This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cStopwatch.cpp
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #include "../NyLPC_cStopwatch.h" 00027 #if NyLPC_ARCH==NyLPC_ARCH_MBEDRTOS 00028 #include "mbed.h" 00029 #include "rtos.h" 00030 00031 static Timer _timer; 00032 static NyLPC_TBool _is_start=NyLPC_TBool_FALSE; 00033 /** 00034 * 現在のtickCountを返します。 00035 */ 00036 NyLPC_TUInt32 NyLPC_cStopwatch_now(void) 00037 { 00038 return (NyLPC_TUInt32)(_timer. read_ms()); 00039 } 00040 00041 /** 00042 * インスタンスを生成します。 00043 */ 00044 void NyLPC_cStopwatch_initialize(NyLPC_TcStopwatch_t* i_inst) 00045 { 00046 if(!_is_start){ 00047 _timer.start(); 00048 _is_start=NyLPC_TBool_TRUE; 00049 } 00050 return; 00051 } 00052 /** 00053 * 基準時刻をセットします。 00054 * 値は、システム依存のtick値です。 00055 */ 00056 void NyLPC_cStopwatch_set(NyLPC_TcStopwatch_t* i_inst,NyLPC_TUInt32 i_initial) 00057 { 00058 i_inst->_tick=i_initial; 00059 return; 00060 } 00061 00062 /** 00063 * 基準時刻に、現在時刻をセットします。 00064 */ 00065 void NyLPC_cStopwatch_setNow(NyLPC_TcStopwatch_t* i_inst) 00066 { 00067 i_inst->_tick=NyLPC_cStopwatch_now(); 00068 } 00069 /** 00070 * タイムアウト計測を開始します。 00071 * この関数は、基準時刻に現在の時刻をセットします。 00072 */ 00073 void NyLPC_cStopwatch_startExpire(NyLPC_TcStopwatch_t* i_inst,NyLPC_TUInt32 i_timeout) 00074 { 00075 NyLPC_cStopwatch_setNow(i_inst); 00076 i_inst->_ex_timeout=i_timeout; 00077 } 00078 00079 /** 00080 * NyLPC_cStopwatch_startExpireで設定した時間を経過したかを返します。 00081 */ 00082 NyLPC_TBool NyLPC_cStopwatch_isExpired(NyLPC_TcStopwatch_t* i_inst) 00083 { 00084 //経過時間の判定 00085 if(NyLPC_cStopwatch_elapseInMsec(i_inst)>i_inst->_ex_timeout){ 00086 i_inst->_ex_timeout=0; 00087 } 00088 return (i_inst->_ex_timeout==0); 00089 } 00090 00091 /** 00092 * 基準時刻と現在時刻の差を計算して、経過時間をミリ秒で返します。 00093 */ 00094 NyLPC_TUInt32 NyLPC_cStopwatch_elapseInMsec(const NyLPC_TcStopwatch_t* i_inst) 00095 { 00096 return (NyLPC_cStopwatch_now()-i_inst->_tick); 00097 } 00098 00099 #endif
Generated on Tue Jul 12 2022 15:46:16 by
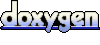