This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cModRomFiles.c
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #include "NyLPC_cModRomFiles_protected.h" 00027 #include "NyLPC_stdlib.h" 00028 #include "NyLPC_http.h" 00029 #include "../NyLPC_cHttpdUtils.h" 00030 #include "../NyLPC_cHttpdConnection_protected.h" 00031 00032 00033 /** 00034 * コンストラクタ。 00035 * @param i_ref_path 00036 * 16文字以内のルートパスを指定します。文字列は外部参照です。インスタンスをfinalizeするまで維持してください。 00037 * 文字列は/で開始され/で終了する必要があります。 00038 * path:= /({.,14}/)? 00039 * @param i_data 00040 * ROMFile構造体の配列の先頭アドレス 00041 */ 00042 void NyLPC_cModRomFiles_initialize(NyLPC_TcModRomFiles_t* i_inst,const NyLPC_TChar* i_ref_root_path,const struct NyLPC_TRomFileData* i_data,int i_num_of_data) 00043 { 00044 NyLPC_cModUrl_initialize(&(i_inst->super)); 00045 i_inst->_data=i_data; 00046 i_inst->_num_of_data=i_num_of_data; 00047 i_inst->_ref_root_path=i_ref_root_path; 00048 } 00049 void NyLPC_cModRomFiles_finalize(NyLPC_TcModRomFiles_t* i_inst) 00050 { 00051 NyLPC_cModUrl_finalize(&(i_inst->super)); 00052 } 00053 00054 /** 00055 * モジュールがコネクションをハンドリングできるかを返します。 00056 */ 00057 NyLPC_TBool NyLPC_cModRomFiles_canHandle(NyLPC_TcModRomFiles_t* i_inst,NyLPC_TcHttpdConnection_t* i_connection) 00058 { 00059 const NyLPC_TChar* in_url; 00060 const NyLPC_TChar* base_url=i_inst->_ref_root_path; 00061 //connectonの状態を確認 00062 if(!NyLPC_cHttpdConnection_getReqStatus(i_connection)==NyLPC_cHttpdConnection_ReqStatus_REQPARSE) 00063 { 00064 return NyLPC_TBool_FALSE; 00065 } 00066 in_url=NyLPC_cHttpdConnection_getUrlPrefix(i_connection); 00067 size_t base_url_len=strlen(base_url); 00068 //check '/'+base_url+'/' 00069 if(strlen(in_url)-2<base_url_len){ 00070 return NyLPC_TBool_FALSE; 00071 } 00072 if(in_url[0]!='/' || strncmp(in_url+1,base_url,base_url_len)!=0 || in_url[base_url_len+1]!='/'){ 00073 return NyLPC_TBool_FALSE; 00074 } 00075 return NyLPC_TBool_TRUE; 00076 } 00077 00078 00079 00080 /** 00081 * モジュールを実行します。 00082 */ 00083 NyLPC_TBool NyLPC_cModRomFiles_execute(NyLPC_TcModRomFiles_t* i_inst,NyLPC_TcHttpdConnection_t* i_connection) 00084 { 00085 int i; 00086 char url[32]; 00087 NyLPC_TUInt8 method_type; 00088 //URLサフィックスを取得(rootpath+'2'*2) 00089 if(!NyLPC_cModUrl_execute2(&(i_inst->super),i_connection,url,32,strlen(i_inst->_ref_root_path)+2,NyLPC_cModUrl_ParseMode_PATH_ONLY)) 00090 { 00091 //Response処理はモジュール内で実行済 00092 NyLPC_OnErrorGoto(Error1); 00093 } 00094 //GETかHEADに制限 00095 method_type=NyLPC_cHttpdConnection_getMethod(i_connection); 00096 if(method_type!=NyLPC_THttpMethodType_GET && method_type!=NyLPC_THttpMethodType_HEAD) 00097 { 00098 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,405); 00099 NyLPC_OnErrorGoto(Error1); 00100 } 00101 if(strlen(url)==0) 00102 { 00103 //PrefixがURLよりも短ければ403エラーで処理終了。 00104 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,403); 00105 NyLPC_OnErrorGoto(Error1); 00106 } 00107 //URL比較 00108 for(i=i_inst->_num_of_data-1;i>=0;i--) 00109 { 00110 if(strcmp(url,i_inst->_data[i].name)!=0){ 00111 continue; 00112 } 00113 //Request::ConnectionがClose設定,又はHTTP1.1では無い場合,CLOSE 00114 if(i_inst->super._header.connection==NyLPC_THttpMessgeHeader_Connection_CLOSE || i_inst->super._header.startline.req.version!=NyLPC_THttpVersion_11) 00115 { 00116 NyLPC_cHttpdConnection_setConnectionMode(i_connection,NyLPC_TcHttpdConnection_CONNECTION_MODE_CLOSE); 00117 } 00118 return NyLPC_cHttpdUtils_sendFixedContentBatch(i_connection,i_inst->_data[i].content_type,i_inst->_data[i].data,(i_inst->_data[i].size>0)?i_inst->_data[i].size:strlen(i_inst->_data[i].data)); 00119 } 00120 //404Error 00121 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,404); 00122 Error1: 00123 return NyLPC_TBool_FALSE; 00124 }
Generated on Tue Jul 12 2022 15:46:16 by
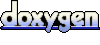