Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
BusInOut.h
00001 /* mbed Microcontroller Library - BusInOut 00002 * Copyright (c) 2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_BUSINOUT_H 00006 #define MBED_BUSINOUT_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 #include "DigitalInOut.h" 00013 00014 namespace mbed { 00015 00016 /** A digital input output bus, used for setting the state of a collection of pins 00017 */ 00018 class BusInOut : public Base { 00019 00020 public: 00021 00022 /** Create an BusInOut, connected to the specified pins 00023 * 00024 * @param p<n> DigitalInOut pin to connect to bus bit p<n> (p5-p30, NC) 00025 * 00026 * @note 00027 * It is only required to specify as many pin variables as is required 00028 * for the bus; the rest will default to NC (not connected) 00029 */ 00030 BusInOut(PinName p0, PinName p1 = NC, PinName p2 = NC, PinName p3 = NC, 00031 PinName p4 = NC, PinName p5 = NC, PinName p6 = NC, PinName p7 = NC, 00032 PinName p8 = NC, PinName p9 = NC, PinName p10 = NC, PinName p11 = NC, 00033 PinName p12 = NC, PinName p13 = NC, PinName p14 = NC, PinName p15 = NC, 00034 const char *name = NULL); 00035 00036 BusInOut(PinName pins[16], const char *name = NULL); 00037 00038 virtual ~BusInOut(); 00039 00040 /* Group: Access Methods */ 00041 00042 /** Write the value to the output bus 00043 * 00044 * @param value An integer specifying a bit to write for every corresponding DigitalInOut pin 00045 */ 00046 void write(int value); 00047 00048 00049 /** Read the value currently output on the bus 00050 * 00051 * @returns 00052 * An integer with each bit corresponding to associated DigitalInOut pin setting 00053 */ 00054 int read(); 00055 00056 /** Set as an output 00057 */ 00058 void output(); 00059 00060 /** Set as an input 00061 */ 00062 void input(); 00063 00064 /** Set the input pin mode 00065 * 00066 * @param mode PullUp, PullDown, PullNone 00067 */ 00068 void mode(PinMode pull); 00069 00070 #ifdef MBED_OPERATORS 00071 /** A shorthand for write() 00072 */ 00073 BusInOut& operator= (int v); 00074 BusInOut& operator= (BusInOut& rhs); 00075 00076 /** A shorthand for read() 00077 */ 00078 operator int(); 00079 #endif 00080 00081 #ifdef MBED_RPC 00082 virtual const struct rpc_method *get_rpc_methods(); 00083 static struct rpc_class *get_rpc_class(); 00084 #endif 00085 00086 protected: 00087 00088 DigitalInOut* _pin[16]; 00089 00090 #ifdef MBED_RPC 00091 static void construct(const char *arguments, char *res); 00092 #endif 00093 00094 }; 00095 00096 } // namespace mbed 00097 00098 #endif 00099
Generated on Tue Jul 12 2022 11:27:25 by
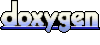