This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_stdlib.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 /** 00027 * @file 00028 * このファイルは、ルートモジュールにあるヘッダファイルを集積します。 00029 * 基本的な型の定義も行います。 00030 */ 00031 #ifndef NyLPC_stdlib_h 00032 #define NyLPC_stdlib_h 00033 #include "NyLPC_config.h" 00034 00035 #include <string.h> 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif /* __cplusplus */ 00040 00041 /********************************************************************** 00042 * 00043 * ASSERT/ABORT 00044 * 00045 **********************************************************************/ 00046 #define MIMIC_DEBUG 1 00047 #ifdef MIMIC_DEBUG 00048 00049 /** 00050 * 引数チェック専用のASSERT構文です。 00051 * デバック時のみ有効です。 00052 */ 00053 #define NyLPC_ArgAssert(e) if(!(e)){NyLPC_assertHook(NULL,-1);}; 00054 00055 /** 00056 * ASSERTマクロです。 00057 * デバック時のみ有効です。 00058 */ 00059 #define NyLPC_Assert(e) if(!(e)){NyLPC_assertHook(NULL,-1);}; 00060 00061 /** 00062 * デバック用のフックマクロです。 00063 * デバック時のみ有効です。 00064 */ 00065 #define NyAR_DebugHook() {NyLPC_debugHook(__FILE__,__LINE__);}; 00066 00067 /** 00068 * Abortマクロです。プログラムを異常終了するときのエントリポイントになります。 00069 * デバック時/リリース時のどちらでも有効です。 00070 */ 00071 #define NyLPC_Abort() {NyLPC_abortHook(__FILE__,__LINE__);}; //もうだめ 00072 00073 /** 00074 * Abortマクロです。eが偽の時に、異常終了します。 00075 * デバック時/リリース時のどちらでも有効です。 00076 * @param e 00077 * 評価式です. 00078 */ 00079 #define NyLPC_AbortIfNot(e) if(!(e)){NyLPC_abortHook(__FILE__,__LINE__);}; 00080 00081 /** 00082 * 警告表示用のマクロです.デバックに使います. 00083 * デバック時のみ有効です. 00084 */ 00085 #define NyLPC_Warning() {NyLPC_debugHook(__FILE__,__LINE__);}; 00086 00087 /** 00088 * 警告表示用のマクロです.eが偽の時に、警告を出します. 00089 * デバック時のみ有効です. 00090 * @param e 00091 * 評価式です。 00092 */ 00093 #define NyLPC_WarningIfNot(e) if(!(e)){NyLPC_debugHook(__FILE__,__LINE__);}; 00094 00095 /* 00096 * トレースマクロです。デバックに使います。 00097 * 内部変数に、最後にコールされたファイル名と、行番号を保存します。 00098 * デバック時のみ有効です. 00099 */ 00100 #define NyLPC_Trace() {NyLPC_debugHook(__FILE__,__LINE__);}; 00101 #else 00102 #define NyLPC_ArgAssert(e) if(!(e)){NyLPC_assertHook(NULL,-1);}; 00103 #define NyLPC_Assert(e) if(!(e)){NyLPC_assertHook(NULL,-1);}; 00104 #define NyAR_DebugHook() {NyLPC_debugHook(__FILE__,__LINE__);}; 00105 #define NyLPC_Abort() {NyLPC_abortHook(__FILE__,__LINE__);}; //もうだめ 00106 #define NyLPC_AbortIfNot(e) if(!(e)){NyLPC_abortHook(__FILE__,__LINE__);}; 00107 #define NyLPC_Warning() {NyLPC_debugHook(__FILE__,__LINE__);}; 00108 #define NyLPC_WarningIfNot(e) if(!(e)){NyLPC_debugHook(__FILE__,__LINE__);}; 00109 #define NyLPC_Trace() {NyLPC_debugHook(__FILE__,__LINE__);}; 00110 #endif 00111 00112 /** 00113 * アサートマクロが使う関数です。 00114 * @param m 00115 * __FILE__を指定します。 00116 * @param l 00117 * __LINE__を指定します。 00118 */ 00119 void NyLPC_assertHook(const char* m,int l); 00120 /** 00121 * Abortマクロが使う関数です。 00122 * @param m 00123 * __FILE__を指定します。 00124 * @param l 00125 * __LINE__を指定します。 00126 */ 00127 void NyLPC_abortHook(const char* m,int l); 00128 /** 00129 * Debugマクロが使う関数です。 00130 * @param m 00131 * __FILE__を指定します。 00132 * @param l 00133 * __LINE__を指定します。 00134 */ 00135 void NyLPC_debugHook(const char* m,int l); 00136 00137 /** 00138 * デバック用のステータス変数です。 00139 * アサートを検出した回数を保存します。 00140 */ 00141 extern unsigned int NyLPC_assert_counter; 00142 /** 00143 * デバック用のステータス変数です。 00144 * アサートを検出した回数を保存します。 00145 */ 00146 extern unsigned int NyLPC_abort_counter; 00147 /** 00148 * デバック用のステータス変数です。 00149 * アサートを検出した回数を保存します。 00150 */ 00151 extern unsigned int NyLPC_debug_counter; 00152 00153 /** 00154 * GOTO方式のエラーハンドラを記述するためのマクロです。 00155 * @param l 00156 * 指定ラベルへジャンプします。 00157 */ 00158 #define NyLPC_OnErrorGoto(l) goto l 00159 00160 00161 00162 /********************************************************************** 00163 * 00164 * Basic type 00165 * 00166 **********************************************************************/ 00167 00168 /** 00169 * 真偽値型です。 00170 */ 00171 typedef long NyLPC_TBool; 00172 00173 /** 00174 * TRUEを表します。この値は、ifで評価したときに真を返すことを保障します。 00175 */ 00176 #define NyLPC_TBool_TRUE (0==0) 00177 /** 00178 * FALSEを表します。この値は、ifで評価したときに偽を返すことを保障します。 00179 */ 00180 #define NyLPC_TBool_FALSE (0!=0) 00181 00182 /** 00183 * ウェーイを表します。この値は、NyLPC_TBool_FALSEと同じです。 00184 */ 00185 #define NyLPC_TBool_VEII NyLPC_TBool_FALSE 00186 00187 00188 00189 /** 00190 * 8bit長のバイト文字の型です。 00191 */ 00192 typedef char NyLPC_TChar; 00193 /** 00194 * 符号有り8bit型です。 00195 */ 00196 typedef signed char NyLPC_TInt8; 00197 /** 00198 * 符号有り16bit型です。 00199 */ 00200 typedef signed short NyLPC_TInt16; 00201 /** 00202 * 符号有り32bit型です。 00203 */ 00204 typedef signed long NyLPC_TInt32; 00205 00206 //---------------------------------------------------------------------- 00207 // NyLPC_TUInt8 00208 //---------------------------------------------------------------------- 00209 00210 /** 00211 * 符号無し8bit型です。 00212 */ 00213 typedef unsigned char NyLPC_TUInt8; 00214 00215 /** 00216 * ビット操作関数です。bfのビット番号bに、1をセットします。 00217 * @bf 00218 * 操作対象の変数です。 00219 * @b 00220 * 操作するビットパターンです。 00221 */ 00222 #define NyLPC_TUInt8_setBit(bf,b) NyLPC_TUInt32_setBit(bf,b) 00223 /** 00224 * ビット操作関数です。bfのビット番号bに、0をセットします。 00225 * @bf 00226 * 操作対象の変数です。 00227 * @b 00228 * 操作するビットパターンです。 00229 */ 00230 #define NyLPC_TUInt8_unsetBit(bf,b) NyLPC_TUInt32_unsetBit(bf,b) 00231 /** 00232 * ビット判定関数です。bfのビット番号bが1であるかを確認します。 00233 * @bf 00234 * 判定する変数です。 00235 * @b 00236 * 判定するビットパターンです。 00237 * @return 00238 * ビットが一致するなら真を返します。 00239 */ 00240 #define NyLPC_TUInt8_isBitOn(bf,b) NyLPC_TUInt32_isBitOn(bf,b) 00241 00242 /** 00243 * 8bit長のTRUE値です。 00244 */ 00245 #define NyLPC_TUInt8_TRUE NyLPC_TBool_TRUE 00246 /** 00247 * 8bit長のFALSE値です。 00248 */ 00249 #define NyLPC_TUInt8_FALSE NyLPC_TBool_FALSE 00250 /** 00251 * 8bit長のFALSEをNyLPC_TBoolへ変換します。 00252 * @param a 00253 * 変換する変数です。 00254 * @return 00255 * 変換した値です。 00256 */ 00257 #define NyLPC_TUInt8_castto_TBool(a) ((a)?NyLPC_TBool_TRUE:NyLPC_TBool_FALSE) 00258 00259 //---------------------------------------------------------------------- 00260 // NyLPC_TUInt16 00261 //---------------------------------------------------------------------- 00262 00263 /** 00264 * 符号無し16bit型です。 00265 */ 00266 typedef unsigned short NyLPC_TUInt16; 00267 00268 /** 00269 * INTMAX 00270 */ 00271 #define NyLPC_TUInt16_MAX 0xFFFF 00272 00273 /** 00274 * ビット操作関数です。bfのビット番号bに、1をセットします。 00275 * @bf 00276 * 操作対象の変数です。 00277 * @b 00278 * 操作するビット番号です。 00279 */ 00280 #define NyLPC_TUInt16_setBit(bf,b) NyLPC_TUInt32_setBit(bf,b) 00281 /** 00282 * ビット操作関数です。bfのビット番号bに、0をセットします。 00283 * @bf 00284 * 操作対象の変数です。 00285 * @b 00286 * 操作するビット番号です。 00287 */ 00288 #define NyLPC_TUInt16_unsetBit(bf,b) NyLPC_TUInt32_unsetBit(bf,b) 00289 /** 00290 * ビット判定関数です。bfのビット番号bが1であるかを確認します。 00291 * @bf 00292 * 判定する変数です。 00293 * @b 00294 * 判定するビット番号です。 00295 * @return 00296 * ビットが一致するなら真を返します。 00297 */ 00298 #define NyLPC_TUInt16_isBitOn(bf,b) NyLPC_TUInt32_isBitOn(bf,b) 00299 00300 /** 00301 * バイトオーダーを入れ替えます。 00302 * @param n 00303 * 変換もとの変数です。 00304 * @return 00305 * 入れ替えた16ビット値を返します。 00306 * 00307 */ 00308 #define NyLPC_TUInt16_BSWAP(n) (((((NyLPC_TUInt16)(n))<< 8)&0xff00)|((((NyLPC_TUInt16)(n))>> 8)&0x00ff)) 00309 00310 00311 00312 /** 00313 * バイトオーダーを入れ替えます。 00314 * NyLPC_TUInt16_BSWAPとの違いは、関数であることです。 00315 * @param n 00316 * 変換もとの変数です。 00317 * @return 00318 * 入れ替えた16ビット値を返します。 00319 */ 00320 NyLPC_TUInt16 NyLPC_TUInt16_bswap(NyLPC_TUInt16 n); 00321 /** 00322 * 16bit長のTRUE値です。 00323 */ 00324 #define NyLPC_TUInt16_TRUE NyLPC_TBool_TRUE 00325 /** 00326 * 16bit長のFALSE値です。 00327 */ 00328 #define NyLPC_TUInt16_FALSE NyLPC_TBool_FALSE 00329 /** 00330 * 16bit長のFALSEをNyLPC_TBoolへ変換します。 00331 * @param a 00332 * 変換する変数です。 00333 * @return 00334 * 変換した値です。 00335 */ 00336 #define NyLPC_TUInt16_castto_TBool(a) ((a)?NyLPC_TBool_TRUE:NyLPC_TBool_FALSE) 00337 00338 //---------------------------------------------------------------------- 00339 00340 /** 00341 * 符号無し32bit型です。 00342 */ 00343 typedef unsigned long NyLPC_TUInt32; 00344 /** 00345 * ビット操作関数です。bfのビットパターンbに、1をセットします。 00346 * @bf 00347 * 操作対象の変数です。 00348 * @b 00349 * 操作するビットパターンです。 00350 */ 00351 #define NyLPC_TUInt32_setBit(bf,b) bf=(bf|(1<<b)) 00352 /** 00353 * ビット操作関数です。bfのビットパターンbに、0をセットします。 00354 * @bf 00355 * 操作対象の変数です。 00356 * @b 00357 * 操作するビットパターンです。 00358 */ 00359 #define NyLPC_TUInt32_unsetBit(bf,b) bf=(bf&(~(1<<b))) 00360 /** 00361 * ビット判定関数です。bfのビットパターンbが1であるかを確認します。 00362 * @bf 00363 * 判定する変数です。 00364 * @b 00365 * 判定するビットパターンです。 00366 * @return 00367 * ビットが一致するなら真を返します。 00368 */ 00369 #define NyLPC_TUInt32_isBitOn(bf,b) ((bf&(1<<b))!=0) 00370 00371 /** 00372 * バイトオーダーを入れ替えます。 00373 * @param n 00374 * 変換もとの変数です。 00375 * @return 00376 * 入れ替えた32ビット値を返します。 00377 * 00378 */ 00379 #define NyLPC_TUInt32_BSWAP(n) (((((NyLPC_TUInt32)(n))<<24)&0xff000000)|((((NyLPC_TUInt32)(n))<< 8)&0x00ff0000)|((((NyLPC_TUInt32)(n))>> 8)&0x0000ff00)|((((NyLPC_TUInt32)(n))>>24)&0x000000ff)) 00380 /** 00381 * バイトオーダーを入れ替えます。 00382 * NyLPC_TUInt32_BSWAPとの違いは、関数であることです。 00383 * @param n 00384 * 変換もとの変数です。 00385 * @return 00386 * 入れ替えた32ビット値を返します。 00387 */ 00388 NyLPC_TUInt32 NyLPC_TUInt32_bswap(NyLPC_TUInt32 n); 00389 00390 /** 00391 * 32bit長のTRUE値です。 00392 */ 00393 #define NyLPC_TUInt32_TRUE NyLPC_TBool_TRUE 00394 /** 00395 * 32bit長のFALSE値です。 00396 */ 00397 #define NyLPC_TUInt32_FALSE NyLPC_TBool_FALSE 00398 /** 00399 * 32bit長のFALSEをNyLPC_TBoolへ変換します。 00400 * @param a 00401 * 変換する変数です。 00402 * @return 00403 * 変換した値です。 00404 */ 00405 #define NyLPC_TUInt32_castto_TBool(a) ((a)?NyLPC_TBool_TRUE:NyLPC_TBool_FALSE) 00406 00407 00408 //---------------------------------------------------------------------- 00409 00410 /** 00411 * 長さ付TChar配列の構造体です。 00412 * 00413 */ 00414 struct NyLPC_TCharArrayPtr 00415 { 00416 /** 配列のポインタ */ 00417 NyLPC_TChar* ptr; 00418 /** 配列の長さ */ 00419 NyLPC_TUInt16 len; 00420 }; 00421 /** 00422 * ptrの位置をi_seekだけ進行します。 00423 * @param i_struct 00424 * 操作する構造体 00425 * @param i_seek 00426 * シークするバイト長 00427 */ 00428 NyLPC_TBool NyLPC_TCharArrayPtr_seek(struct NyLPC_TCharArrayPtr* i_struct,NyLPC_TUInt16 i_seek); 00429 00430 00431 /** 00432 * 長さ付TUInt32配列の構造体です。 00433 * 00434 */ 00435 struct NyLPC_TUInt32ArrayPtr 00436 { 00437 /** 配列のポインタ */ 00438 NyLPC_TUInt32* ptr; 00439 /** 要素数 */ 00440 NyLPC_TUInt16 len; 00441 }; 00442 /** 00443 * ptrの位置をi_seekだけ進行します。 00444 * 進行すると、len要素がi_seekだけ減少します。 00445 * @param i_struct 00446 * 操作する構造体 00447 * @param i_seek 00448 * シークする要素長 00449 */ 00450 NyLPC_TBool NyLPC_TUInt32ArrayPtr_seek(struct NyLPC_TUInt32ArrayPtr* i_struct,NyLPC_TUInt16 i_seek); 00451 /** 00452 * 構造体に、参照するバッファの初期位置とサイズをセットします。 00453 * セットしたバッファは、前方シークの可能な書き込みバッファとして使用できます。 00454 */ 00455 void NyLPC_TUInt32ArrayPtr_setBuf(struct NyLPC_TUInt32ArrayPtr* i_struct,NyLPC_TUInt32* i_ptr,NyLPC_TUInt16 i_len); 00456 00457 00458 /** 00459 * TextとIDのテーブルです。 00460 */ 00461 struct NyLPC_TTextIdTbl{ 00462 const char* n; 00463 NyLPC_TUInt8 id; 00464 }; 00465 00466 /** 00467 * テーブルから文字列に一致するIDを返します。 00468 * 大文字と小文字の区別をしません。 00469 * @return 00470 * 一致する文字列のID 00471 * 一致するものがない場合、テーブルの終端の値を返す。 00472 */ 00473 NyLPC_TUInt8 NyLPC_TTextIdTbl_getMatchId(const NyLPC_TChar* i_str,const struct NyLPC_TTextIdTbl i_tbl[]); 00474 /** 00475 * テーブルから文字列に一致するIDを返します。 00476 * 大文字と小文字の区別をしません。 00477 * @return 00478 * 一致する文字列のID 00479 * 一致するものがない場合、テーブルの終端の値を返す。 00480 */ 00481 NyLPC_TUInt8 NyLPC_TTextIdTbl_getMatchIdIgnoreCase(const NyLPC_TChar* i_str,const struct NyLPC_TTextIdTbl i_tbl[]); 00482 /** 00483 * テーブルからIDに一致する文字列を返す. 00484 * @return 00485 * IDに一致する文字列. 00486 * 存在しなければNULL 00487 */ 00488 const NyLPC_TChar* NyLPC_TTextIdTbl_getTextById(NyLPC_TUInt8 i_id,const struct NyLPC_TTextIdTbl i_tbl[]); 00489 00490 /********************************************************************************* 00491 * standard function 00492 *********************************************************************************/ 00493 /** 00494 * Same as tolower 00495 */ 00496 #define NyLPC_tolower(c) (((c) >= 'A' && (c) <= 'Z' )?((c)+'a' - 'A'):(c)) 00497 00498 /** 00499 * @return 00500 * 書き込んだ文字列の長さ 00501 */ 00502 NyLPC_TInt8 NyLPC_itoa(int i_n,char* o_out,NyLPC_TInt8 i_base); 00503 00504 00505 /** 00506 * @return 00507 * 書き込んだ文字列の長さ 00508 */ 00509 NyLPC_TInt8 NyLPC_uitoa (unsigned int i_n,char* o_out,NyLPC_TInt8 i_base); 00510 /** 00511 * 桁数の指定できるuitoaです。 00512 */ 00513 NyLPC_TInt8 NyLPC_uitoa2(unsigned int i_n,char* o_out,NyLPC_TInt8 i_base,NyLPC_TInt8 i_digit); 00514 00515 00516 /** 00517 * Same as reverse 00518 */ 00519 void NyLPC_reverse(char* s); 00520 00521 /** 00522 * Same as stricmp 00523 */ 00524 int NyLPC_stricmp(const char *i_s1, const char *i_s2); 00525 00526 /** 00527 * Same as strnicmp 00528 */ 00529 int NyLPC_strnicmp(const char *i_s1, const char *i_s2,int n); 00530 00531 /** 00532 * Convert a charactor to integer. 00533 */ 00534 int NyLPC_ctoi(char i); 00535 00536 /** 00537 * Convert a character to 16 digit integer. 00538 */ 00539 int NyLPC_ctox(char i); 00540 00541 /** 00542 * va_copyがない場合の対処だお 00543 */ 00544 #ifndef va_copy 00545 # define NyLPC_va_copy(dest, src) ((dest) = (src)) 00546 #else 00547 # define NyLPC_va_copy(dest, src) va_copy((dest),(src)) 00548 #endif 00549 00550 #ifdef __cplusplus 00551 } 00552 #endif /* __cplusplus */ 00553 00554 00555 00556 /********************************************************************************* 00557 * 00558 *********************************************************************************/ 00559 00560 #include "../NyLPC_cMiMicEnv.h" 00561 #include "../NyLPC_cRingBuffer.h" 00562 #include "../NyLPC_cPtrTbl.h" 00563 #include "../NyLPC_cFifoBuffer.h" 00564 #include "../NyLPC_cPtrStream.h" 00565 #include "../NyLPC_cStr.h" 00566 00567 00568 00569 00570 00571 #endif
Generated on Tue Jul 12 2022 15:46:17 by
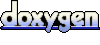