This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cNetConfig.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NYLPC_CNETCONFIG_H_ 00027 #define NYLPC_CNETCONFIG_H_ 00028 00029 #include "NyLPC_stdlib.h" 00030 #include "NyLPC_netif.h" 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif /* __cplusplus */ 00034 00035 #define NyLPC_TcNetConfig_HOSTNAME_LEN 32 00036 /** 00037 * クラス型を定義します。 00038 * NyLPC_cNetConfigクラスは、NyLPC_NetConfigの初期化データを保持します。 00039 * 初期化データのロード・セーブ機能を提供します。 00040 */ 00041 typedef struct NyLPC_TcNetConfig NyLPC_TcNetConfig_t; 00042 00043 00044 /** 00045 * NyLPC_TcIPv4Configクラスの継承クラスです。 00046 * IPv4設定にネットワーク設定項目を加えます。 00047 * 加えられる項目は全て[RECOMMEND]パラメータです。 00048 */ 00049 struct NyLPC_TcNetConfig 00050 { 00051 NyLPC_TcIPv4Config_t super; 00052 /** 00053 * ホスト名 00054 */ 00055 NyLPC_TChar hostname[NyLPC_TcNetConfig_HOSTNAME_LEN]; 00056 /** 00057 * tcp初期設定モードのフラグ値 00058 * NyLPC_TcNetConfig_IPV4_FLAG_Xの組み合わせ 00059 * bit 01:IP初期設定のモード. 0:Manual指定,1:DHCP指定,2:AutoIP指定,3:APIPA指定 00060 */ 00061 NyLPC_TUInt32 tcp_mode; 00062 struct{ 00063 /** 00064 * サービスのフラグセット。 00065 * NyLPC_TcNetConfig_SERVICE_FLAG_xの組み合わせ 00066 */ 00067 NyLPC_TUInt32 flags; 00068 NyLPC_TUInt16 http_port; 00069 NyLPC_TUInt16 padding; 00070 }services; 00071 00072 /** インタフェイス層の設定 */ 00073 }; 00074 #define NyLPC_TcNetConfig_IPV4_FLAG_MODE_MASK 0x00000003 00075 #define NyLPC_TcNetConfig_IPV4_FLAG_MODE_MANUAL 0x00000000 00076 #define NyLPC_TcNetConfig_IPV4_FLAG_MODE_DHCP 0x00000001 00077 #define NyLPC_TcNetConfig_IPV4_FLAG_MODE_AUTOIP 0x00000002 00078 #define NyLPC_TcNetConfig_IPV4_FLAG_MODE_APIPA (NyLPC_TcNetConfig_IPV4_FLAG_MODE_DHCP|NyLPC_TcNetConfig_IPV4_FLAG_MODE_AUTOIP) 00079 /*-------------------------------------------------- 00080 * NyLPC_TcNetConfig.services.flags 00081 --------------------------------------------------*/ 00082 00083 /** 00084 * MDNSサービスの有効・無効(いまのところビットパターンなので注意!) 00085 */ 00086 #define NyLPC_TcNetConfig_SERVICE_FLAG_MDNS 0x00000001 00087 #define NyLPC_TcNetConfig_SERVICE_FLAG_UPNP 0x00000002 00088 00089 00090 /** 00091 * フラッシュメモリから設定値を読み出して、インスタンスを初期化します。 00092 * @param i_is_factory_default 00093 * 出荷時設定を読み出すかを設定します。 00094 */ 00095 void NyLPC_cNetConfig_initialize(NyLPC_TcNetConfig_t* i_inst,NyLPC_TBool i_is_factory_default); 00096 00097 00098 /** 00099 * インスタンスを終期化します。 00100 */ 00101 #define NyLPC_cNetConfig_finalize(i_inst); 00102 00103 #define NyLPC_cNetConfig_setIpAddr(i_inst,ip1,ip2,ip3,ip4) NyLPC_TIPv4Addr_set(&((i_inst)->super.ip_addr),(ip1),(ip2),(ip3),(ip4)); 00104 00105 /** 00106 * Set IPv4 network mask value to instance. 00107 */ 00108 #define NyLPC_cNetConfig_setNetMask(i_inst,ip1,ip2,ip3,ip4) NyLPC_TIPv4Addr_set(&((i_inst)->super.netmask),(ip1),(ip2),(ip3),(ip4)); 00109 00110 /** 00111 * Set IPv4 default gateway address to instance. 00112 */ 00113 #define NyLPC_cNetConfig_setGateway(i_inst,ip1,ip2,ip3,ip4) NyLPC_TIPv4Addr_set(&((i_inst)->super.dr_addr),(ip1),(ip2),(ip3),(ip4)); 00114 00115 /** 00116 * Set ethernet mac address to instance. 00117 */ 00118 #define NyLPC_cNetConfig_setEmac(i_inst,a1,a2,a3,a4,a5,a6) NyLPC_TEthAddr_set(&((i_inst)->interface_setting.ethernet.eth_mac),(a1),(a2),(a3),(a4),(a5),(a6)); 00119 00120 00121 00122 #ifdef __cplusplus 00123 } 00124 #endif /* __cplusplus */ 00125 #endif /* NYLPC_CNETCONFIG_H_ */
Generated on Tue Jul 12 2022 15:46:16 by
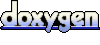