The FirmwareUpdater is a mbed firmware update library with HTTP server on cloud.
Dependents: FirmwareUpdater_TestProgram geigercounter04 firm LPC1768_up_frim
FirmwareUpdater.h
00001 /** 00002 * ============================================================================= 00003 * Firmware updater (Version 0.0.2) 00004 * ============================================================================= 00005 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, including without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 * ============================================================================= 00025 */ 00026 00027 #ifndef FIRMWARE_UPDATER_H 00028 #define FIRMWARE_UPDATER_H 00029 00030 #include "mbed.h" 00031 #include "HTTPClient.h" 00032 #include "LocalFileSystem.h" 00033 #include <string> 00034 00035 /** 00036 * @code 00037 * #include "mbed.h" 00038 * #include "FirmwareUpdater.h" 00039 * #include "EthernetNetIf.h" 00040 * 00041 * EthernetNetIf eth; 00042 * FirmwareUpdater fwup("http://mbed.org/media/uploads/shintamainjp/", "firm", true); 00043 * 00044 * // There are 2 files for the firmware. 00045 * // 1. firm.txt : firmware version file. 00046 * // 2. firm.bin : firmware binary file. 00047 * 00048 * int main() { 00049 * eth.setup(); 00050 * if (fwup.exist() == 0) { 00051 * printf("Found a new firmware.\n"); 00052 * if (fwup.execute() == 0) { 00053 * printf("Update succeed.\n"); 00054 * printf("Resetting this system...\n\n\n\n\n"); 00055 * fwup.reset(); 00056 * } else { 00057 * printf("Update failed!\n"); 00058 * } 00059 * } 00060 * } 00061 * @endcode 00062 */ 00063 class FirmwareUpdater { 00064 public: 00065 /** 00066 * Create. 00067 * 00068 * @param url URL for firmware. Do not include a target file name. 00069 * @param name An application name. Do not include a extention. 00070 * @param log True if logging. 00071 */ 00072 FirmwareUpdater(std::string url, std::string name, bool log = false); 00073 00074 /** 00075 * Dispose. 00076 */ 00077 ~FirmwareUpdater(); 00078 00079 /** 00080 * Get a URL. 00081 * 00082 * @return URL. 00083 */ 00084 const std::string getURL() const; 00085 00086 /** 00087 * Get a name. 00088 * 00089 * @return name. 00090 */ 00091 const std::string getName() const; 00092 00093 /** 00094 * Checking a new firmware. 00095 * Compare versions of the software between local storage on mbed and on webserver. 00096 * 00097 * @return Return 0 if a new firmware exists. 00098 */ 00099 int exist(); 00100 00101 /** 00102 * Execute update. 00103 * 00104 * @return Return 0 if it succeed. 00105 */ 00106 int execute(); 00107 00108 /** 00109 * Reset system. 00110 */ 00111 void reset(); 00112 00113 private: 00114 static const int MAXNAMELEN = 8; 00115 static const std::string EXT_BIN; 00116 static const std::string EXT_BINTMP; 00117 static const std::string EXT_TXT; 00118 static const std::string EXT_TXTTMP; 00119 const std::string url; 00120 const std::string name; 00121 const bool log; 00122 LocalFileSystem local; 00123 HTTPClient client; 00124 00125 /** 00126 * Fetch a file. 00127 * 00128 * @param src_url URL of a source file. 00129 * @param local_file Local file name. 00130 * 00131 * @return Return 0 if it succeed. 00132 */ 00133 int fetch(std::string src_url, std::string local_file); 00134 00135 /** 00136 * Copy a file. 00137 * 00138 * @param local_file1 Source file. 00139 * @param local_file2 Destination file. 00140 * 00141 * @return Return 0 if it succeed. 00142 */ 00143 int copy(std::string local_file1, std::string local_file2); 00144 00145 /** 00146 * Output a message to a log file. 00147 * 00148 * @param format ... 00149 */ 00150 void LOG(const char* format, ...); 00151 00152 /** 00153 * Cleanup all bin files. 00154 */ 00155 int cleanupAllBinFiles(void); 00156 00157 /** 00158 * Create a new binary file name. 00159 * 00160 * @param ver Version. 00161 * @param buf A pointer to a buffer. 00162 * @param siz A size of the buffer. 00163 * 00164 * @return Return 0 if it succeed. 00165 */ 00166 int createNewBinName(const int ver, char *buf, size_t siz); 00167 00168 /** 00169 * Read a version from a file. 00170 * 00171 * @param filename file name. 00172 * @return A version. 00173 */ 00174 int readVersionFromFile(const char *filename); 00175 00176 /** 00177 * Read a version from a URL. 00178 * 00179 * @param url URL. 00180 * @return A version. 00181 */ 00182 int readVersionFromURL(const char *url); 00183 }; 00184 00185 #endif
Generated on Wed Jul 13 2022 06:52:56 by
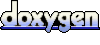