSED_90, UNUSED_91, UNUSED_92, UNUSED_93, 00509 UNUSED_94, UNUSED_95, UNUSED_96, UNUSED_97, 00510 UNUSED_98, UNUSED_99, UNUSED_9A, UNUSED_9B, 00511 UNUSED_9C, UNUSED_9D, UNUSED_9E, UNUSED_9F, 00512 UNUSED_A0, UNUSED_A1, UNUSED_A2, UNUSED_A3, 00513 UNUSED_A4, UNUSED_A5, UNUSED_A6, UNUSED_A7, 00514 UNUSED_A8, UNUSED_A9, UNUSED_AA, UNUSED_AB, 00515 UNUSED_AC, UNUSED_AD, UNUSED_AE, UNUSED_AF, 00516 UNUSED_B0, UNUSED_B1, UNUSED_B2, UNUSED_B3, 00517 UNUSED_B4, UNUSED_B5, UNUSED_B6, UNUSED_B7, 00518 UNUSED_B8, UNUSED_B9, UNUSED_BA, UNUSED_BB, 00519 UNUSED_BC, UNUSED_BD, UNUSED_BE, UNUSED_BF, 00520 UNUSED_C0, UNUSED_C1, UNUSED_C2, UNUSED_C3, 00521 UNUSED_C4, UNUSED_C5, UNUSED_C6, UNUSED_C7, 00522 UNUSED_C8, UNUSED_C9, UNUSED_CA, UNUSED_CB, 00523 UNUSED_CC, UNUSED_CD, UNUSED_CE, UNUSED_CF, 00524 UNUSED_D0, UNUSED_D1, UNUSED_D2, UNUSED_D3, 00525 UNUSED_D4, UNUSED_D5, UNUSED_D6, UNUSED_D7, 00526 UNUSED_D8, UNUSED_D9, UNUSED_DA, UNUSED_DB, 00527 UNUSED_DC, UNUSED_DD, UNUSED_DE, UNUSED_DF, 00528 UNUSED_E0, UNUSED_E1, UNUSED_E2, UNUSED_E3, 00529 UNUSED_E4, UNUSED_E5, UNUSED_E6, UNUSED_E7, 00530 UNUSED_E8, UNUSED_E9, UNUSED_EA, UNUSED_EB, 00531 UNUSED_EC, UNUSED_ED, UNUSED_EE, UNUSED_EF, 00532 UNUSED_F0, UNUSED_F1, UNUSED_F2, UNUSED_F3, 00533 UNUSED_F4, UNUSED_F5, UNUSED_F6, UNUSED_F7, 00534 UNUSED_F8, UNUSED_F9, UNUSED_FA, UNUSED_FB, 00535 UNUSED_FC, UNUSED_FD, UNUSED_FE, UNUSED_FF 00536 #endif /* HAVE_PYTHON27 */ 00537 } PmBcode_t, *pPmBcode_t; 00538 00539 00540 /** 00541 * Interprets the available threads. Does not return. 00542 * 00543 * @param returnOnNoThreads Loop forever if 0, exit with status if no more 00544 * threads left. 00545 * @return Return status if called with returnOnNoThreads != 0, 00546 * will not return otherwise. 00547 */ 00548 PmReturn_t interpret(const uint8_t returnOnNoThreads); 00549 00550 /** 00551 * Selects a thread to run and changes the VM internal variables to 00552 * let the switch-loop execute the chosen one in the next iteration. 00553 * For the moment the algorithm is primitive and will change the 00554 * thread each time it is called in a round-robin fashion. 00555 */ 00556 PmReturn_t interp_reschedule(void); 00557 00558 /** 00559 * Creates a thread object and adds it to the queue of threads to be 00560 * executed while interpret() is running. 00561 * 00562 * The given obj may be a function, module, or class. 00563 * Creates a frame for the given function. 00564 * 00565 * @param pfunc Ptr to function to be executed as a thread. 00566 * @return Return status 00567 */ 00568 PmReturn_t interp_addThread(pPmFunc_t pfunc); 00569 00570 /** 00571 * Sets the reschedule flag. 00572 * 00573 * @param boolean Reschedule on next occasion if boolean is true; clear 00574 * the flag otherwise. 00575 */ 00576 void interp_setRescheduleFlag(uint8_t boolean); 00577 00578 #endif /* __INTERP_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
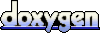