SuperTweet interface driver classes.
Dependents: SuperTweet_TestProgram StarBoardOrangeExpansion1 GSL_04-Network_Twitter
SuperTweet.h
00001 /** 00002 * SuperTweet API interface driver. (Version 0.0.1) 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 */ 00007 00008 #include <string> 00009 #include "HTTPClient.h" 00010 00011 /** 00012 * SuperTweet driver class. 00013 */ 00014 class SuperTweet { 00015 public: 00016 00017 /** 00018 * Create. 00019 * 00020 * @param account Account name. 00021 * @param password Password. 00022 */ 00023 SuperTweet(const std::string account, const std::string password); 00024 00025 /** 00026 * Dispose. 00027 */ 00028 virtual ~SuperTweet(); 00029 00030 /** 00031 * Set timeout. 00032 * 00033 * @param ms Timeout. [ms] 00034 */ 00035 void setTimeout(int ms); 00036 00037 /** 00038 * Returns the 20 most recent statuses posted by the authenticating user. 00039 * 00040 * @param func A pointer to a callback function. 00041 * @return Result code. 00042 */ 00043 virtual HTTPResult getStatusesUserTimeline(void (*func)(char *buf, size_t siz) = NULL) = 0; 00044 00045 /** 00046 * Returns the 20 most recent statuses, including retweets if they exist, posted by the authenticating user and the user's they follow. 00047 * 00048 * @param func A pointer to a callback function. 00049 * @return Result code. 00050 */ 00051 virtual HTTPResult getStatusesHomeTimeline(void (*func)(char *buf, size_t siz) = NULL) = 0; 00052 00053 /** 00054 * Updates the authenticating user's status. 00055 * A status update with text identical to the authenticating user's text identical 00056 * to the authenticating user's current status will be ignored to prevent duplicates. 00057 * 00058 * @param func A pointer to a callback function. 00059 * @return Result code. 00060 */ 00061 virtual HTTPResult postStatusesUpdate(const std::string datatext, void (*func)(char *buf, size_t siz) = NULL) = 0; 00062 00063 protected: 00064 static const std::string URLBASE_V1; 00065 const std::string account; 00066 const std::string password; 00067 HTTPClient client; 00068 };
Generated on Tue Jul 12 2022 20:54:58 by
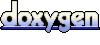