This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
TcpSocket.h
00001 #pragma once 00002 //////////////////////////////////////////////////////////////////////////////// 00003 // TcpSocket.h 00004 //////////////////////////////////////////////////////////////////////////////// 00005 00006 #include "NyLPC_netif.h" 00007 #include "IpAddr.h" 00008 00009 namespace MiMic 00010 { 00011 /** 00012 * Tcp Socket Class. 00013 * The class is used by Net constructor. 00014 */ 00015 class TcpSocket 00016 { 00017 private: 00018 NyLPC_TiTcpSocket_t* _inst; 00019 public: 00020 /** wrapped base LPC class.*/ 00021 NyLPC_TiTcpSocket_t* refBaseInstance(){return this->_inst;} 00022 00023 public: 00024 TcpSocket(); 00025 virtual ~TcpSocket(); 00026 /** 00027 * @param i_host_addr 00028 * must be IPv4 address format. 00029 */ 00030 bool connect (const IpAddr& i_addr,unsigned short i_port); 00031 bool send(const void* i_tx,unsigned short i_tx_size); 00032 /** 00033 * This function return recieved data and size. 00034 * The function sets the head of the readable buffer which can always be read. 00035 * A position is not changed until pseek was called. 00036 * @param i_rx 00037 * address of variable which accepts received data pointer. 00038 * @retrun 00039 * n<-1 Error 00040 * n==0 Timeout (connection still established) 00041 * n>0 Success. readable data size in i_rx. 00042 */ 00043 int precv(const void* &i_rx); 00044 int precv(const char* &i_rx); 00045 /** 00046 * true if precv has data. 00047 * This can avoid the block of precv. 00048 */ 00049 bool canRecv(); 00050 00051 /** 00052 * This function seek rx pointer to next. 00053 * @param i_rx_size 00054 * seek size. Must be returned value which is small or equal by the precv. 00055 */ 00056 void pseek(unsigned short i_rx_seek); 00057 void close(); 00058 }; 00059 00060 }
Generated on Tue Jul 12 2022 15:46:17 by
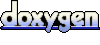