
This is a sample program, "SevenSegmentLed" library this is. I re-create the sample program anew.
Dependencies: SevenSegLed mbed
main.cpp
00001 /************************************************* 00002 * SevenSegLed Library sample program 00003 * 00004 * <schematic> 00005 * 1.seven segment numeric LED Display : OSL40562-LR 00006 * http://akizukidenshi.com/download/OSL40562-LR.pdf 00007 * 00008 * OSL40562-LR Resister LPC1768 00009 * Pin No Function [ohm] PinNo 00010 * --------------------------------------------------------------------------- 00011 * 1 segment E 100 p10 00012 * 2 segment D 100 p11 00013 * 3 segment Dp 100 p12 00014 * 4 segment C 100 p13 00015 * 5 segment G 100 p14 00016 * 6 common 4 - p15 00017 * 7 segment B 100 p26 00018 * 8 common 3 - p27 00019 * 9 common 2 - p28 00020 * 10 segment F 100 p29 00021 * 11 segment A 100 p30 00022 * 12 common 1 - p21 00023 * 00024 **************************************************/ 00025 00026 #include "mbed.h" 00027 #include "SevenSegLed.h" 00028 00029 // common type (0:anode common 1:cathode common) 00030 // | 00031 // | display mode (0:smooth 1:hard) 00032 // | | 00033 // | | segA segB segC segD segE segF segG segP com1 com2 com3 com4 (com5,com6,com7,com8 = NC) 00034 // | | | | | | | | | | | | | | 00035 SevenSegLed sevenSegLed(1, 0, p30, p26, p13, p11, p10, p29, p14, p12, p21, p28, p27, p15); // OSL40562-LR 00036 00037 //================================ 00038 // display buffer 00039 //================================ 00040 // com1 00041 // | com2 00042 // | | com3 00043 // | | | com4 00044 // | | | | 00045 uint8_t D_7seg[4] = {0, 0, 0, 0}; // 0x0 to 0x9 = "0" to "9" ,0xA to 0xF = "A" to "F", 0x10 = extinction 00046 uint8_t D_dot[4] = {0, 1, 0, 0}; // 0:extinction 1: light 00047 00048 00049 //============================== 00050 // Variable counter & work 00051 //============================== 00052 uint8_t count = 0; // free run counter (0x00 to 0xFF overflow to 0x00) 00053 uint8_t work; 00054 00055 //============================== 00056 // main 00057 //============================== 00058 int main() { 00059 00060 while(1) { 00061 00062 //========================== 00063 // Show hexadecimal 00064 //========================== 00065 work = count / 0x10; 00066 D_7seg[0] = work; 00067 00068 work = count % 0x10; 00069 D_7seg[1] = work; 00070 00071 //======================== 00072 // Show decimal 00073 //======================== 00074 work = count / 10; 00075 work %= 10; 00076 D_7seg[2] = work; 00077 00078 work = count % 10; 00079 D_7seg[3] = work; 00080 00081 //======================= 00082 // Show dot 00083 //======================= 00084 if((count % 2) == 0){ 00085 D_dot[1] = 1; 00086 D_dot[3] = 0; 00087 } 00088 else{ 00089 D_dot[1] = 0; 00090 D_dot[3] = 1; 00091 } 00092 00093 D_dot[0] = 0; 00094 //D_dot[1] = 0; 00095 D_dot[2] = 0; 00096 //D_dot[3] = 0; 00097 00098 //============================== 00099 // set dynamic output data 00100 //============================== 00101 sevenSegLed.SevenSegLed_main(D_7seg, D_dot); 00102 00103 //============================== 00104 // counter increment & time wait 00105 //============================== 00106 count++; 00107 00108 wait(1.0); 00109 00110 } 00111 }
Generated on Sun Jul 17 2022 15:14:44 by
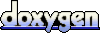