Embed:
(wiki syntax)
Show/hide line numbers
HMC6343.h
00001 /** 00002 * @author Serge Sozonoff 00003 * @author Aaron Berk 00004 * Partially based on the work of Aaron Berk for the HMC6352 00005 * 00006 * @section LICENSE 00007 * 00008 * Copyright (c) 2010 ARM Limited 00009 * 00010 * Permission is hereby granted, free of charge, to any person obtaining a copy 00011 * of this software and associated documentation files (the "Software"), to deal 00012 * in the Software without restriction, including without limitation the rights 00013 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00014 * copies of the Software, and to permit persons to whom the Software is 00015 * furnished to do so, subject to the following conditions: 00016 * 00017 * The above copyright notice and this permission notice shall be included in 00018 * all copies or substantial portions of the Software. 00019 * 00020 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00021 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00022 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00023 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00024 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00025 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00026 * THE SOFTWARE. 00027 * 00028 * @section DESCRIPTION 00029 * 00030 * Honeywell HMC6343 digital compass. 00031 * 00032 * Datasheet: 00033 * 00034 * http://www.ssec.honeywell.com/magnetic/datasheets/HMC6343.pdf 00035 */ 00036 00037 #ifndef HMC6343_H 00038 #define HMC6343_H 00039 00040 /** 00041 * Includes 00042 */ 00043 #include "mbed.h" 00044 00045 /** 00046 * Defines 00047 */ 00048 #define HMC6343_I2C_ADDRESS 0x32 >> 1 //7-bit address 00049 00050 //Commands. 00051 #define HMC6343_EEPROM_WRITE 0xF1 00052 #define HMC6343_EEPROM_READ 0xE1 00053 #define HMC6343_ENTER_SLEEP 0x83 00054 #define HMC6343_EXIT_SLEEP 0x84 00055 #define HMC6343_ENTER_STANDBY 0x76 00056 #define HMC6343_ENTER_RUN 0x75 00057 #define HMC6343_RESET 0x82 00058 #define HMC6343_ENTER_CALIB 0x71 00059 #define HMC6343_EXIT_CALIB 0x7E 00060 #define HMC6343_GET_ACCEL_DATA 0x40 00061 #define HMC6343_GET_MAG_DATA 0x45 00062 #define HMC6343_GET_HEADING_DATA 0x50 00063 #define HMC6343_GET_TILT_DATA 0x55 00064 #define HMC6343_GET_HEADING_DATA 0x50 00065 #define HMC6343_SET_XFWD_PLS_ZUP 0x72 00066 #define HMC6343_SET_XFWD_YUP 0x73 00067 #define HMC6343_SET_ZFWD_NEG_XUP 0x74 00068 #define HMC6343_GET_OPMODE 0x65 00069 00070 //EEPROM locations. 00071 #define HMC6343_SLAVE_ADDR 0x00 00072 #define HMC6343_OPMOD_REG1 0x04 00073 #define HMC6343_OPMOD_REG2 0x05 00074 #define HMC6343_SN_LSB 0x06 00075 #define HMC6343_SN_MSB 0x07 00076 #define HMC6343_DEV_LSB 0x0A 00077 #define HMC6343_DEV_MSB 0x0B 00078 #define HMC6343_VAR_LSB 0x0C 00079 #define HMC6343_VAR_MSB 0x0D 00080 #define HMC6343_XOFFSET_LSB 0x0E 00081 #define HMC6343_XOFFSET_MSB 0x0F 00082 #define HMC6343_YOFFSET_LSB 0x10 00083 #define HMC6343_YOFFSET_MSB 0x11 00084 #define HMC6343_ZOFFSET_LSB 0x12 00085 #define HMC6343_ZOFFSET_MSB 0x13 00086 #define HMC6343_IIRF_LSB 0x14 00087 #define HMC6343_IIRF_MSB 0x15 00088 #define HMC6343_SOFT_VER 0x02 00089 00090 00091 #define HMC6343_X_AXIS 0x01 00092 #define HMC6343_Y_AXIS 0x02 00093 #define HMC6343_Z_AXIS 0x04 00094 00095 00096 // Operation mode bit masks 00097 // LSB 00098 #define HMC6343_COMP 0x80 00099 #define HMC6343_CAL 0x40 00100 #define HMC6343_FILTER 0x20 00101 #define HMC6343_RUN 0x10 00102 #define HMC6343_STDBY 0x08 00103 #define HMC6343_UF 0x04 00104 #define HMC6343_UE 0x02 00105 #define HMC6343_LEVEL 0x01 00106 00107 00108 //Operational mode register masks. 00109 #define HMC6343_CM_MR_1HZ 0x00 00110 #define HMC6343_CM_MR_5HZ 0x100 00111 #define HMC6343_CM_MR_10HZ 0x200 00112 00113 00114 struct Heading { 00115 float heading; 00116 float pitch; 00117 float roll; 00118 }; 00119 00120 /** 00121 * Honeywell HMC6343 digital compass. 00122 */ 00123 class HMC6343 { 00124 00125 public: 00126 00127 /** 00128 * Constructor. 00129 * 00130 * @param sda mbed pin to use for SDA line of I2C interface. 00131 * @param scl mbed pin to use for SCL line of I2C interface. 00132 */ 00133 HMC6343(PinName sda, PinName scl); 00134 00135 HMC6343(I2C& p_itc); 00136 00137 /** 00138 * Sample the device and return the result. 00139 * 00140 * @return In heading output mode, the current heading as a number between 00141 * 0-3599, representing 0-359.9 degrees. 00142 * In raw magnetometer X output mode, the raw output of the X-axis 00143 * magnetometer. 00144 * In raw magnetometer Y mode, the raw output of the Y-axis 00145 * magnetometer. 00146 * In magnetometer X mode, the corrected output of the X-axis 00147 * magnetometer. 00148 * In magnetometer Y mode, the corrected output of the Y-axis 00149 * magnetometer. 00150 */ 00151 void sampleHeading(Heading* p_heading); 00152 00153 /** 00154 * Update bridge offsets. 00155 * 00156 * Performs a set/reset immediately. 00157 */ 00158 void setReset(void); 00159 00160 /** 00161 * Enter into or exit from calibration mode. 00162 * 00163 * @param enterOrExit 0x45 -> Exit 00164 * 0x43 -> Enter 00165 */ 00166 void setCalibrationMode(int enterOrExit); 00167 00168 /** 00169 * Save the current operation mode byte to EEPROM. 00170 */ 00171 void saveOpMode(void); 00172 00173 /** 00174 * Read the memory location on the device which contains the slave address. 00175 * 00176 * @return The slave address of the device. 00177 */ 00178 int getSlaveAddress(void); 00179 00180 /** 00181 * Read the current offset for X or Y axis magnetometer. 00182 * 00183 * @param axis 0x01 -> X-axis 00184 * 0x02 -> Y-axis 00185 * 0x04 -> Z-axis 00186 * @return The current offset for the axis as a 16-bit number. 00187 */ 00188 int getOffset(int axis); 00189 00190 00191 /** 00192 * Get the software version on the device. 00193 * 00194 * @return The software version number. 00195 */ 00196 int getSoftwareVersion(void); 00197 00198 /** 00199 * Get the current operation mode. 00200 * 00201 * @return 0x00 -> Standby mode 00202 * 0x01 -> Query mode 00203 * 0x02 -> Continuous mode 00204 */ 00205 int getOpMode(void); 00206 00207 /** 00208 * Set the operation mode. 00209 * 00210 * @param periodicSetReset 0x00 -> No periodic set/reset 00211 * 0x01 -> Periodic set/reset 00212 */ 00213 void setOpMode(int opMode); 00214 00215 00216 void setMagneticVariation(float var); 00217 float getMagneticVariation(); 00218 void setMagneticDeviation(float data); 00219 float getMagneticDeviation(); 00220 bool isOpModeFlagSet(int flag); 00221 void setIIRFilter(short data); 00222 short getIIRFilter(); 00223 void setMagOffset(int axis, int offset); 00224 int getMagOffset(int axis); 00225 char getMeasurementRate(); 00226 00227 private: 00228 00229 I2C* i2c_; 00230 int operationMode_; 00231 00232 /** 00233 * Write to EEPROM on the device. 00234 * 00235 * @param address Address to write to. 00236 * @param data Data to write. 00237 */ 00238 void writeEeprom(int address, int data); 00239 00240 /** 00241 * Read EEPROM on the device. 00242 * 00243 * @param address Address to read from. 00244 * @return The contents of the memory address. 00245 */ 00246 int readEeprom(int address); 00247 00248 void writeShort(int lsb_address, short data); 00249 short readShort(int lsb_eprom_address); 00250 }; 00251 00252 #endif /* HMC6343_H */
Generated on Wed Jul 13 2022 02:55:49 by
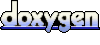