SuperTweet interface driver classes.
Dependents: SuperTweet_TestProgram StarBoardOrangeExpansion1 GSL_04-Network_Twitter
SuperTweetV1XML.cpp
00001 /** 00002 * SuperTweet API interface driver. (Version 0.0.1) 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 */ 00007 00008 #include "SuperTweetV1XML.h" 00009 00010 /** 00011 * Create. 00012 * 00013 * @param account Account name. 00014 * @param password Password. 00015 */ 00016 SuperTweetV1XML::SuperTweetV1XML(const std::string account, const std::string password) 00017 : SuperTweet(account, password) { 00018 } 00019 00020 /** 00021 * Dispose. 00022 */ 00023 SuperTweetV1XML::~SuperTweetV1XML() { 00024 } 00025 00026 /** 00027 * Returns the 20 most recent statuses posted by the authenticating user. 00028 * 00029 * @param func A pointer to a callback function. 00030 * @return Result code. 00031 */ 00032 HTTPResult SuperTweetV1XML::getStatusesUserTimeline(void (*func)(char *buf, size_t siz)) { 00033 HTTPMap map; 00034 HTTPStream stream; 00035 const std::string url = URLBASE_V1 + "statuses/user_timeline.xml"; 00036 char buf[BUFSIZ + 1] = {0}; 00037 00038 result = HTTP_OK; 00039 completed = false; 00040 stream.readNext((byte*)buf, sizeof(buf) - 1); 00041 HTTPResult r = client.get(url.c_str(), &stream, this, &SuperTweetV1XML::callback); 00042 while (!completed) { 00043 Net::poll(); 00044 if (stream.readable()) { 00045 buf[stream.readLen()] = 0; 00046 if (func != NULL) { 00047 func(buf, stream.readLen()); 00048 } 00049 stream.readNext((byte*)buf, sizeof(buf) - 1); 00050 } 00051 } 00052 return result; 00053 } 00054 00055 /** 00056 * Returns the 20 most recent statuses, including retweets if they exist, posted by the authenticating user and the user's they follow. 00057 * 00058 * @param func A pointer to a callback function. 00059 * @return Result code. 00060 */ 00061 HTTPResult SuperTweetV1XML::getStatusesHomeTimeline(void (*func)(char *buf, size_t siz)) { 00062 HTTPMap map; 00063 HTTPStream stream; 00064 const std::string url = URLBASE_V1 + "statuses/home_timeline.xml"; 00065 char buf[BUFSIZ + 1] = {0}; 00066 00067 result = HTTP_OK; 00068 completed = false; 00069 stream.readNext((byte*)buf, sizeof(buf) - 1); 00070 HTTPResult r = client.get(url.c_str(), &stream, this, &SuperTweetV1XML::callback); 00071 if (r != HTTP_OK) { 00072 while (!completed) { 00073 Net::poll(); 00074 if (stream.readable()) { 00075 buf[stream.readLen()] = 0; 00076 if (func != NULL) { 00077 func(buf, stream.readLen()); 00078 } 00079 stream.readNext((byte*)buf, sizeof(buf) - 1); 00080 } 00081 } 00082 } 00083 return result; 00084 } 00085 00086 /** 00087 * Updates the authenticating user's status. 00088 * A status update with text identical to the authenticating user's text identical 00089 * to the authenticating user's current status will be ignored to prevent duplicates. 00090 * 00091 * @param func A pointer to a callback function. 00092 * @return Result code. 00093 */ 00094 HTTPResult SuperTweetV1XML::postStatusesUpdate(const std::string datatext, void (*func)(char *buf, size_t siz)) { 00095 HTTPMap map; 00096 HTTPStream stream; 00097 map["status"] = datatext; 00098 const std::string url = URLBASE_V1 + "statuses/update.xml"; 00099 char buf[BUFSIZ + 1] = {0}; 00100 00101 result = HTTP_OK; 00102 completed = false; 00103 stream.readNext((byte*)buf, sizeof(buf) - 1); 00104 HTTPResult r = client.post(url.c_str(), map, &stream, this, &SuperTweetV1XML::callback); 00105 while (!completed) { 00106 Net::poll(); 00107 if (stream.readable()) { 00108 buf[stream.readLen()] = 0; 00109 if (func != NULL) { 00110 func(buf, stream.readLen()); 00111 } 00112 stream.readNext((byte*)buf, sizeof(buf) - 1); 00113 } 00114 } 00115 return result; 00116 } 00117 00118 void SuperTweetV1XML::callback(HTTPResult r) { 00119 result = r; 00120 completed = true; 00121 }
Generated on Tue Jul 12 2022 20:54:58 by
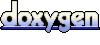