
seeing i robot - with all the file systems and complete code
Dependencies: mbed SRF05 Servo CMPS03
VS1002.cpp
00001 #include "VS1002.h" 00002 #include "mbed.h" 00003 00004 00005 00006 /* ================================================================== 00007 * Constructor 00008 * =================================================================*/ 00009 VS1002::VS1002( 00010 PinName mmosi, PinName mmiso, PinName ssck, PinName ccs, const char *name, 00011 PinName mosi, PinName miso, PinName sck, PinName cs, PinName rst, 00012 PinName dreq, PinName dcs, PinName vol) 00013 : 00014 _sd(mmosi, mmiso, ssck, ccs, name), 00015 _spi(mosi, miso, sck), 00016 _CS(cs), 00017 _RST(rst), 00018 _DREQ(dreq), 00019 _DCS(dcs), 00020 _VOL(vol) { 00021 00022 } 00023 00024 /*=================================================================== 00025 * Functions 00026 *==================================================================*/ 00027 00028 void VS1002::cs_low(void) 00029 { 00030 _CS = 0; 00031 } 00032 void VS1002::cs_high(void) 00033 { 00034 _CS = 1; 00035 } 00036 void VS1002::dcs_low(void) 00037 { 00038 _DCS = 0; 00039 } 00040 void VS1002::dcs_high(void) 00041 { 00042 _DCS = 1; 00043 } 00044 void VS1002::sci_en(void) //SCI enable 00045 { 00046 cs_high(); 00047 dcs_high(); 00048 cs_low(); 00049 } 00050 void VS1002::sci_dis(void) //SCI disable 00051 { 00052 cs_high(); 00053 } 00054 void VS1002::sdi_en(void) //SDI enable 00055 { 00056 dcs_high(); 00057 cs_high(); 00058 dcs_low(); 00059 } 00060 void VS1002::sdi_dis(void) //SDI disable 00061 { 00062 dcs_high(); 00063 } 00064 void VS1002::reset(void) //hardware reset 00065 { 00066 wait(0.01); 00067 _RST = 0; 00068 wait(0.01); 00069 _RST = 1; 00070 wait(0.10); 00071 } 00072 void VS1002::power_down(void) //hardware and software reset 00073 { 00074 cs_low(); 00075 reset(); 00076 sci_write(0x00, SM_PDOWN); 00077 wait(0.01); 00078 reset(); 00079 } 00080 void VS1002::sci_initialise(void) 00081 { 00082 _RST = 1; //no reset 00083 _spi.format(8,0); //spi 8bit interface, steady state low 00084 _spi.frequency(1000000); //rising edge data record, freq. 1Mhz 00085 00086 cs_low(); 00087 for(int i=0; i<4; i++) 00088 { 00089 _spi.write(0xFF); //clock the chip a bit 00090 } 00091 cs_high(); 00092 dcs_high(); 00093 wait_us(5); 00094 // fprintf(stderr, "Timeout waiting for v1.x card\n"); 00095 00096 } 00097 void VS1002::sdi_initialise(void) 00098 { 00099 _spi.format(8,0); 00100 _spi.frequency(7000000); //set to 7MHz 00101 00102 cs_high(); 00103 dcs_high(); 00104 } 00105 void VS1002::sci_write(unsigned char address, unsigned short int data) 00106 { 00107 sci_en(); //enables SCI/disables SDI 00108 00109 while(!_DREQ); //wait unitl data request is high 00110 _spi.write(0x02); //SCI write 00111 _spi.write(address); //register address 00112 _spi.write((data >> 8) & 0xFF); //write out first half of data word 00113 _spi.write(data & 0xFF); //write out second half of data word 00114 00115 sci_dis(); //enables SDI/disables SCI 00116 wait_us(5); 00117 } 00118 void VS1002::sdi_write(unsigned char datum) 00119 { 00120 sdi_en(); 00121 00122 while(!_DREQ); 00123 _spi.write(datum); 00124 00125 sci_dis(); 00126 } 00127 unsigned short int VS1002::read(unsigned short int address) 00128 { 00129 cs_low(); //enables SCI/disables SDI 00130 00131 while(!_DREQ); //wait unitl data request is high 00132 _spi.write(0x03); //SCI write 00133 _spi.write(address); //register address 00134 unsigned short int received = _spi.write(0x00); //write out dummy byte 00135 received <<= 8; 00136 received += _spi.write(0x00); //write out dummy byte 00137 00138 cs_high(); //enables SDI/disables SCI 00139 00140 return received; //return received word 00141 } 00142 void VS1002::sine_test_activate(unsigned char wave) 00143 { 00144 cs_high(); //enables SDI/disables SCI 00145 00146 while(!_DREQ); //wait unitl data request is high 00147 _spi.write(0x53); //SDI write 00148 _spi.write(0xEF); //SDI write 00149 _spi.write(0x6E); //SDI write 00150 _spi.write(wave); //SDI write 00151 _spi.write(0x00); //filler byte 00152 _spi.write(0x00); //filler byte 00153 _spi.write(0x00); //filler byte 00154 _spi.write(0x00); //filler byte 00155 00156 cs_low(); //enables SCI/disables SDI 00157 } 00158 void VS1002::sine_test_deactivate(void) 00159 { 00160 cs_high(); 00161 00162 while(!_DREQ); 00163 _spi.write(0x45); //SDI write 00164 _spi.write(0x78); //SDI write 00165 _spi.write(0x69); //SDI write 00166 _spi.write(0x74); //SDI write 00167 _spi.write(0x00); //filler byte 00168 _spi.write(0x00); //filler byte 00169 _spi.write(0x00); //filler byte 00170 _spi.write(0x00); //filler byte 00171 } 00172 void VS1002::volume(void) 00173 { 00174 #ifdef FIXED_VOL 00175 unsigned char volumize = (0 * 255); // FIXED VOL (not support volume input) 00176 #else 00177 unsigned char volumize = (0 * 255); 00178 #endif 00179 while(!_DREQ); 00180 00181 unsigned short int attenuation = ((256 * volumize) + volumize); 00182 sci_write(0x0B, attenuation); 00183 } 00184 00185 void VS1002::play_song(int song_number) 00186 { 00187 /*====== Song Select ======*/ 00188 00189 // char list[10000] = {0}; 00190 char list[1000] = {0}; 00191 char str[16] = {"/sd/"}; 00192 unsigned int startplace = 0; 00193 unsigned int endplace = 0; 00194 unsigned int play = 0; 00195 num_of_files = 0; 00196 00197 DIR *d; 00198 struct dirent *p; 00199 d = opendir("/sd"); 00200 if(d != NULL) 00201 { 00202 while((p = readdir(d)) != NULL) 00203 { 00204 strcat(list, "*"); 00205 strcat(list, p->d_name); 00206 fprintf(stderr,"\n\rFilename:%s", p->d_name); 00207 num_of_files++; 00208 } 00209 } 00210 else 00211 { 00212 perror("Could not open directory!"); 00213 } 00214 strcat(list, "*"); //terminating * 00215 if(num_of_files < song_number) 00216 { 00217 return; 00218 } 00219 while(play != song_number) 00220 { 00221 char symbol = list[startplace]; 00222 startplace++; 00223 if(symbol == 0x2A) //0x2A = "*" 00224 { 00225 play++; 00226 } 00227 } 00228 play = 0; 00229 while(play != (song_number+1)) 00230 { 00231 char symbol = list[endplace]; 00232 endplace++; 00233 if(symbol == 0x2A) //0x2A = "*" 00234 { 00235 play++; 00236 } 00237 } 00238 00239 strncat(str, &list[startplace], endplace-startplace); 00240 str[(endplace-startplace)+3] = '\0'; 00241 00242 //printf("list: %s\r\n",list); //debug 00243 00244 /*====== File Transfer ======*/ 00245 00246 // return if not MP3 file 00247 if (!((strstr(str,"MP3")!=NULL)||(strstr(str,"mp3")!=NULL))) return; 00248 // display filename.mp3 00249 fprintf(stderr, "\n\rNow Playing: %s\r\n",str); 00250 00251 FILE *song; 00252 unsigned char array[512]; 00253 00254 song = fopen(str, "rb"); 00255 00256 if(!song) 00257 { 00258 exit(1); 00259 } 00260 00261 while(!feof(song)) 00262 { 00263 fread(&array, 1, 512, song); 00264 for(int i=0; i<512; i++) 00265 { 00266 #ifndef FS_ONLY 00267 sdi_write(array[i]); 00268 // printf("."); 00269 #endif 00270 } 00271 #ifndef FS_ONLY 00272 volume(); 00273 #endif 00274 } 00275 for(int n=0; n<2048; n++) 00276 { 00277 #ifndef FS_ONLY 00278 sdi_write(0x00); 00279 #endif 00280 } 00281 fclose(song); //close the file 00282 }
Generated on Sun Jul 24 2022 09:54:37 by
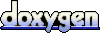