This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cOnchipFlashWriter.c
00001 #include "NyLPC_config.h" 00002 #if NyLPC_MCU==NyLPC_MCU_LPC4088 || NyLPC_MCU==NyLPC_MCU_LPC17xx 00003 #include "LPC17xx_IAP.h" 00004 #include "NyLPC_cOnchipFlashWriter.h" 00005 00006 00007 /** 00008 * 指定したアドレスが、オンチップフラッシュかどうか 00009 */ 00010 NyLPC_TBool NyLPC_cOnchipFlashWriter_isOnchipFlash(const void* i_addr) 00011 { 00012 unsigned long snum; 00013 return LPC17xx_IAP_addr2Sector(i_addr,&snum)==LPC17xx_IAP_CMD_SUCCESS; 00014 } 00015 00016 NyLPC_TUInt8 _work[256]; 00017 00018 00019 NyLPC_TBool NyLPC_cOnchipFlashWriter_write(const void* i_dest,const void* i_src,NyLPC_TUInt32 i_size) 00020 { 00021 NyLPC_TUInt32 size; 00022 const char* src; 00023 NyLPC_TUInt32 snum; 00024 const char* fblock_addr; 00025 const char* dest_addr; 00026 NyLPC_TUInt32 wsize; 00027 NyLPC_TUInt16 s_padding; 00028 NyLPC_TUInt16 free_size; 00029 if(i_size%4!=0){ 00030 NyLPC_OnErrorGoto(Error); 00031 } 00032 if(((NyLPC_TUInt32)i_dest)%4!=0){ 00033 NyLPC_OnErrorGoto(Error); 00034 } 00035 size=i_size; 00036 src=(const char*)i_src; 00037 dest_addr=(const char*)i_dest; 00038 for(;size>0;){ 00039 //開始位置の端数を調べる 00040 s_padding=((NyLPC_TUInt32)dest_addr)%256; 00041 //書き込みアドレス取得 00042 fblock_addr=dest_addr-s_padding; 00043 //書込み可能サイズを計算 00044 free_size=256-s_padding; 00045 //書込みサイズを決定 00046 wsize=(free_size>size)?size:free_size; 00047 //Flashから一時RAMへ前方パディングを読む 00048 if(s_padding>0){ 00049 memcpy(_work,fblock_addr,s_padding); 00050 } 00051 //書き込むデータを一時RAMへ書き込む 00052 memcpy(_work+s_padding,src,wsize); 00053 //後半 00054 if(256-(wsize+s_padding)>0){ 00055 memcpy(_work+s_padding+wsize,fblock_addr+(wsize+s_padding),256-(wsize+s_padding)); 00056 } 00057 00058 //Flashへ書込み 00059 //開始セクタ 00060 if(!LPC17xx_IAP_addr2Sector(fblock_addr,&snum)){ 00061 NyLPC_OnErrorGoto(Error); 00062 } 00063 //IAPのprepareコマンド 00064 00065 if(LPC17xx_IAP_CMD_SUCCESS!=LPC17xx_IAP_prepare(snum,snum)){ 00066 NyLPC_OnErrorGoto(Error); 00067 } 00068 //IAPのwriteコマンド 00069 if(LPC17xx_IAP_CMD_SUCCESS!=LPC17xx_IAP_copyRam2Flash(fblock_addr,_work,256)){ 00070 NyLPC_OnErrorGoto(Error); 00071 } 00072 dest_addr+=wsize; 00073 src+=wsize; 00074 size-=wsize; 00075 } 00076 return NyLPC_TBool_TRUE; 00077 Error: 00078 return NyLPC_TBool_FALSE; 00079 } 00080 00081 /** 00082 * セクタ+オフセット形式で、データを書き込みます。 00083 */ 00084 NyLPC_TBool NyLPC_cOnchipFlashWriter_writeSector(NyLPC_TUInt16 i_sector,NyLPC_TUInt32 i_offset,const void* i_src,NyLPC_TUInt32 i_size) 00085 { 00086 void* addr; 00087 if(!LPC17xx_IAP_sector2Addr(i_sector,&addr)){ 00088 return NyLPC_TBool_FALSE; 00089 } 00090 addr=(void*)((NyLPC_TUInt32)addr+i_offset); 00091 return NyLPC_cOnchipFlashWriter_write(addr,i_src,i_size); 00092 00093 } 00094 /** 00095 * FlashRomのセクタ番号Nにイレースを実行します。 00096 */ 00097 NyLPC_TBool NyLPC_cOnchipFlashWriter_elase(NyLPC_TUInt16 i_sector_s,NyLPC_TUInt16 i_sector_e) 00098 { 00099 if(LPC17xx_IAP_CMD_SUCCESS!=LPC17xx_IAP_prepare(i_sector_s,i_sector_e)){ 00100 return NyLPC_TBool_FALSE; 00101 } 00102 if(LPC17xx_IAP_CMD_SUCCESS!=LPC17xx_IAP_erase(i_sector_s,i_sector_e)){ 00103 return NyLPC_TBool_FALSE; 00104 } 00105 return NyLPC_TBool_TRUE; 00106 } 00107 00108 00109 00110 #ifdef TEST 00111 #include "stdio.h" 00112 unsigned long buf[128]={0x1,0x2,0x03,0x04,0x05}; 00113 #define MIMIC_CONFIG_ADDR ((long*)(0x00018000+1280)) 00114 void setup(void) 00115 { 00116 NyLPC_TcFlashWriter_t writer; 00117 NyLPC_cFlashWriter_initialize(&writer); 00118 unsigned long p; 00119 NyLPC_cFlashWriter_elase(&writer,29); 00120 // NyLPC_cFlashWriter_write(&writer,MIMIC_CONFIG_ADDR-8,buf,5*4); 00121 NyLPC_cFlashWriter_finalize(&writer); 00122 return; 00123 } 00124 void loop(void) 00125 { 00126 //Implementation 00127 //ここにメインタスクを書きます。 00128 for(;;){} 00129 } 00130 #endif 00131 #endif
Generated on Tue Jul 12 2022 15:46:16 by
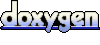