
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
global.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __GLOBAL_H__ 00010 #define __GLOBAL_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief VM Globals 00016 * 00017 * VM globals header. 00018 */ 00019 00020 00021 /** The global root PmGlobals Dict object */ 00022 #define PM_PBUILTINS (pPmObj_t)(gVmGlobal.builtins) 00023 00024 /** The global None object */ 00025 #define PM_NONE (pPmObj_t)(gVmGlobal.pnone) 00026 00027 /** The global False object */ 00028 #define PM_FALSE (pPmObj_t)(gVmGlobal.pfalse) 00029 00030 /** The global True object */ 00031 #define PM_TRUE (pPmObj_t)(gVmGlobal.ptrue) 00032 00033 /** The global integer 0 object */ 00034 #define PM_ZERO (pPmObj_t)(gVmGlobal.pzero) 00035 00036 /** The global integer 1 object */ 00037 #define PM_ONE (pPmObj_t)(gVmGlobal.pone) 00038 00039 /** The global integer -1 object */ 00040 #define PM_NEGONE (pPmObj_t)(gVmGlobal.pnegone) 00041 00042 /** The global string "code" */ 00043 #define PM_CODE_STR (pPmObj_t)(gVmGlobal.pcodeStr) 00044 00045 #ifdef HAVE_CLASSES 00046 /** The global string "__init__" */ 00047 #define PM_INIT_STR (pPmObj_t)(gVmGlobal.pinitStr) 00048 #endif /* HAVE_CLASSES */ 00049 00050 #ifdef HAVE_GENERATORS 00051 /** The global string "Generator" */ 00052 #define PM_GENERATOR_STR (pPmObj_t)(gVmGlobal.pgenStr) 00053 /** The global string "next" */ 00054 #define PM_NEXT_STR (pPmObj_t)(gVmGlobal.pnextStr) 00055 #endif /* HAVE_GENERATORS */ 00056 00057 #ifdef HAVE_ASSERT 00058 /** The global string "Exception" */ 00059 #define PM_EXCEPTION_STR (pPmObj_t)(gVmGlobal.pexnStr) 00060 #endif /* HAVE_ASSERT */ 00061 00062 #ifdef HAVE_BYTEARRAY 00063 /** The global string "bytearray" */ 00064 #define PM_BYTEARRAY_STR (pPmObj_t)(gVmGlobal.pbaStr) 00065 #endif /* HAVE_BYTEARRAY */ 00066 00067 /** The global string "__md" */ 00068 #define PM_MD_STR (pPmObj_t)(gVmGlobal.pmdStr) 00069 00070 00071 /** 00072 * This struct contains ALL of PyMite's globals 00073 */ 00074 typedef struct PmVmGlobal_s 00075 { 00076 /** Global none obj (none) */ 00077 pPmObj_t pnone; 00078 00079 /** Global integer 0 obj */ 00080 pPmInt_t pzero; 00081 00082 /** Global integer 1 obj */ 00083 pPmInt_t pone; 00084 00085 /** Global integer -1 obj */ 00086 pPmInt_t pnegone; 00087 00088 /** Global boolean False obj */ 00089 pPmInt_t pfalse; 00090 00091 /** Global boolean True obj */ 00092 pPmInt_t ptrue; 00093 00094 /** The string "code", used in interp.c RAISE_VARARGS */ 00095 pPmString_t pcodeStr; 00096 00097 /** Dict for builtins */ 00098 pPmDict_t builtins; 00099 00100 /** Paths to available images */ 00101 PmImgPaths_t imgPaths; 00102 00103 /** The single native frame. Static alloc so it won't be GC'd */ 00104 PmNativeFrame_t nativeframe; 00105 00106 /** PyMite release value for when an error occurs */ 00107 uint8_t errVmRelease; 00108 00109 /** PyMite source file ID number for when an error occurs */ 00110 uint8_t errFileId; 00111 00112 /** Line number for when an error occurs */ 00113 uint16_t errLineNum; 00114 00115 /** Thread list */ 00116 pPmList_t threadList; 00117 00118 /** Ptr to current thread */ 00119 pPmThread_t pthread; 00120 00121 #ifdef HAVE_CLASSES 00122 /* NOTE: placing this field before the nativeframe field causes errors */ 00123 /** The string "__init__", used in interp.c CALL_FUNCTION */ 00124 pPmString_t pinitStr; 00125 #endif /* HAVE_CLASSES */ 00126 00127 #ifdef HAVE_GENERATORS 00128 /** The string "Generator", used in interp.c CALL_FUNCTION */ 00129 pPmString_t pgenStr; 00130 /** The string "next", used in interp.c FOR_ITER */ 00131 pPmString_t pnextStr; 00132 #endif /* HAVE_GENERATORS */ 00133 00134 #ifdef HAVE_ASSERT 00135 /** The string "Exception", used in RAISE_VARARGS */ 00136 pPmString_t pexnStr; 00137 #endif /* HAVE_ASSERT */ 00138 00139 #ifdef HAVE_BYTEARRAY 00140 /** The global string "bytearray" */ 00141 pPmString_t pbaStr; 00142 #endif /* HAVE_BYTEARRAY */ 00143 00144 /** The global string "__md" */ 00145 pPmString_t pmdStr; 00146 00147 #ifdef HAVE_PRINT 00148 /** Remembers when a space is needed before printing the next object */ 00149 uint8_t needSoftSpace; 00150 /** Remembers when something has printed since the last newline */ 00151 uint8_t somethingPrinted; 00152 #endif /* HAVE_PRINT */ 00153 00154 /** Flag to trigger rescheduling */ 00155 uint8_t reschedule; 00156 } PmVmGlobal_t, 00157 *pPmVmGlobal_t; 00158 00159 00160 extern volatile PmVmGlobal_t gVmGlobal; 00161 00162 00163 /** 00164 * Initializes the global struct 00165 * 00166 * @return Return status 00167 */ 00168 PmReturn_t global_init(void); 00169 00170 /** 00171 * Sets the builtins dict into the given module's attrs. 00172 * 00173 * If not yet done, loads the "__bt" module via global_loadBuiltins(). 00174 * Restrictions described in that functions documentation apply. 00175 * 00176 * @param pmod Module whose attrs receive builtins 00177 * @return Return status 00178 */ 00179 PmReturn_t global_setBuiltins(pPmFunc_t pmod); 00180 00181 /** 00182 * Loads the "__bt" module and sets the builtins dict (PM_PBUILTINS) 00183 * to point to __bt's attributes dict. 00184 * Creates "None" = None entry in builtins. 00185 * 00186 * When run, there should not be any other threads in the interpreter 00187 * thread list yet. 00188 * 00189 * @return Return status 00190 */ 00191 PmReturn_t global_loadBuiltins(void); 00192 00193 #endif /* __GLOBAL_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
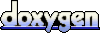