
9 tile screen working with one image space, platform for development
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ledScreen.h" 00003 00004 // screen instance 00005 ledScreen screen; 00006 00007 // sin lookup table and related functions 00008 unsigned char sinlut[256]; 00009 00010 void initSinLut() { 00011 for (int i=0; i<256; i++) 00012 sinlut[i] = cos((float)i / 256.0 * (3.14159265 * 2))*127 + 128; 00013 } 00014 00015 inline unsigned char lut_sin(int x) { 00016 00017 return (x>0)?sinlut[x%256]:sinlut[(-x)%256]; 00018 } 00019 00020 00021 // Example frame makes: 00022 00023 00024 //rainbow 00025 void makeFrame1(unsigned char* data) { 00026 00027 static int time=0; 00028 time++; 00029 00030 // override data with a intensity gradient test pattern 00031 for (int x=0; x<3*16; x++) { 00032 for (int y=0; y<48; y++) { 00033 00034 int i = (x + y*(16*3)) * 3; // figure out the memory location 00035 00036 data[i] = lut_sin((x+y)*255/48+(time/2)%256); //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00037 data[i+1] = lut_sin(((x+y)*255/48+(time/2)+ 85)%256); //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00038 data[i+2] = lut_sin(((x+y)*255/48+(time/2)+170)%256); //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00039 } 00040 } 00041 00042 } 00043 00044 //cool lines 00045 void makeFrame2(unsigned char* data) { 00046 00047 static int time=0; 00048 time++; 00049 00050 // override data with a intensity gradient test pattern 00051 for (int x=0; x<3*16; x++) { 00052 for (int y=0; y<16; y++) { 00053 00054 int i = (x + y*(16*3)) * 3; // figure out the memory location 00055 00056 data[i] = lut_sin(x*255/48+(time)%256 + y*16); //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00057 data[i+1] = lut_sin(x*255/48+(time)%256 + y*16 + 85); //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00058 data[i+2] = lut_sin(x*255/48+(time)%256 + y*16 + 170); //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00059 } 00060 } 00061 00062 } 00063 00064 // static gradients 00065 void makeFrame3(unsigned char* data) { 00066 00067 static int time=0; 00068 time++; 00069 00070 // override data with a intensity gradient test pattern 00071 for (int x=0; x<3*16; x++) { 00072 for (int y=0; y<16; y++) { 00073 00074 int i = (x + y*(16*3)) * 3; // figure out the memory location 00075 00076 data[i] = x*256/48; //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00077 data[i+1] = 0; // green 00078 data[i+2] = y*256/16; //(i/3)%256 ; // blue 00079 } 00080 } 00081 00082 } 00083 00084 00085 void makeFrame33(unsigned char* data) { 00086 00087 static int time=0; 00088 time++; 00089 00090 for (int x=0; x<3*16; x++) { 00091 for (int y=0; y<48; y++) { 00092 00093 int i = (x + y*(16*3)) * 3; // figure out the memory location 00094 00095 data[i] = x*256/48; //(sin((float)(x+time)/15.0)+1.0)*128 ; // red 00096 data[i+1] = 0; // green 00097 data[i+2] = y*256/48; //(i/3)%256 ; // blue 00098 } 00099 } 00100 } 00101 00102 int main() 00103 { 00104 00105 // framebuffer on client side 00106 unsigned char imageSource[256*3*3*3] = { 0 }; 00107 00108 // prepare sin lookup table (optional) 00109 initSinLut(); 00110 00111 // start the screen output, which will keep outputting the frames that are in its internal buffer (updated via .transformFrame) 00112 screen.start(); 00113 00114 while (1) { 00115 00116 makeFrame1(imageSource); // prepare framebuffer with current frame 00117 screen.transformFrame(imageSource); // write framebuffer to output framebuffer 00118 wait_ms(0.5); // slow down the framerate (optional) 00119 } 00120 00121 }
Generated on Thu Jul 28 2022 19:52:51 by
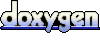