This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_iNetInterface.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NYLPC_INETINTERFACE_H_ 00027 #define NYLPC_INETINTERFACE_H_ 00028 00029 00030 #include "NyLPC_stdlib.h" 00031 #include "NyLPC_os.h" 00032 #include "NyLPC_iTcpSocket.h" 00033 #include "NyLPC_iUdpSocket.h" 00034 #include "NyLPC_iTcpListener.h" 00035 #include "NyLPC_cIPv4Config.h" 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif /* __cplusplus */ 00039 00040 struct NyLPC_TNetInterfaceInfo{ 00041 const char* device_name; 00042 const NyLPC_TcIPv4Config_t* current_config; 00043 }; 00044 00045 /** 00046 * ソケット生成のヒント値 00047 */ 00048 typedef NyLPC_TUInt16 NyLPC_TSocketType; 00049 #define NyLPC_TSocketType_TCP_NORMAL 0x0100 00050 #define NyLPC_TSocketType_TCP_HTTP 0x0101 00051 #define NyLPC_TSocketType_UDP_NORMAL 0x0200 00052 #define NyLPC_TSocketType_UDP_NOBUF 0x0202 00053 /********************************************************************** 00054 * Function 00055 **********************************************************************/ 00056 00057 /** 00058 * 制限時間付きでソケットに接続を待ちます。 00059 */ 00060 typedef NyLPC_TiTcpSocket_t* (*NyLPC_TiNetInterface_createTcpSocetEx)(NyLPC_TSocketType i_socktype); 00061 typedef NyLPC_TiUdpSocket_t* (*NyLPC_TiNetInterface_createUdpSocetEx)(NyLPC_TUInt16 i_port,NyLPC_TSocketType i_socktype); 00062 typedef NyLPC_TiTcpListener_t* (*NyLPC_TiNetInterface_createTcpListener)(NyLPC_TUInt16 i_port); 00063 /** 00064 * Start関数はイベント関数の定期コールが開始される前に呼び出されます。 00065 */ 00066 typedef void (*NyLPC_TiNetInterface_start)(const NyLPC_TcIPv4Config_t* i_cfg); 00067 typedef void (*NyLPC_TiNetInterface_stop)(void); 00068 00069 typedef void(*NyLPC_TiNetInterface_sendArpRequest)(const struct NyLPC_TIPv4Addr* i_addr); 00070 typedef NyLPC_TBool(*NyLPC_TiNetInterface_hasArpInfo)(const struct NyLPC_TIPv4Addr* i_addr); 00071 typedef NyLPC_TBool(*NyLPC_TiNetInterface_isInitService)(void); 00072 00073 typedef const struct NyLPC_TNetInterfaceInfo* (*NyLPC_TiNetInterface_getInterfaceInfo)(void); 00074 00075 /********************************************************************** 00076 * Interface 00077 **********************************************************************/ 00078 struct NyLPC_TiNetInterface_Interface 00079 { 00080 NyLPC_TiNetInterface_createTcpSocetEx createTcpSocketEx; 00081 NyLPC_TiNetInterface_createUdpSocetEx createUdpSocetEx; 00082 NyLPC_TiNetInterface_createTcpListener createTcpListener; 00083 NyLPC_TiNetInterface_start start; 00084 NyLPC_TiNetInterface_stop stop; 00085 NyLPC_TiNetInterface_sendArpRequest sendarprequest; 00086 NyLPC_TiNetInterface_hasArpInfo hasarpinfo; 00087 NyLPC_TiNetInterface_isInitService isinitservice; 00088 NyLPC_TiNetInterface_getInterfaceInfo getinterfaceinfo; 00089 }; 00090 00091 00092 00093 #ifdef __cplusplus 00094 } 00095 #endif /* __cplusplus */ 00096 00097 #endif /* NYLPC_CTCPLISTENER_H_ */ 00098
Generated on Tue Jul 12 2022 15:46:17 by
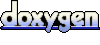