This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
UrlReader.h
00001 #pragma once 00002 00003 namespace MiMic 00004 { 00005 /** 00006 * This class is NULL terminated URL text reader. 00007 * (Currentry, The class can read only absolute URL.) 00008 */ 00009 class UrlReader 00010 { 00011 protected: 00012 const char* _ref_str; 00013 public: 00014 /** 00015 * The constructor 00016 * This function creates reader interface for null terminated text. 00017 * @param i_ref_str 00018 * URL text for read. 00019 * This is referenced pointer. Must hold it until an instance is closed. 00020 * If omited this, Should call setUrl function before call other functions. 00021 */ 00022 UrlReader(const char* i_ref_url=NULL); 00023 /** 00024 * This function sets new text. 00025 * @param i_ref_str 00026 * URL text for read. 00027 * This is referenced pointer. Must hold it until an instance is closed. 00028 */ 00029 void setUrl(const char* i_ref_url); 00030 //bool hasHost(const char* key); 00031 //bool getHost(const char* i_ref_url); 00032 //bool hasPath(const char* i_ref_url); 00033 //bool getPath(const char* i_ref_url); 00034 /** 00035 * This function confirms URL has a query key. 00036 * @param key 00037 * a query key name in text. 00038 * @return 00039 * TRUE if text has key name, otherwise FALSE. 00040 */ 00041 bool hasQueryKey(const char* key); 00042 /** 00043 * This function gets a query key value. 00044 * @param key 00045 * a query key name. 00046 * @param o_ref_value 00047 * address of variable which store a pointer to key value. 00048 * It is part of URL which is not terminated by '\0'. 00049 * @param o_val_len 00050 * length of value text. 00051 * @return 00052 * true if got value. otherwise false. 00053 */ 00054 bool getQueryStr(const char* key,const char* &o_ref_val,int &o_val_len); 00055 /** 00056 * This function gets a converted query key value in unsigned integer. 00057 * @param key 00058 * a query key name. 00059 * @param v 00060 * address of variable which store a pointer to key value. 00061 * @return 00062 * true if got value. otherwise false. 00063 */ 00064 bool getQueryUInt(const char* key,unsigned int &v); 00065 /** 00066 * This function gets a converted query key value in integer. 00067 * @param key 00068 * a query key name. 00069 * @param v 00070 * address of variable which store a pointer to key value. 00071 * @return 00072 * true if got value. otherwise false. 00073 */ 00074 bool getQueryInt(const char* key,int &v); 00075 00076 /** 00077 * This function gets a query key value and check it is equal. 00078 * @param key 00079 * key name. 00080 * @param v 00081 * string to check 00082 * @return 00083 * true if got value that is same as v. otherwise false. 00084 */ 00085 bool isQueryEqualStr(const char* key,const char* v); 00086 /** 00087 * This function gets a query key value and check it is equal. 00088 * @param key 00089 * key name. 00090 * @param v 00091 * string to check 00092 * @return 00093 * true if got value that is same as v. otherwise false. 00094 */ 00095 bool isQueryEqualUInt(const char* key,unsigned int v); 00096 /** 00097 * This function gets a query key value and check it is equal. 00098 * @param key 00099 * key name. 00100 * @param v 00101 * string to check 00102 * @return 00103 * true if got value that is same as v. otherwise false. 00104 */ 00105 bool isQueryEqualInt(const char* key,int v); 00106 /** 00107 * This function gets path part pointer and length from text. 00108 * @param i_path 00109 * address of variable which store a pointer to path part. 00110 * @param i_l 00111 * length of path part. 00112 * @return 00113 * true if got. otherwise false 00114 */ 00115 bool getPath(const char* &path,int &l); 00116 00117 /** 00118 * This function checks whether a path section in URL string is equal. 00119 * 00120 */ 00121 bool isPathEqual(const char* path); 00122 00123 }; 00124 }
Generated on Tue Jul 12 2022 15:46:17 by
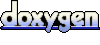