This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cMiMicVM_protected.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NYLPC_CMIMICVM_PROTECTED_H_ 00027 #define NYLPC_CMIMICVM_PROTECTED_H_ 00028 #include "NyLPC_cMiMicVM.h" 00029 00030 #ifdef __cplusplus 00031 extern "C" { 00032 #endif /* __cplusplus */ 00033 //---------------------------------------------------------------------- 00034 //MiMicOpCodeのタイプ値 00035 //---------------------------------------------------------------------- 00036 00037 #define NyLPC_TcMiMicVM_OP_TYPE_AND 0x11 00038 #define NyLPC_TcMiMicVM_OP_TYPE_OR 0x12 00039 #define NyLPC_TcMiMicVM_OP_TYPE_XOR 0x13 00040 #define NyLPC_TcMiMicVM_OP_TYPE_NOT 0x14 00041 00042 #define NyLPC_TcMiMicVM_OP_TYPE_SHL 0x21 00043 #define NyLPC_TcMiMicVM_OP_TYPE_SHR 0x22 00044 00045 #define NyLPC_TcMiMicVM_OP_TYPE_ADD 0x31 00046 #define NyLPC_TcMiMicVM_OP_TYPE_SUB 0x32 00047 #define NyLPC_TcMiMicVM_OP_TYPE_MUL 0x33 00048 00049 #define NyLPC_TcMiMicVM_OP_TYPE_MGET 0x41 00050 #define NyLPC_TcMiMicVM_OP_TYPE_MPUT 0x42 00051 00052 #define NyLPC_TcMiMicVM_OP_TYPE_SGET 0x51 00053 #define NyLPC_TcMiMicVM_OP_TYPE_SPUT 0x52 00054 00055 #define NyLPC_TcMiMicVM_OP_TYPE_NOP 0x61 00056 #define NyLPC_TcMiMicVM_OP_TYPE_EXIT 0x62 00057 #define NyLPC_TcMiMicVM_OP_TYPE_CALL 0x63 00058 00059 #define NyLPC_TcMiMicVM_OP_TYPE_LD 0x71 00060 00061 00062 #define NyLPC_TcMiMicVM_CP_TYPE_END 0x01 00063 /* 00064 #define NyLPC_TcMiMicVM_OP_TYPE_V_END 0x1001 00065 #define NyLPC_TcMiMicVM_OP_TYPE_V_DB8 0x1011 00066 #define NyLPC_TcMiMicVM_OP_TYPE_V_DB16 0x1012 00067 #define NyLPC_TcMiMicVM_OP_TYPE_V_DB32 0x1013 00068 */ 00069 00070 //---------------------------------------------------------------------- 00071 //MiMicOprandのタイプ値 00072 //---------------------------------------------------------------------- 00073 00074 #define NyLPC_TcMiMicVM_OPR_TYPE_NONE 0x01 00075 #define NyLPC_TcMiMicVM_OPR_TYPE_WM_WM 0x11 00076 #define NyLPC_TcMiMicVM_OPR_TYPE_WM_H08 0x12 00077 #define NyLPC_TcMiMicVM_OPR_TYPE_WM_H16 0x13 00078 #define NyLPC_TcMiMicVM_OPR_TYPE_WM_H32 0x14 00079 #define NyLPC_TcMiMicVM_OPR_TYPE_WM 0x21 00080 #define NyLPC_TcMiMicVM_OPR_TYPE_H08 0x22 00081 #define NyLPC_TcMiMicVM_OPR_TYPE_H16 0x23 00082 #define NyLPC_TcMiMicVM_OPR_TYPE_H32 0x24 00083 00084 00085 00086 00087 00088 /** 00089 * インストラクションセットのバイナリデータ型。 00090 * MiMicVMで実行するインストラクションをバッファから参照するために使います。 00091 * キャスト元のバッファは、32bitのunsigned intを想定しています。境界は32bit単位です。 00092 * 00093 * 構造体は、 00094 * 00095 */ 00096 union NyLPC_TcMiMicVM_TInstruction{ 00097 /** 00098 * オペコード情報のみを格納します 00099 */ 00100 struct{ 00101 NyLPC_TcMiMicVM_OP_TYPE opc; 00102 NyLPC_TUInt8 oprtype; 00103 }op; 00104 //32ビットコード 00105 00106 /** 00107 * WM[8],WM[8]オペランドの命令を格納します。 00108 */ 00109 struct{ 00110 NyLPC_TcMiMicVM_OP_TYPE opc; 00111 NyLPC_TUInt8 oprtype; 00112 NyLPC_TUInt8 wm1; 00113 NyLPC_TUInt8 wm2; 00114 }wmwm_32; 00115 struct{ 00116 NyLPC_TcMiMicVM_OP_TYPE opc; 00117 NyLPC_TUInt8 oprtype; 00118 NyLPC_TUInt8 wm; 00119 NyLPC_TUInt8 h8; 00120 }wmh08_32; 00121 struct{ 00122 NyLPC_TcMiMicVM_OP_TYPE opc; 00123 NyLPC_TUInt8 oprtype; 00124 NyLPC_TUInt8 wm; 00125 }wm_32; 00126 struct{ 00127 NyLPC_TcMiMicVM_OP_TYPE opc; 00128 NyLPC_TUInt8 oprtype; 00129 NyLPC_TUInt8 h8; 00130 }h8_32; 00131 struct{ 00132 NyLPC_TcMiMicVM_OP_TYPE opc; 00133 NyLPC_TUInt8 oprtype; 00134 NyLPC_TUInt8 h16; 00135 }h16_32; 00136 //64bit命令 00137 struct{ 00138 NyLPC_TcMiMicVM_OP_TYPE opc; 00139 NyLPC_TUInt8 oprtype; 00140 NyLPC_TUInt8 wm; 00141 NyLPC_TUInt8 _padding; 00142 NyLPC_TUInt16 h16; 00143 }wmh16_64; 00144 struct{ 00145 NyLPC_TcMiMicVM_OP_TYPE opc; 00146 NyLPC_TUInt8 oprtype; 00147 NyLPC_TUInt8 wm; 00148 NyLPC_TUInt8 _padding; 00149 NyLPC_TUInt32 h32; 00150 }wmh32_64; 00151 struct{ 00152 NyLPC_TcMiMicVM_OP_TYPE opc; 00153 NyLPC_TUInt8 oprtype; 00154 NyLPC_TUInt8 _padding[2]; 00155 NyLPC_TUInt32 h32; 00156 }h32_64; 00157 }; 00158 00159 #ifdef __cplusplus 00160 } 00161 #endif /* __cplusplus */ 00162 00163 #endif /* NYLPC_CMIMICVM_PROTECTED_H_ */
Generated on Tue Jul 12 2022 15:46:16 by
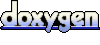