This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_iTcpSocket.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NYLPC_ITCPSOCKET_H_ 00027 #define NYLPC_ITCPSOCKET_H_ 00028 00029 00030 #include "NyLPC_stdlib.h" 00031 #include "NyLPC_os.h" 00032 #include "NyLPC_NetIf_ip_types.h" 00033 00034 #ifdef __cplusplus 00035 extern "C" { 00036 #endif /* __cplusplus */ 00037 00038 typedef struct NyLPC_TiTcpSocket NyLPC_TiTcpSocket_t; 00039 00040 /********************************************************************** 00041 * Function 00042 **********************************************************************/ 00043 00044 00045 typedef const struct NyLPC_TIPv4Addr* (*NyLPC_TiTcpSocket_getPeerAddr)(const NyLPC_TiTcpSocket_t* i_inst); 00046 typedef NyLPC_TUInt16 (*NyLPC_TiTcpSocket_getPeerPort)(const NyLPC_TiTcpSocket_t* i_inst); 00047 00048 00049 typedef NyLPC_TBool (*NyLPC_TiTcpSocket_accept)(NyLPC_TiTcpSocket_t* i_inst,NyLPC_TUInt32 i_wait_in_msec); 00050 /** 00051 * @return 00052 * 1 - 以上:受信に成功した。 00053 * 0 - タイムアウト 00054 * -1 - ソケットがクローズしている 00055 */ 00056 typedef NyLPC_TInt32 (*NyLPC_TiTcpSocket_precv)(NyLPC_TiTcpSocket_t* i_inst,const void** o_buf_ptr,NyLPC_TUInt32 i_wait_msec); 00057 typedef void (*NyLPC_TiTcpSocket_pseek)(NyLPC_TiTcpSocket_t* i_inst,NyLPC_TUInt16 i_seek); 00058 /** 00059 * 送信未達は保障されません。 00060 * エラーを検出したら、基本的にはソケットをクローズしてください。 00061 * @param i_wait_msec 00062 * 送信失敗までの待ち時間を指定します。現在は、 00063 * RTT推定ができないため、TCPの再送を考慮して、最低でも10秒(10000)程度を指定してください。 00064 * @return 00065 * 送信したバイト数を返します。エラーならば0未満の数を返します。 00066 * 00067 */ 00068 typedef NyLPC_TInt32(*NyLPC_TiTcpSocket_send)(NyLPC_TiTcpSocket_t* i_inst,const void* i_buf_ptr,NyLPC_TInt32 i_len,NyLPC_TUInt32 i_wait_in_msec); 00069 typedef void(*NyLPC_TiTcpSocket_close)(NyLPC_TiTcpSocket_t* i_inst,NyLPC_TUInt32 i_wait_in_msec); 00070 00071 00072 /** 00073 * NyLPC_iTcpSocket_psendで送信するための送信バッファ準備します。 00074 * @param i_hint 00075 * 送信を希望するデータサイズを指定します。 00076 * アロケータは出来る限り希望に沿ってメモリを返します。 00077 * @param o_buf_size 00078 * 取得できたバッファサイズを返します。 00079 * @return 00080 * 成功した場合、送信バッファを返します。 00081 * アプリケーションは、可能な限り速やかにデータを書き込んで、NyLPC_iTcpSocket_psendをコールしてください。 00082 * @note 00083 * Optionフィールドを持つパケットを送信する場合は、オプションデータサイズの合計をデータサイズに指定して、payloadwriterで調整すること。 00084 */ 00085 typedef void* (*NyLPC_TiTcpSocket_allocSendBuf)(NyLPC_TiTcpSocket_t* i_inst,NyLPC_TUInt16 i_hint,NyLPC_TUInt16* o_buf_size,NyLPC_TUInt32 i_wait_in_msec); 00086 00087 /** 00088 * NyLPC_iTcpSocket_allocSendBufで確保したメモリを開放します。 00089 */ 00090 typedef void (*NyLPC_TiTcpSocket_releaseSendBuf)(NyLPC_TiTcpSocket_t* i_inst,void* i_buf_ptr); 00091 00092 /** 00093 * 事前にAllocしたTxパケットを送信します。 00094 * このAPIはゼロコピー送信をサポートするためのものです。 00095 * @param i_buf_ptr 00096 * allocSendBufで取得したメモリを指定します。 00097 * @return 00098 * 失敗した場合、メモリは開放されません。 00099 */ 00100 typedef NyLPC_TBool (*NyLPC_TiTcpSocket_psend)(NyLPC_TiTcpSocket_t* i_inst,void* i_buf_ptr,int i_len,NyLPC_TUInt32 i_wait_in_msec); 00101 00102 /** 00103 * TCPソケットをクライアントとしてサーバへ接続します。 00104 */ 00105 typedef NyLPC_TBool (*NyLPC_TiTcpSocket_connect)(NyLPC_TiTcpSocket_t* i_inst,const struct NyLPC_TIPv4Addr* i_addr,NyLPC_TUInt16 i_peer_port,NyLPC_TUInt32 i_wait_in_msec); 00106 00107 typedef void (*NyLPC_TiTcpSocket_finalize)(NyLPC_TiTcpSocket_t* i_inst); 00108 00109 /********************************************************************** 00110 * Interface 00111 **********************************************************************/ 00112 00113 /** 00114 */ 00115 struct NyLPC_TiTcpSocket_Interface 00116 { 00117 NyLPC_TiTcpSocket_getPeerAddr getpeeraddr; 00118 NyLPC_TiTcpSocket_getPeerPort getpeerport; 00119 NyLPC_TiTcpSocket_accept accept; 00120 NyLPC_TiTcpSocket_precv precv; 00121 NyLPC_TiTcpSocket_pseek pseek; 00122 NyLPC_TiTcpSocket_send send; 00123 NyLPC_TiTcpSocket_close close; 00124 NyLPC_TiTcpSocket_allocSendBuf allocSendBuf; 00125 NyLPC_TiTcpSocket_releaseSendBuf releaseSendBuf; 00126 NyLPC_TiTcpSocket_psend psend; 00127 NyLPC_TiTcpSocket_connect connect; 00128 NyLPC_TiTcpSocket_finalize finalize; 00129 }; 00130 00131 struct NyLPC_TiTcpSocket 00132 { 00133 const struct NyLPC_TiTcpSocket_Interface* _interface; 00134 }; 00135 00136 00137 #define NyLPC_iTcpSocket_getPeerAddr(i_inst) ((i_inst)->_interface->getpeeraddr((i_inst))) 00138 #define NyLPC_iTcpSocket_getPeerPort(i_inst) ((i_inst)->_interface->getpeerport((i_inst))) 00139 #define NyLPC_iTcpSocket_accept(i_inst,i_wait_in_msec) ((i_inst)->_interface->accept((i_inst),(i_wait_in_msec))) 00140 #define NyLPC_iTcpSocket_precv(i_inst,o_buf_ptr,i_wait_msec) ((i_inst)->_interface->precv((i_inst),(o_buf_ptr),(i_wait_msec))) 00141 #define NyLPC_iTcpSocket_pseek(i_inst,i_seek) ((i_inst)->_interface->pseek((i_inst),(i_seek))) 00142 #define NyLPC_iTcpSocket_send(i_inst,i_buf_ptr,i_len,i_wait_in_msec) ((i_inst)->_interface->send((i_inst),(i_buf_ptr),(i_len),(i_wait_in_msec))) 00143 #define NyLPC_iTcpSocket_close(i_inst,i_wait_in_msec) ((i_inst)->_interface->close((i_inst),(i_wait_in_msec))) 00144 #define NyLPC_iTcpSocket_allocSendBuf(i_inst,i_hint,o_buf_size,i_wait_in_msec) ((i_inst)->_interface->allocSendBuf((i_inst),(i_hint),(o_buf_size),(i_wait_in_msec))) 00145 #define NyLPC_iTcpSocket_releaseSendBuf(i_inst,i_buf_ptr) ((i_inst)->_interface->releaseSendBuf((i_inst),(i_buf_ptr))) 00146 #define NyLPC_iTcpSocket_psend(i_inst,i_buf_ptr,i_len,i_wait_in_msec) ((i_inst)->_interface->psend((i_inst),(i_buf_ptr),(i_len),(i_wait_in_msec))) 00147 #define NyLPC_iTcpSocket_connect(i_inst,i_addr,i_peer_port,i_wait_in_msec) ((i_inst)->_interface->connect((i_inst),(i_addr),(i_peer_port),(i_wait_in_msec))) 00148 #define NyLPC_iTcpSocket_finalize(i_inst) ((i_inst)->_interface->finalize((i_inst))) 00149 00150 #ifdef __cplusplus 00151 } 00152 #endif /* __cplusplus */ 00153 00154 #endif /* NYLPC_ITCPSOCKET_H_ */ 00155
Generated on Tue Jul 12 2022 15:46:17 by
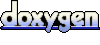