This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cPtrStream.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NYLPC_TCPTRSTREAM_H_ 00027 #define NYLPC_TCPTRSTREAM_H_ 00028 00029 /********************************************************************** 00030 * 00031 * NyLPC_TcPtrStream class 00032 * 00033 **********************************************************************/ 00034 #include "NyLPC_stdlib.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif /* __cplusplus */ 00039 00040 /** 00041 * ブロッキングストリームのベース型です。 00042 */ 00043 typedef struct NyLPC_TcPtrStream NyLPC_TcPtrStream_t; 00044 00045 00046 typedef NyLPC_TInt32 (*NyLPC_TcPtrStream_pread)(NyLPC_TcPtrStream_t* i_inst,const void** o_buf_ptr,NyLPC_TUInt32 i_wait_msec); 00047 typedef NyLPC_TBool (*NyLPC_TcPtrStream_write)(NyLPC_TcPtrStream_t* i_inst,const void* i_data,NyLPC_TInt16 i_length,NyLPC_TUInt32 i_wait_msec); 00048 typedef void (*NyLPC_TcPtrStream_rseek)(NyLPC_TcPtrStream_t* i_inst,NyLPC_TUInt16 i_seek); 00049 typedef void (*NyLPC_TcPtrStream_close)(NyLPC_TcPtrStream_t* i_inst); 00050 00051 struct NyLPC_TcPtrStream_TInterface 00052 { 00053 NyLPC_TcPtrStream_pread pread; 00054 NyLPC_TcPtrStream_write write; 00055 NyLPC_TcPtrStream_rseek readSeek; 00056 NyLPC_TcPtrStream_close close; 00057 }; 00058 00059 00060 struct NyLPC_TcPtrStream 00061 { 00062 const struct NyLPC_TcPtrStream_TInterface* _interface; 00063 }; 00064 00065 /** 00066 * ストリームからデータを読み出して、そのポインタを返します。 00067 * @return 00068 * ストリームから読み込んだデータサイズを返します。 00069 * 0の場合はタイムアウトです。 00070 * 0未満の場合はエラーです。 00071 */ 00072 #define NyLPC_cPtrStream_read(i_inst,o_buf_ptr,i_wait_msec) (i_inst)->_interface->read((i_inst),o_buf_ptr,i_wait_msec) 00073 /** 00074 * ストリームへデータを書き込みます。 00075 * @param i_length 00076 * i_dataのデータ長を指定します。-1の場合、strlenを実行します。 00077 * @return 00078 * 規定時間内にストリームへの書き込みが完了すると、TRUEを返します。 00079 */ 00080 #define NyLPC_cPtrStream_write(i_inst,i_data,i_length,i_wait_msec) (i_inst)->_interface->write((i_inst),i_data,i_length,i_wait_msec) 00081 00082 /** 00083 * 改行付でストリームへ書き込みます。 00084 */ 00085 NyLPC_TBool NyLPC_cPtrStream_writeln(NyLPC_TcPtrStream_t* i_inst,const void* i_data,NyLPC_TInt16 i_length,NyLPC_TUInt32 i_wait_msec); 00086 /** 00087 * 数値をストリームへ書き込みます。 00088 * @param i_base 00089 * 10以上を指定してください。 00090 */ 00091 NyLPC_TBool NyLPC_cPtrStream_writeInt(NyLPC_TcPtrStream_t* i_inst,NyLPC_TInt32 i_val,NyLPC_TUInt32 i_wait_msec,NyLPC_TUInt32 i_base); 00092 00093 /** 00094 * 書き込みメモリを要求します。 00095 * @return 00096 * 書き込みメモリの先頭ポインタを返します。この値は次回のpwriteに入力してください。 00097 */ 00098 #define NyLPC_cPtrStream_wreserv(i_inst,i_hint,o_len) 00099 00100 00101 #define NyLPC_cPtrStream_rseek(i_inst,i_seek) (i_inst)->_interface->preadSeek((i_inst),i_seek) 00102 /** 00103 ストリームに対する操作を閉じます。 00104 readの場合は占有している共有メモリの解放、ブロックしているタスクの解放をします。 00105 writeの場合はバッファに残っているデータの書き込みを保証します。 00106 */ 00107 #define NyLPC_cPtrStream_close(i_inst) i_inst->_interface->close(i_inst) 00108 00109 #ifdef __cplusplus 00110 } 00111 #endif /* __cplusplus */ 00112 00113 #endif /* NYLPC_TCPTRSTREAM_H_ */
Generated on Tue Jul 12 2022 15:46:16 by
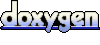