
Sample code for how to erase/write LPC1768, LPC11U24, LPC1114, LPC812 and LPC824 internal flash memory. This program uses IAP call of MCU's ROM routines. The IAP library also supports read/write of EEPROM in LPC11U24.
main.cpp
00001 /** IAP demo : demo code for internal Flash memory access library 00002 * 00003 * The internal Flash memory access is described in the LPC1768 and LPC11U24 usermanual. 00004 * http://www.nxp.com/documents/user_manual/UM10360.pdf 00005 * http://www.nxp.com/documents/user_manual/UM10462.pdf 00006 * 00007 * LPC1768 -- 00008 * Chapter 2: "LPC17xx Memory map" 00009 * Chapter 32: "LPC17xx Flash memory interface and programming" 00010 * refering Rev. 01 - 4 January 2010 00011 * 00012 * LPC11U24 -- 00013 * Chapter 2: "LPC11Uxx Memory mapping" 00014 * Chapter 20: "LPC11Uxx Flash programming firmware" 00015 * refering Rev. 03 - 16 July 2012 00016 * 00017 * This main.cpp demonstrates how the flash can be erased and wrote. 00018 * 00019 * This program tries to... 00020 * 0. read device ID and serial# 00021 * 1. check if the targat sector blank 00022 * 2. erase the sector if it was not blank 00023 * 3. write into the flash (prepare before write) 00024 * 4. verify the data by IAP command 00025 * 5. show the content of the flash 00026 * 00027 * The Flash must be erased as sectors. No overwrite can be done like SRAM. 00028 * So erase should be done in size of 4K or 32K. 00029 * 00030 * Writing sector can be done with size of 256, 512, 1024 or 4096. 00031 * If other size is used, the IAP returns an error. 00032 * The SRAM memory should be allocated in 00033 * 00034 * 00035 * Released under the MIT License: http://mbed.org/license/mit 00036 * 00037 * revision 1.0 09-Mar-2010 1st release 00038 * revision 1.1 12-Mar-2010 chaged: to make possible to reserve flash area for user 00039 * it can be set by USER_FLASH_AREA_START and USER_FLASH_AREA_SIZE in IAP.h 00040 * revision 2.0 26-Nov.2012 LPC11U24 code added 00041 * revision 2.1 26-Nov-2012 EEPROM access code imported from Suga koubou san's (http://mbed.org/users/okini3939/) library 00042 * http://mbed.org/users/okini3939/code/M0_EEPROM_test/ 00043 * revision 3.0 09-Jan-2015 LPC812 and LPC824 support added 00044 * revision 3.1 13-Jan-2015 LPC1114 support added 00045 * revision 3.1.1 16-Jan-2015 Target MCU name changed for better compatibility across the platforms 00046 * revision 3.1.2 10-Mar-2015 merged with pull requests. reinvoke_isp() added and modified read_serial() to return a pointer. 00047 * revision 3.1.2.1 15-Aug-2018 added: just minor feature in "memdump()" function 00048 * revision 3.1.3 16-Aug-2018 "write page" function demo for LPC81X/LPC82X 00049 */ 00050 00051 #include "mbed.h" 00052 #include "IAP.h" 00053 00054 #define MEM_SIZE 256 00055 00056 #if defined(TARGET_LPC176X) 00057 #define TARGET_SECTOR 29 // use sector 29 as target sector if it is on LPC1768 00058 #elif defined(TARGET_LPC11UXX) || defined(TARGET_LPC11XX) 00059 #define TARGET_SECTOR 7 // use sector 7 as target sector if it is on LPC11U24 00060 #define TARGET_EEPROM_ADDRESS 64 00061 #define TARGET_EEPROM_ADDRESS 64 00062 #elif defined(TARGET_LPC81X) || defined(TARGET_LPC82X) 00063 #define TARGET_SECTOR 15 // use sector 15 as target sector if it is on LPC812 00064 #endif 00065 00066 void memdump( char *p, int n ); 00067 int write_page( char *src, int target_page ); 00068 00069 IAP iap; 00070 00071 00072 int main() 00073 { 00074 char mem[ MEM_SIZE ]; // memory, it should be aligned to word boundary 00075 int *serial_number; 00076 int r; 00077 00078 printf( "\r\n\r\n=== IAP: Flash memory writing test ===\r\n" ); 00079 printf( " device-ID = 0x%08X\r\n", iap.read_ID() ); 00080 00081 serial_number = iap.read_serial(); 00082 00083 printf( " serial# =" ); 00084 for ( int i = 0; i < 4; i++ ) 00085 printf( " %08X", *(serial_number + i) ); 00086 printf( "\r\n" ); 00087 00088 printf( " CPU running %dkHz\r\n", SystemCoreClock / 1000 ); 00089 printf( " user reserved flash area: start_address=0x%08X, size=%d bytes\r\n", iap.reserved_flash_area_start(), iap.reserved_flash_area_size() ); 00090 printf( " read_BootVer=0x%08X\r\r\n", iap.read_BootVer() ); 00091 00092 for ( int i = 0; i < MEM_SIZE; i++ ) 00093 mem[ i ] = i & 0xFF; 00094 00095 // blank check: The mbed will erase all flash contents after downloading new executable 00096 00097 r = iap.blank_check( TARGET_SECTOR, TARGET_SECTOR ); 00098 printf( "blank check result = 0x%08X\r\n", r ); 00099 00100 // erase sector, if required 00101 00102 if ( r == SECTOR_NOT_BLANK ) { 00103 iap.prepare( TARGET_SECTOR, TARGET_SECTOR ); 00104 r = iap.erase( TARGET_SECTOR, TARGET_SECTOR ); 00105 printf( "erase result = 0x%08X\r\n", r ); 00106 } 00107 00108 // copy RAM to Flash 00109 00110 iap.prepare( TARGET_SECTOR, TARGET_SECTOR ); 00111 r = iap.write( mem, sector_start_adress[ TARGET_SECTOR ], MEM_SIZE ); 00112 printf( "copied: SRAM(0x%08X)->Flash(0x%08X) for %d bytes. (result=0x%08X)\r\n", mem, sector_start_adress[ TARGET_SECTOR ], MEM_SIZE, r ); 00113 00114 // compare 00115 00116 r = iap.compare( mem, sector_start_adress[ TARGET_SECTOR ], MEM_SIZE ); 00117 printf( "compare result = \"%s\"\r\n", r ? "FAILED" : "OK" ); 00118 00119 //#define WRITE_NEXT_BLOCK 00120 #ifdef WRITE_NEXT_BLOCK 00121 00122 // copy RAM to Flash 00123 00124 iap.prepare( TARGET_SECTOR, TARGET_SECTOR ); 00125 r = iap.write( mem, sector_start_adress[ TARGET_SECTOR ] + 256, MEM_SIZE ); 00126 printf( "copied: SRAM(0x%08X)->Flash(0x%08X) for %d bytes. (result=0x%08X)\r\n", mem, sector_start_adress[ TARGET_SECTOR ], MEM_SIZE, r ); 00127 00128 // compare 00129 00130 r = iap.compare( mem, sector_start_adress[ TARGET_SECTOR ] + 256, MEM_SIZE ); 00131 printf( "compare result = \"%s\"\r\n", r ? "FAILED" : "OK" ); 00132 00133 #endif 00134 00135 printf( "showing the flash contents...\r\n" ); 00136 memdump( sector_start_adress[ TARGET_SECTOR ], MEM_SIZE * 3 ); 00137 00138 00139 #if defined(TARGET_LPC81X) || defined(TARGET_LPC82X) 00140 iap.prepare( TARGET_SECTOR, TARGET_SECTOR ); 00141 r = iap.erase_page( 241, 241 ); // 241 is page number for sector 7 with 64 byte offset 00142 00143 printf( "\r\nerase page test\r\n" ); 00144 printf( "erase page result = \"%s\"\r\n", r ? "FAILED" : "OK" ); 00145 printf( "showing memory dump to confirm 0x00003C40 to 0x00003C7F are erased\r\n(should be changed to 0xFF)\r\n" ); 00146 00147 memdump( sector_start_adress[ TARGET_SECTOR ], MEM_SIZE ); 00148 00149 00150 char test_data[ 64 ]; 00151 00152 for ( int i = 0; i < 64; i++ ) 00153 test_data[ i ] = 0xAA; 00154 00155 r = iap.write_page( test_data, 241 ); // 241 is page number for sector 7 with 64 byte offset 00156 00157 printf( "\r\nwrite page test\r\n" ); 00158 printf( "write page result = \"%s\"\r\n", r ? "FAILED" : "OK" ); 00159 printf( "showing memory dump to confirm 0x00003C40 to 0x00003C7F are written\r\n(should be changed to 0xAA)\r\n" ); 00160 00161 memdump( sector_start_adress[ TARGET_SECTOR ], MEM_SIZE ); 00162 00163 #endif 00164 00165 00166 #if defined(TARGET_LPC11UXX) // SAMPLE OF EEPROM ACCESS (LPC11U24 only) 00167 printf( "IAP: EEPROM writing test\r\n" ); 00168 char mem2[ MEM_SIZE ]; 00169 00170 r = iap.write_eeprom( mem, (char*)TARGET_EEPROM_ADDRESS, MEM_SIZE ); 00171 printf( "copied: SRAM(0x%08X)->EEPROM(0x%08X) for %d bytes. (result=0x%08X)\r\n", mem, TARGET_EEPROM_ADDRESS, MEM_SIZE, r ); 00172 00173 r = iap.read_eeprom( (char*)TARGET_EEPROM_ADDRESS, mem2, MEM_SIZE ); 00174 printf( "copied: EEPROM(0x%08X)->SRAM(0x%08X) for %d bytes. (result=0x%08X)\r\n", TARGET_EEPROM_ADDRESS, mem, MEM_SIZE, r ); 00175 00176 // compare 00177 r = memcmp(mem, mem2, MEM_SIZE); 00178 printf( "compare result = \"%s\"\r\n", r ? "FAILED" : "OK" ); 00179 00180 printf( "showing the EEPROM contents...\r\n" ); 00181 memdump( mem2, MEM_SIZE ); 00182 #endif 00183 00184 printf( "Done! (=== IAP: Flash memory writing test ===)\r\n\r\n" ); 00185 } 00186 00187 #include <ctype.h> 00188 00189 void memdump( char *base, int n ) 00190 { 00191 unsigned int *p; 00192 char s[17] = { '\x0' }; 00193 00194 printf( " memdump from 0x%08X for %d bytes", (unsigned long)base, n ); 00195 00196 p = (unsigned int *)((unsigned int)base & ~(unsigned int)0x3); 00197 00198 for ( int i = 0; i < (n >> 2); i++, p++ ) { 00199 if ( !(i % 4) ) { 00200 printf( " : %s\r\n 0x%08X :", s, (unsigned int)p ); 00201 00202 for ( int j = 0; j < 16; j++) 00203 s[ j ] = isgraph( (int)(*((char *)p + j)) ) ? (*((char *)p + j)) : ' '; 00204 } 00205 printf( " 0x%08X", *p ); 00206 } 00207 printf( " : %s\r\n", s ); 00208 }
Generated on Tue Jul 12 2022 17:32:59 by
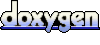