
Test HTTP Server, including RPCFunction
Dependencies: EthernetNetIf mbed HTTPServer
HTTPServer.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "HTTPServer.h" 00004 #include "RPCFunction.h" 00005 00006 EthernetNetIf eth; 00007 00008 HTTPServer svr; 00009 00010 LocalFileSystem fs("webfs"); 00011 00012 //Create a function of the required format 00013 void testFunc(char * input, char * output); 00014 //Attach it to an RPC object 00015 RPCFunction rpcTestFunc(&testFunc, "testFunc"); 00016 00017 int main() { 00018 00019 Base::add_rpc_class<DigitalOut>(); 00020 Base::add_rpc_class<PwmOut>(); 00021 Base::add_rpc_class<AnalogIn>(); 00022 00023 00024 printf("Setting up...\r\n"); 00025 EthernetErr ethErr = eth.setup(); 00026 if (ethErr) { 00027 printf("Error %d in setup.\r\n", ethErr); 00028 return -1; 00029 } 00030 printf("Setup OK\r\n"); 00031 00032 FSHandler::mount("/webfs", "/"); //Mount /webfs path on web root path 00033 00034 svr.addHandler<SimpleHandler>("/hello"); //Default handler 00035 svr.addHandler<RPCHandler>("/rpc"); 00036 svr.addHandler<FSHandler>("/"); //Default handler 00037 00038 svr.bind(80); 00039 00040 printf("Listening...\r\n"); 00041 00042 Timer tm; 00043 tm.start(); 00044 //Listen indefinitely 00045 while (true) { 00046 Net::poll(); 00047 if (tm.read()>.5) { 00048 tm.start(); 00049 printf("alive!\r\n"); 00050 } 00051 } 00052 00053 return 0; 00054 } 00055 00056 void testFunc(char * input, char * output) { 00057 00058 static int toggle=0; 00059 00060 if (toggle != 0) { 00061 sprintf(output, "Hello"); 00062 toggle = 0; 00063 } else { 00064 sprintf(output, "Bye !"); 00065 toggle = 1; 00066 } 00067 } 00068
Generated on Fri Jul 22 2022 07:46:42 by
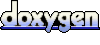