This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_iHttpPtrStream.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NyLPC_TiHttpPtrStream_H_ 00027 #define NyLPC_TiHttpPtrStream_H_ 00028 00029 /********************************************************************** 00030 * 00031 * NyLPC_TiHttpPtrStream class 00032 * 00033 **********************************************************************/ 00034 #include "NyLPC_stdlib.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif /* __cplusplus */ 00039 00040 /** 00041 * Httpストリームのインタフェイス。 00042 */ 00043 typedef struct NyLPC_TiHttpPtrStream NyLPC_TiHttpPtrStream_t; 00044 00045 /** 00046 * ストリームのエンコーディングモード 00047 */ 00048 typedef NyLPC_TUInt8 NyLPC_TiHttpPtrStream_ET; 00049 #define NyLPC_TiHttpPtrStream_ET_NONE 0x00 00050 #define NyLPC_TiHttpPtrStream_ET_CHUNKED 0x01 00051 00052 /** 00053 * HTTP通信タイムアウトのデフォルト値 00054 */ 00055 #define NyLPC_TiHttpPtrStream_DEFAULT_HTTP_TIMEOUT (8*1000) 00056 00057 00058 typedef NyLPC_TInt32 (*NyLPC_TiHttpPtrStream_pread)(void* i_inst,const void** o_buf_ptr,NyLPC_TUInt32 i_timeout); 00059 typedef NyLPC_TBool (*NyLPC_TiHttpPtrStream_write)(void* i_inst,const void* i_data,NyLPC_TInt32 i_length); 00060 typedef void (*NyLPC_TiHttpPtrStream_rseek)(void* i_inst,NyLPC_TUInt16 i_seek); 00061 typedef NyLPC_TBool (*NyLPC_TiHttpPtrStream_flush)(void* i_inst); 00062 typedef void (*NyLPC_TiHttpPtrStream_setReadEncoding)(void* i_inst,NyLPC_TiHttpPtrStream_ET i_et); 00063 typedef void (*NyLPC_TiHttpPtrStream_setWriteEncoding)(void* i_inst,NyLPC_TiHttpPtrStream_ET i_et); 00064 00065 /** 00066 * _interface_httpptrstreamで宣言してください。 00067 */ 00068 struct NyLPC_TiHttpPtrStream_TInterface 00069 { 00070 NyLPC_TiHttpPtrStream_pread pread; 00071 NyLPC_TiHttpPtrStream_write write; 00072 NyLPC_TiHttpPtrStream_rseek readSeek; 00073 NyLPC_TiHttpPtrStream_flush flush; 00074 NyLPC_TiHttpPtrStream_setReadEncoding setreadencoding; 00075 NyLPC_TiHttpPtrStream_setWriteEncoding setwriteencoding; 00076 }; 00077 struct NyLPC_TiHttpPtrStream 00078 { 00079 /** 00080 * 継承クラスで実装すべきハンドラ 00081 */ 00082 const struct NyLPC_TiHttpPtrStream_TInterface* absfunc; 00083 }; 00084 00085 /** 00086 * ストリームからデータを読み出して、そのポインタを返します。 00087 * @return 00088 * ストリームから読み込んだデータサイズを返します。 00089 * 0の場合はタイムアウトです。 00090 * 0未満の場合はエラーです。 00091 */ 00092 #define NyLPC_iHttpPtrStream_pread(i_inst,o_buf_ptr,i_timeout) (i_inst)->absfunc->pread((i_inst),(o_buf_ptr),i_timeout) 00093 /** 00094 * ストリームへデータを書き込みます。 00095 * @param i_length 00096 * i_dataのデータ長を指定します。-1の場合、strlenを実行します。 00097 * @return 00098 * 規定時間内にストリームへの書き込みが完了すると、TRUEを返します。 00099 */ 00100 #define NyLPC_iHttpPtrStream_write(i_inst,i_data,i_length) (i_inst)->absfunc->write((i_inst),i_data,i_length) 00101 #define NyLPC_iHttpPtrStream_rseek(i_inst,i_seek) (i_inst)->absfunc->readSeek((i_inst),(i_seek)) 00102 /** 00103 * バッファに残っているデータを送信し、空にします。 00104 */ 00105 #define NyLPC_iHttpPtrStream_flush(i_inst) (i_inst)->absfunc->flush(i_inst) 00106 00107 /** 00108 * 読み出しエンコーディングの設定。 00109 * この関数は削除するかもしれない。使用しないこと。 00110 * @bug 関数が非対称。cHttpBasicBodyParserがチャンク読み出し処理を肩代わりしている? 00111 */ 00112 #define NyLPC_iHttpPtrStream_setReadEncoding(i_inst,i_et) (i_inst)->absfunc->setreadencoding((i_inst),i_et) 00113 /** 00114 * 書込みエンコーディングの設定。 00115 */ 00116 #define NyLPC_iHttpPtrStream_setWriteEncoding(i_inst,i_et) (i_inst)->absfunc->setwriteencoding((i_inst),i_et) 00117 00118 #ifdef __cplusplus 00119 } 00120 #endif /* __cplusplus */ 00121 00122 #endif /* NyLPC_TiHttpPtrStream_H_ */
Generated on Tue Jul 12 2022 15:46:17 by
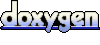