This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cModRemoteMcu.c
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #include "NyLPC_cModRemoteMcu.h" 00027 #include "NyLPC_stdlib.h" 00028 #include "NyLPC_http.h" 00029 #include "NyLPC_mimicVm.h" 00030 #include "../NyLPC_cHttpdConnection_protected.h" 00031 #include "../NyLPC_cHttpdUtils.h" 00032 #include "NyLPC_net.h" 00033 00034 #define MVM_VERSION "ModRemoteMcu/1.0;Json/1.0" 00035 #define SIZE_OF_IBUF 256 00036 struct TModMiMicRemoteMcuHeader 00037 { 00038 struct NyLPC_THttpBasicHeader super; 00039 NyLPC_TUInt8 _content_id; 00040 //解析用 00041 NyLPC_TUInt8 _qery_name_id; 00042 NyLPC_TUInt8 _astate; 00043 NyLPC_TInt16 _prefix_len; 00044 NyLPC_TcStr_t _tstr; 00045 NyLPC_TChar _tstr_buf[16]; 00046 struct NyLPC_TUInt32ArrayPtr _binarray; 00047 /** 文字列のパーサ*/ 00048 NyLPC_TcMiMicDbCompiler_t _binparser; 00049 NyLPC_TcMiMicTxtCompiler_t _txtcmp; 00050 union{ 00051 struct{ 00052 NyLPC_TUInt8 v;//バージョン 00053 NyLPC_TUInt8 o;//outputスタイル 00054 /** 00055 * il_bufはbcとdbの2パートのデータを格納します。 00056 * 先頭からbc_lenの長さのBCパートと、db_partからdb_lenの長さのデータです。 00057 */ 00058 struct{ 00059 /** MiMicVMインストラクションの蓄積用。前半にTXT,後半にDBを格納する。 */ 00060 NyLPC_TUInt32 bc_buf[SIZE_OF_IBUF]; 00061 /** MiMicVM入力ストリーム(MimicDB)の開始位置(bufの一部を指す) */ 00062 const NyLPC_TUInt32* db_part; 00063 /** MiMicTXTのワード長(1ワード32bit)*/ 00064 NyLPC_TUInt16 txt_len; 00065 /** MiMicDBのワード長(1ワード32bit)*/ 00066 NyLPC_TUInt16 db_len; 00067 }vm_instruction; 00068 }mvm; 00069 struct{ 00070 /** 00071 * 不明な名前の場合は、ここに名前をコピー 00072 */ 00073 NyLPC_TChar path[32]; 00074 }unknown; 00075 }content; 00076 }; 00077 00078 00079 static void mvm(NyLPC_TcHttpdConnection_t* i_connection,const struct TModMiMicRemoteMcuHeader* i_rqh); 00080 static void status(NyLPC_TcHttpdConnection_t* i_connection); 00081 00082 00083 00084 00085 #define ST_PARSE_PATH 1 00086 #define ST_PARSE_QUERY_NAME 2 00087 #define ST_PARSE_QUERY_VALUE 3 //Query読み出し中 00088 #define ST_PARSE_QUERY_VALUE_V 4 00089 #define ST_PARSE_QUERY_VALUE_O 5 00090 #define ST_PARSE_QUERY_VALUE_BC 6 00091 #define ST_PARSE_QUERY_VALUE_DB 7 00092 /** 00093 * コンテンツID定義(コンテンツ名に対応) 00094 */ 00095 #define CONTENT_ID_MVM 2 00096 #define CONTENT_ID_STATUS 3 00097 #define CONTENT_ID_UNKNOWN 0 00098 00099 00100 #define QNAME_ID_V 1 00101 #define QNAME_ID_O 2 00102 #define QNAME_ID_BC 3 00103 #define QNAME_ID_UNKNOWN 0 00104 00105 /** 00106 * TRemoteMcuRequest.content.mvm.oの値 00107 */ 00108 #define QVAL_O_UNKNOWN 0 //default 00109 #define QVAL_O_XML 1 00110 #define QVAL_O_JSON 2 00111 00112 #define QVAL_V_UNKNOWN 0 00113 #define QVAL_V_1 1 00114 00115 00116 static const struct NyLPC_TTextIdTbl url_tbl[]= 00117 { 00118 {"mvm.api",CONTENT_ID_MVM}, 00119 {"status.api",CONTENT_ID_STATUS}, 00120 {NULL,CONTENT_ID_UNKNOWN} 00121 }; 00122 00123 static const struct NyLPC_TTextIdTbl qname_id_table[]= 00124 { 00125 {"o",QNAME_ID_O}, 00126 {"bc",QNAME_ID_BC}, 00127 {"v",QNAME_ID_V}, 00128 {NULL,QNAME_ID_UNKNOWN} 00129 }; 00130 00131 00132 00133 static NyLPC_TBool urlHandler(NyLPC_TcHttpBasicHeaderParser_t* i_inst,NyLPC_TChar i_c,struct NyLPC_THttpBasicHeader* o_out) 00134 { 00135 NyLPC_TUInt16 ol; 00136 struct TModMiMicRemoteMcuHeader* out=(struct TModMiMicRemoteMcuHeader*)o_out; 00137 //読み飛ばし 00138 if(out->_prefix_len<0){ 00139 out->_prefix_len++; 00140 return NyLPC_TBool_TRUE;//読み飛ばし 00141 } 00142 if(out->_astate==ST_PARSE_PATH){ 00143 if(i_c!='\0' && i_c!='?'){ 00144 if(!NyLPC_cStr_put(&(out->_tstr),i_c)){ 00145 //ERROR 00146 NyLPC_OnErrorGoto(ERROR); 00147 } 00148 }else{ 00149 out->_content_id=NyLPC_TTextIdTbl_getMatchId(NyLPC_cStr_str(&(out->_tstr)),url_tbl); 00150 switch(out->_content_id) 00151 { 00152 case CONTENT_ID_MVM: 00153 out->content.mvm.vm_instruction.txt_len=0; 00154 out->content.mvm.vm_instruction.db_len=0; 00155 out->content.mvm.vm_instruction.db_part=NULL; 00156 NyLPC_TUInt32ArrayPtr_setBuf(&out->_binarray,out->content.mvm.vm_instruction.bc_buf,SIZE_OF_IBUF); 00157 out->content.mvm.o=QVAL_O_JSON; 00158 out->content.mvm.v=QVAL_V_UNKNOWN; 00159 break; 00160 default: 00161 break; 00162 } 00163 NyLPC_cStr_clear(&(out->_tstr)); 00164 out->_astate=ST_PARSE_QUERY_NAME;//クエリ名解析へ 00165 } 00166 return NyLPC_TBool_TRUE; 00167 } 00168 switch(out->_content_id) 00169 { 00170 case CONTENT_ID_MVM: 00171 switch(out->_astate){ 00172 case ST_PARSE_QUERY_NAME: 00173 if(i_c!='\0' && i_c!='&' && i_c!='='){ 00174 if(!NyLPC_cStr_put(&(out->_tstr),i_c)){ 00175 NyLPC_OnErrorGoto(ERROR); 00176 } 00177 }else{ 00178 //Query確定。 00179 out->_qery_name_id=NyLPC_TTextIdTbl_getMatchId(NyLPC_cStr_str(&(out->_tstr)),qname_id_table); 00180 NyLPC_cStr_clear(&(out->_tstr)); 00181 //クエリ値がある場合 00182 switch(out->_qery_name_id){ 00183 case QNAME_ID_O: 00184 out->_astate=ST_PARSE_QUERY_VALUE_O;//MIMICBCのDBパラメータパーサを借用。 00185 break; 00186 case QNAME_ID_V: 00187 out->_astate=ST_PARSE_QUERY_VALUE_V; 00188 break; 00189 case QNAME_ID_BC: 00190 out->_astate=ST_PARSE_QUERY_VALUE_BC; 00191 break; 00192 default: 00193 out->_astate=ST_PARSE_QUERY_VALUE; 00194 break; 00195 } 00196 } 00197 return NyLPC_TBool_TRUE; 00198 case ST_PARSE_QUERY_VALUE: 00199 //未知のクエリは無視 00200 if(i_c!='\0' && i_c!='&'){ 00201 }else{ 00202 //クエリ値解析完了 00203 out->_astate=ST_PARSE_QUERY_NAME; 00204 } 00205 return NyLPC_TBool_TRUE; 00206 case ST_PARSE_QUERY_VALUE_O: 00207 if(i_c!='\0' && i_c!='&'){ 00208 if(!NyLPC_cStr_put(&(out->_tstr),i_c)){ 00209 NyLPC_OnErrorGoto(ERROR); 00210 } 00211 }else{ 00212 if(NyLPC_cStr_isEqual(&(out->_tstr),"j")){ 00213 out->content.mvm.o=QVAL_O_JSON; 00214 }else if(NyLPC_cStr_isEqual(&(out->_tstr),"x")){ 00215 out->content.mvm.o=QVAL_O_XML; 00216 } 00217 out->_astate=ST_PARSE_QUERY_NAME; 00218 NyLPC_cStr_clear(&(out->_tstr)); 00219 } 00220 return NyLPC_TBool_TRUE; 00221 case ST_PARSE_QUERY_VALUE_V: 00222 if(i_c!='\0' && i_c!='&'){ 00223 if(!NyLPC_cStr_put(&(out->_tstr),i_c)){ 00224 NyLPC_OnErrorGoto(ERROR); 00225 } 00226 }else{ 00227 if(NyLPC_cStr_isEqual(&(out->_tstr),"1")){ 00228 out->content.mvm.v=QVAL_V_1; 00229 } 00230 out->_astate=ST_PARSE_QUERY_NAME; 00231 NyLPC_cStr_clear(&(out->_tstr)); 00232 } 00233 return NyLPC_TBool_TRUE; 00234 case ST_PARSE_QUERY_VALUE_BC: 00235 if(i_c!='\0' && i_c!='&'){ 00236 //コンパイル 00237 switch(NyLPC_cMiMicTxtCompiler_compileFragment2(&(out->_txtcmp),i_c,&(out->_binarray),&ol)) 00238 { 00239 case NyLPC_TcMiMicTxtCompiler_RET_OK: 00240 //命令確定。 00241 break; 00242 case NyLPC_TcMiMicTxtCompiler_RET_OK_END: 00243 //命令終端検出->モード切替 00244 out->content.mvm.vm_instruction.txt_len=SIZE_OF_IBUF-out->_binarray.len+ol; 00245 out->content.mvm.vm_instruction.db_part=out->content.mvm.vm_instruction.bc_buf+out->content.mvm.vm_instruction.txt_len; 00246 out->_astate=ST_PARSE_QUERY_VALUE_DB; 00247 break; 00248 case NyLPC_TcMiMicTxtCompiler_RET_CONTINUE: 00249 //何もしない 00250 break; 00251 case NyLPC_TcMiMicTxtCompiler_RET_NG: 00252 default: 00253 //ERROR 00254 NyLPC_OnErrorGoto(ERROR); 00255 } 00256 } 00257 return NyLPC_TBool_TRUE; 00258 // //フラグメント終端が検出できない終了はエラー 00259 // NyLPC_OnErrorGoto(ERROR); 00260 case ST_PARSE_QUERY_VALUE_DB: 00261 if(i_c!='\0' && i_c!='&'){ 00262 switch(NyLPC_cMiMicDbCompiler_compileFragment2(&(out->_binparser),i_c,out->_binarray.ptr)) 00263 { 00264 case NyLPC_TcMiMicDbCompiler_RET_CONTINUE: 00265 break; 00266 case NyLPC_TcMiMicDbCompiler_RET_OK: 00267 // 00268 if(!NyLPC_TUInt32ArrayPtr_seek(&(out->_binarray),1)){ 00269 //ERROR 00270 NyLPC_OnErrorGoto(ERROR); 00271 } 00272 break; 00273 case NyLPC_TcMiMicDbCompiler_RET_ERROR: 00274 default: 00275 //ERROR 00276 NyLPC_OnErrorGoto(ERROR); 00277 } 00278 }else{ 00279 //区切りのいいところで終わってる? 00280 if(NyLPC_cMiMicDbCompiler_hasFragment(&(out->_binparser))){ 00281 //ERROR 00282 NyLPC_OnErrorGoto(ERROR); 00283 } 00284 out->content.mvm.vm_instruction.db_len=((NyLPC_TUInt8*)(out->_binarray.ptr)-(NyLPC_TUInt8*)(out->content.mvm.vm_instruction.db_part))/sizeof(NyLPC_TUInt32); 00285 00286 //終端しているなら、次のクエリへ 00287 out->_astate=ST_PARSE_QUERY_NAME; 00288 } 00289 return NyLPC_TBool_TRUE; 00290 default: 00291 break; 00292 } 00293 NyLPC_OnErrorGoto(ERROR); 00294 default: 00295 NyLPC_OnErrorGoto(ERROR); 00296 } 00297 return NyLPC_TBool_TRUE; 00298 ERROR: 00299 return NyLPC_TBool_FALSE; 00300 } 00301 /** 00302 * デフォルトハンドラ 00303 */ 00304 static const struct NyLPC_TcHttpBasicHeaderParser_Handler handler= 00305 { 00306 NULL, 00307 urlHandler 00308 }; 00309 00310 00311 /** 00312 * コンストラクタ。 00313 */ 00314 void NyLPC_cModRemoteMcu_initialize(NyLPC_TcModRemoteMcu_t* i_inst,const NyLPC_TChar* i_ref_root_path) 00315 { 00316 NyLPC_cModRomFiles_initialize(&i_inst->super,i_ref_root_path,NULL,0); 00317 } 00318 void NyLPC_cModRemoteMcu_finalize(NyLPC_TcModRemoteMcu_t* i_inst) 00319 { 00320 NyLPC_cModRomFiles_finalize(&i_inst->super); 00321 } 00322 /** 00323 * モジュールがコネクションをハンドリングできるかを返します。 00324 */ 00325 NyLPC_TBool NyLPC_cModRemoteMcu_canHandle(NyLPC_TcModRemoteMcu_t* i_inst,NyLPC_TcHttpdConnection_t* i_connection) 00326 { 00327 return NyLPC_cModRomFiles_canHandle(&i_inst->super,i_connection); 00328 } 00329 00330 static struct TModMiMicRemoteMcuHeader single_header; 00331 00332 /** 00333 * モジュールを実行します。 00334 */ 00335 NyLPC_TBool NyLPC_cModRemoteMcu_execute(NyLPC_TcModRemoteMcu_t* i_inst,NyLPC_TcHttpdConnection_t* i_connection) 00336 { 00337 NyLPC_TcHttpBasicHeaderParser_t parser; 00338 NyLPC_TUInt8 method_type; 00339 //リクエストParse済へ遷移(この関数の後はModが責任を持ってリクエストを返却) 00340 NyLPC_cHttpdConnection_setReqStatusParsed(i_connection); 00341 00342 00343 //VM起動の為の排他ロック 00344 NyLPC_cHttpdConnection_lock(i_connection); 00345 00346 00347 //URL解析の準備 00348 single_header._prefix_len=-((NyLPC_TInt16)strlen(i_inst->super._ref_root_path)+2); 00349 single_header._astate=ST_PARSE_PATH; 00350 NyLPC_cStr_initialize(&single_header._tstr,single_header._tstr_buf,16); 00351 NyLPC_cMiMicDbCompiler_initialize(&single_header._binparser); 00352 NyLPC_cMiMicTxtCompiler_initialize(&single_header._txtcmp); 00353 00354 NyLPC_cHttpBasicHeaderParser_initialize(&parser,&handler); 00355 00356 00357 //プリフェッチしたデータを流す 00358 NyLPC_cHttpBasicHeaderParser_parseInit(&parser,&(single_header.super)); 00359 NyLPC_cHttpdConnection_pushPrefetchInfo(i_connection,&parser,&single_header.super); 00360 //後続をストリームから取り込む 00361 if(!NyLPC_cHttpBasicHeaderParser_parseStream(&parser,NyLPC_cHttpdConnection_refStream(i_connection),&(single_header.super))){ 00362 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,500); 00363 NyLPC_OnErrorGoto(Error1); 00364 } 00365 if(!NyLPC_cHttpBasicHeaderParser_parseFinish(&parser,&(single_header.super))){ 00366 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,500); 00367 NyLPC_OnErrorGoto(Error1); 00368 } 00369 //GETかHEADに制限 00370 method_type=NyLPC_cHttpdConnection_getMethod(i_connection); 00371 if(method_type!=NyLPC_THttpMethodType_GET && method_type!=NyLPC_THttpMethodType_HEAD) 00372 { 00373 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,405); 00374 NyLPC_OnErrorGoto(Error1); 00375 } 00376 //Request::ConnectionがClose設定,又はHTTP1.1では無い場合,CLOSE 00377 if(single_header.super.connection==NyLPC_THttpMessgeHeader_Connection_CLOSE || single_header.super.startline.req.version!=NyLPC_THttpVersion_11) 00378 { 00379 NyLPC_cHttpdConnection_setConnectionMode(i_connection,NyLPC_TcHttpdConnection_CONNECTION_MODE_CLOSE); 00380 } 00381 //CGIの実行 00382 switch(single_header._content_id) 00383 { 00384 case CONTENT_ID_MVM: 00385 mvm(i_connection,&single_header); 00386 break; 00387 case CONTENT_ID_STATUS: 00388 status(i_connection); 00389 break; 00390 default: 00391 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,400); 00392 NyLPC_OnErrorGoto(Error1); 00393 } 00394 NyLPC_cStr_finalize(&single_header._tstr); 00395 NyLPC_cMiMicDbCompiler_finalize(&single_header._binparser); 00396 NyLPC_cMiMicTxtCompiler_finalize(&single_header._txtcmp); 00397 NyLPC_cHttpBasicHeaderParser_finalize(&parser); 00398 //占有解除 00399 NyLPC_cHttpdConnection_unlock(i_connection); 00400 return NyLPC_TBool_TRUE; 00401 Error1: 00402 NyLPC_cStr_finalize(&single_header._tstr); 00403 NyLPC_cMiMicDbCompiler_finalize(&single_header._binparser); 00404 NyLPC_cMiMicTxtCompiler_finalize(&single_header._txtcmp); 00405 NyLPC_cHttpBasicHeaderParser_finalize(&parser); 00406 //VM排他ロックの解除 00407 NyLPC_cHttpdConnection_unlock(i_connection); 00408 return NyLPC_TBool_FALSE; 00409 } 00410 00411 00412 /** 00413 * イベントハンドラを継承 00414 */ 00415 struct TVmEventHandler 00416 { 00417 struct NyLPC_TcMiMicVM_TEvent super; 00418 const struct TModMiMicRemoteMcuHeader* req; 00419 NyLPC_TcHttpdConnection_t* connection; 00420 NyLPC_TUInt16 db_pos; 00421 /** ストリームへ出力したデータの数*/ 00422 NyLPC_TUInt16 st_len; 00423 /** Bodyを送信するかのフラグ*/ 00424 NyLPC_TBool is_send_body; 00425 }; 00426 00427 /** 00428 * ストリームハンドラ(put) 00429 */ 00430 static NyLPC_TBool mvmputs_json(struct NyLPC_TcMiMicVM_TEvent* i_eh,NyLPC_TUInt32 i_val) 00431 { 00432 struct TVmEventHandler* eh=(struct TVmEventHandler*)i_eh; 00433 if(eh->is_send_body){ 00434 if(eh->st_len>0){ 00435 eh->st_len++; 00436 return NyLPC_cHttpdConnection_sendResponseBodyF(eh->connection,",%u",i_val); 00437 }else{ 00438 eh->st_len++; 00439 return NyLPC_cHttpdConnection_sendResponseBodyF(eh->connection,"%u",i_val); 00440 } 00441 } 00442 return NyLPC_TBool_TRUE; 00443 } 00444 00445 /** 00446 * ストリームハンドラ(get) 00447 */ 00448 static NyLPC_TBool mvmgets(struct NyLPC_TcMiMicVM_TEvent* i_eh,NyLPC_TUInt32* o_val) 00449 { 00450 struct TVmEventHandler* eh=(struct TVmEventHandler*)i_eh; 00451 //読み出し済みDBサイズの確認 00452 if(eh->req->content.mvm.vm_instruction.db_len<=eh->db_pos){ 00453 //読めない 00454 return NyLPC_TBool_FALSE; 00455 } 00456 *o_val=eh->req->content.mvm.vm_instruction.db_part[eh->db_pos]; 00457 eh->db_pos++; 00458 return NyLPC_TBool_TRUE; 00459 } 00460 /** 00461 * ネイティブCALLハンドラ 00462 */ 00463 static NyLPC_TUInt32 nativeCall(struct NyLPC_TcMiMicVM_TEvent* i_evh,NyLPC_TUInt32 i_id,NyLPC_TcMiMicVM_t* i_vm) 00464 { 00465 (void)i_evh; 00466 // NyLPC_TNativeFunction f=getNativeFunctionById(i_id); 00467 // if(f==NULL){ 00468 // return NyLPC_cMiMicVM_RESULT_RUNTIME_NG_UNKNOWN_CALL; 00469 // } 00470 // return f(i_vm)?NyLPC_cMiMicVM_RESULT_OK:NyLPC_cMiMicVM_RESULT_RUNTIME_NG_CALL; 00471 return NyLPC_cMiMicVM_RESULT_RUNTIME_NG_CALL; 00472 } 00473 00474 00475 static void mvmsleep(struct NyLPC_TcMiMicVM_TEvent* i_eh,NyLPC_TUInt32 i_sleep_in_msec) 00476 { 00477 (void)i_eh; 00478 NyLPC_cThread_sleep(i_sleep_in_msec); 00479 } 00480 00481 /** 00482 * RemoteMCUのステータスを返す。基本的にjson 00483 * { 00484 * application:"[VERSION]" 00485 * } 00486 */ 00487 static void status(NyLPC_TcHttpdConnection_t* i_connection) 00488 { 00489 if(!NyLPC_cHttpdUtils_sendJsonHeader(i_connection)){ 00490 return; 00491 } 00492 //JSONを書く。 00493 if(NyLPC_cHttpdConnection_getMethod(i_connection)==NyLPC_THttpMethodType_GET){ 00494 NyLPC_cHttpdConnection_sendResponseBodyF(i_connection,"{\"application\":\""MVM_VERSION"\"}"); 00495 } 00496 return; 00497 } 00498 /** 00499 * MimicVMの起動と,ResponseJSONの起動 00500 * @return 00501 * 持続性接続を継続するかの真偽値 00502 */ 00503 static void mvm(NyLPC_TcHttpdConnection_t* i_connection,const struct TModMiMicRemoteMcuHeader* i_rqh) 00504 { 00505 struct TVmEventHandler he; 00506 NyLPC_TcMiMicVM_t vm; 00507 NyLPC_TUInt32 vmret; 00508 if(i_rqh->content.mvm.v!=QVAL_V_1 || i_rqh->content.mvm.o!=QVAL_O_JSON) 00509 { 00510 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,400); 00511 return; 00512 } 00513 00514 //Bodyを書く 00515 //ハンドラインスタンスの設定 00516 if(!NyLPC_cHttpdUtils_sendJsonHeader(i_connection)){ 00517 NyLPC_OnErrorGoto(Error1); 00518 } 00519 00520 he.super.get_stream=mvmgets; 00521 he.super.put_stream=mvmputs_json; 00522 he.super.native_call=nativeCall; 00523 he.super.sleep=mvmsleep; 00524 he.db_pos=0; 00525 he.st_len=0; 00526 he.connection=i_connection; 00527 he.req=i_rqh; 00528 he.is_send_body=(NyLPC_cHttpdConnection_getMethod(i_connection)==NyLPC_THttpMethodType_GET); 00529 00530 //起動VMの初期化 00531 NyLPC_cMiMicVM_initialize(&vm,(struct NyLPC_TcMiMicVM_TEvent*)&(he.super)); 00532 00533 //JSONを書く。 00534 if(he.is_send_body){ 00535 if(!NyLPC_cHttpdConnection_sendResponseBodyF(i_connection,"{\"version\":\""MVM_VERSION"\",\"stream\":[")){ 00536 NyLPC_OnErrorGoto(Error1); 00537 } 00538 } 00539 //VMの実行 00540 vmret=NyLPC_cMiMicVM_run(&(vm),i_rqh->content.mvm.vm_instruction.bc_buf,i_rqh->content.mvm.vm_instruction.txt_len); 00541 //only GET method 00542 if(he.is_send_body){ 00543 if(!NyLPC_cHttpdConnection_sendResponseBodyF(i_connection,"],\"result\":%u}",vmret)){ 00544 NyLPC_OnErrorGoto(Error1); 00545 } 00546 } 00547 NyLPC_cMiMicVM_finalize(&vm); 00548 return; 00549 Error1: 00550 NyLPC_cMiMicVM_finalize(&vm); 00551 return; 00552 } 00553
Generated on Tue Jul 12 2022 15:46:16 by
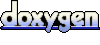