This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cMiMicTxtCompiler.c
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #include <ctype.h> 00027 #include <stdlib.h> 00028 #include "NyLPC_cMiMicTxtCompiler.h" 00029 #include "NyLPC_cMiMicVM_protected.h" 00030 #include "NyLPC_mimicvm_utils_protected.h" 00031 00032 00033 struct TInstructionDef 00034 { 00035 /** MiMicBCのオペコード */ 00036 const char* bcopcode; 00037 /** インストラクションID */ 00038 NyLPC_TcMiMicVM_OP_TYPE opid; 00039 /** オペコードのタイプ */ 00040 NyLPC_TcMiMicVM_OPR_TYPE optype; 00041 }; 00042 static NyLPC_TBool bc2opc(const NyLPC_TChar* i_char,NyLPC_TcMiMicVM_OP_TYPE* o_opc,NyLPC_TcMiMicVM_OPR_TYPE* o_opt); 00043 static NyLPC_TBool bc2ctrlc(const NyLPC_TChar* i_char,NyLPC_TcMiMicVM_OP_TYPE* o_opc,NyLPC_TcMiMicVM_OPR_TYPE* o_opt); 00044 static NyLPC_TBool txt2UInt(const NyLPC_TChar* i_txt,NyLPC_TUInt8 i_num,void* out); 00045 static NyLPC_TBool txt2WMId(const NyLPC_TChar* i_txt,NyLPC_TUInt8* out); 00046 static void NyLPC_TcMiMicVM_OPR_TYPE_getOpInfo(NyLPC_TcMiMicVM_OPR_TYPE i_type,NyLPC_TUInt8* oprbc_len,NyLPC_TUInt8* o_istlen); 00047 00048 00049 void NyLPC_cMiMicTxtCompiler_initialize(NyLPC_TcMiMicTxtCompiler_t* i_inst) 00050 { 00051 i_inst->out_buf=NULL; 00052 i_inst->st=NyLPC_TcMiMicTxtCompiler_ST_OPC; 00053 i_inst->tmp_len=0; 00054 } 00055 00056 00057 00058 #define NyLPC_TcMiMicTxtCompiler_ST_OPC 0x01 //OPをパース中 00059 #define NyLPC_TcMiMicTxtCompiler_ST_OPR 0x02 //オペランドを解析中 00060 #define NyLPC_TcMiMicTxtCompiler_ST_CTR 0x03 //制御パラメータを解析中 00061 #define NyLPC_TcMiMicTxtCompiler_ST_OK 0x04 //パースを完了した。(.EMD検出) 00062 #define NyLPC_TcMiMicTxtCompiler_ST_NG 0xff //パースでエラーが発生ウェーイ 00063 00064 00065 00066 /** 00067 * バイトコードフラグメントから1命令をコンパイルして、i_binへ出力します。 00068 * バイトコードは、バイナリ値に変換されます。 00069 * バイナリ値は、MiMicVMの実行形式です。 00070 * @param i_bin 00071 * コンパイルしたBCを格納する配列を指定します。 00072 * 関数が成功した場合、配列のポインターは追加したBCの数だけ進行します。 00073 * @return 00074 * 実行結果を返します。NyLPC_TcMiMicTxtCompiler_RET_OKの場合に、i_binへo_bin_lenの長さのインストラクションを出力します。 00075 * 00076 */ 00077 NyLPC_TcMiMicTxtCompiler_RET NyLPC_cMiMicTxtCompiler_compileFragment(NyLPC_TcMiMicTxtCompiler_t* i_inst,const struct NyLPC_TCharArrayPtr* i_bc,struct NyLPC_TUInt32ArrayPtr* i_bin,NyLPC_TUInt16* o_bin_len,NyLPC_TUInt16* o_parsed_bc) 00078 { 00079 union NyLPC_TcMiMicVM_TInstruction* wptr; 00080 int i; 00081 for(i=0;i<i_bc->len;i++) 00082 { 00083 switch(i_inst->st){ 00084 case NyLPC_TcMiMicTxtCompiler_ST_OPC: 00085 if(i_inst->tmp_len>2){ 00086 NyLPC_OnErrorGoto(ERROR); 00087 } 00088 i_inst->tmp[i_inst->tmp_len]=*(i_bc->ptr+i); 00089 i_inst->tmp_len++; 00090 if(i_inst->tmp_len==2){ 00091 //[A-Z]{2}がそろった。命令コードか制御命令か判定 00092 if(bc2opc(i_inst->tmp,&(i_inst->_current_opc),&(i_inst->_current_oprtype))){ 00093 //命令コードならインストラクションの情報をもらってくる。 00094 NyLPC_TcMiMicVM_OPR_TYPE_getOpInfo(i_inst->_current_oprtype,&i_inst->_oprbc_len,&i_inst->_inst_len); 00095 //オペランドが無ければ、命令確定。 00096 if(i_inst->_oprbc_len<=0){ 00097 //書込みポインタ保管 00098 wptr=(union NyLPC_TcMiMicVM_TInstruction*)i_bin->ptr; 00099 //バッファのシーク 00100 if(!NyLPC_TUInt32ArrayPtr_seek(i_bin,1)){ 00101 NyLPC_OnErrorGoto(ERROR); 00102 } 00103 //インストラクションの出力と処理したBC長の計算 00104 wptr->op.opc=i_inst->_current_opc; 00105 wptr->op.oprtype=i_inst->_current_oprtype; 00106 *o_parsed_bc=i+1; //パースしたBCの長さ 00107 *o_bin_len=i_inst->_inst_len; 00108 //解析バッファの長さをリセットして、次のBCブロックのパース準備 00109 i_inst->tmp_len=0; 00110 return NyLPC_TcMiMicTxtCompiler_RET_OK;//命令確定。 00111 } 00112 //オペランドがあるなら、パース対象を変更。 00113 i_inst->tmp_len=0; 00114 i_inst->st=NyLPC_TcMiMicTxtCompiler_ST_OPR; 00115 }else if(bc2ctrlc(i_inst->tmp,&(i_inst->_current_opc),&(i_inst->_current_oprtype))){ 00116 //命令コードならインストラクションの情報をもらってくる。 00117 NyLPC_TcMiMicVM_OPR_TYPE_getOpInfo(i_inst->_current_oprtype,&i_inst->_oprbc_len,&i_inst->_inst_len); 00118 //制御コードの解析 00119 if(i_inst->_oprbc_len>0){ 00120 //パラメータのある制御命令未定義だからエラー。 00121 NyLPC_OnErrorGoto(ERROR); 00122 } 00123 //END制御命令? 00124 if(i_inst->_current_opc==NyLPC_TcMiMicVM_CP_TYPE_END){ 00125 *o_bin_len=0; 00126 *o_parsed_bc=i+1; //パースしたBCの長さ 00127 i_inst->tmp_len=0; 00128 return NyLPC_TcMiMicTxtCompiler_RET_OK_END;//命令確定。(パース完了) 00129 } 00130 //END制御命令以外ならエラー 00131 NyLPC_OnErrorGoto(ERROR); 00132 }else{ 00133 //不明な命令 00134 NyLPC_OnErrorGoto(ERROR); 00135 } 00136 } 00137 break; 00138 case NyLPC_TcMiMicTxtCompiler_ST_CTR: 00139 NyLPC_OnErrorGoto(ERROR); 00140 case NyLPC_TcMiMicTxtCompiler_ST_OPR: 00141 //オペランド蓄積. 00142 i_inst->tmp[i_inst->tmp_len]=*(i_bc->ptr+i); 00143 i_inst->tmp_len++; 00144 //オペランド長さになるまで値を追記 00145 if(i_inst->_oprbc_len==i_inst->tmp_len){ 00146 //書込みポインタ保管 00147 wptr=(union NyLPC_TcMiMicVM_TInstruction*)i_bin->ptr; 00148 //シーク 00149 if(!NyLPC_TUInt32ArrayPtr_seek(i_bin,i_inst->_inst_len)){ 00150 NyLPC_OnErrorGoto(ERROR); 00151 } 00152 wptr->op.opc=i_inst->_current_opc; 00153 wptr->op.oprtype=i_inst->_current_oprtype; 00154 //オペランドの変換処理 00155 switch(i_inst->_current_oprtype){ 00156 case NyLPC_TcMiMicVM_OPR_TYPE_WM_WM: 00157 if(!( txt2WMId(i_inst->tmp,&(wptr->wmwm_32.wm1)) && 00158 txt2WMId(i_inst->tmp+2,&(wptr->wmwm_32.wm2)))) 00159 { 00160 NyLPC_OnErrorGoto(ERROR); 00161 } 00162 break; 00163 case NyLPC_TcMiMicVM_OPR_TYPE_WM_H08: 00164 if(!( txt2WMId(i_inst->tmp,&(wptr->wmh08_32.wm)) && 00165 txt2UInt(i_inst->tmp+2,2,&(wptr->wmh08_32.h8)))) 00166 { 00167 NyLPC_OnErrorGoto(ERROR); 00168 } 00169 break; 00170 case NyLPC_TcMiMicVM_OPR_TYPE_WM_H32: 00171 if(!( txt2WMId(i_inst->tmp,&(wptr->wmh32_64.wm)) && 00172 txt2UInt(i_inst->tmp+2,8,&(wptr->wmh32_64.h32)))){ 00173 NyLPC_OnErrorGoto(ERROR); 00174 } 00175 break; 00176 case NyLPC_TcMiMicVM_OPR_TYPE_WM: 00177 if(!txt2WMId(i_inst->tmp,&(wptr->wm_32.wm))){ 00178 NyLPC_OnErrorGoto(ERROR); 00179 } 00180 break; 00181 case NyLPC_TcMiMicVM_OPR_TYPE_H32: 00182 if(!txt2UInt(i_inst->tmp,8,&(wptr->h32_64.h32))){ 00183 NyLPC_OnErrorGoto(ERROR); 00184 } 00185 break; 00186 case NyLPC_TcMiMicVM_OPR_TYPE_H08: 00187 if(!txt2UInt(i_inst->tmp,2,&(wptr->h8_32.h8))) 00188 { 00189 NyLPC_OnErrorGoto(ERROR); 00190 } 00191 break; 00192 default: 00193 NyLPC_OnErrorGoto(ERROR); 00194 } 00195 //OPR解析成功。パースしたブロックサイズの計算。 00196 *o_parsed_bc=i+1; //パースしたBCの長さ 00197 *o_bin_len=i_inst->_inst_len; 00198 i_inst->tmp_len=0; 00199 i_inst->st=NyLPC_TcMiMicTxtCompiler_ST_OPC; 00200 return NyLPC_TcMiMicTxtCompiler_RET_OK; 00201 } 00202 break; 00203 default: 00204 NyLPC_OnErrorGoto(ERROR); 00205 } 00206 } 00207 *o_bin_len=0; 00208 *o_parsed_bc=i_bc->len; 00209 return NyLPC_TcMiMicTxtCompiler_RET_CONTINUE; 00210 ERROR: 00211 i_inst->st=NyLPC_TcMiMicTxtCompiler_ST_NG; 00212 return NyLPC_TcMiMicTxtCompiler_RET_NG; 00213 } 00214 00215 00216 00217 /** 00218 * フラグメント入力のMiMicBCをコンパイルします。 00219 * この関数は、1文字のフラグメントMiMicBCをコンパイラに入力します。 00220 * @param i_bin 00221 * コンパイルしたBCを格納する配列を指定します。 00222 * 関数が成功した場合、配列のポインターは追加したBCの数だけ進行します。 00223 * @return 00224 * 実行結果を返します。TRUEのときは、ステータスをチェックしてください。 00225 * 00226 */ 00227 NyLPC_TcMiMicTxtCompiler_RET NyLPC_cMiMicTxtCompiler_compileFragment2(NyLPC_TcMiMicTxtCompiler_t* i_inst,NyLPC_TChar i_bc,struct NyLPC_TUInt32ArrayPtr* i_bin,NyLPC_TUInt16* o_bin_len) 00228 { 00229 struct NyLPC_TCharArrayPtr bc; 00230 NyLPC_TUInt16 bc_len; 00231 bc.ptr=&i_bc; 00232 bc.len=1; 00233 return NyLPC_cMiMicTxtCompiler_compileFragment(i_inst,&bc,i_bin,o_bin_len,&bc_len); 00234 } 00235 00236 00237 00238 /** 00239 * 2バイトのバイトコードを、制御コードに変換します。 00240 */ 00241 static NyLPC_TBool bc2ctrlc(const NyLPC_TChar* i_char,NyLPC_TcMiMicVM_OP_TYPE* o_opc,NyLPC_TcMiMicVM_OPR_TYPE* o_opt) 00242 { 00243 //バイトコード変換の為のテーブル 00244 const struct TInstructionDef _bc_type_tbl[]= 00245 { 00246 //制御命令 00247 {".E",NyLPC_TcMiMicVM_CP_TYPE_END,NyLPC_TcMiMicVM_OPR_TYPE_NONE}, 00248 {NULL} 00249 }; 00250 int i; 00251 for(i=0;_bc_type_tbl[i].bcopcode!=NULL;i++){ 00252 //2バイト一致? 00253 if((*i_char==_bc_type_tbl[i].bcopcode[0])&&(*(i_char+1)==_bc_type_tbl[i].bcopcode[1])){ 00254 *o_opc=_bc_type_tbl[i].opid; 00255 *o_opt=_bc_type_tbl[i].optype; 00256 return NyLPC_TBool_TRUE; 00257 } 00258 } 00259 return NyLPC_TBool_FALSE; 00260 } 00261 00262 00263 /** 00264 * 2バイトのバイトコードを、命令定義に変換します。 00265 */ 00266 static NyLPC_TBool bc2opc(const NyLPC_TChar* i_char,NyLPC_TcMiMicVM_OP_TYPE* o_opc,NyLPC_TcMiMicVM_OPR_TYPE* o_opt) 00267 { 00268 //バイトコード変換の為のテーブル 00269 const struct TInstructionDef _bc_type_tbl[]= 00270 { 00271 {"AA",NyLPC_TcMiMicVM_OP_TYPE_AND,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00272 {"AB",NyLPC_TcMiMicVM_OP_TYPE_AND,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00273 {"AE",NyLPC_TcMiMicVM_OP_TYPE_OR,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00274 {"AF",NyLPC_TcMiMicVM_OP_TYPE_OR,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00275 {"AI",NyLPC_TcMiMicVM_OP_TYPE_XOR,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00276 {"AJ",NyLPC_TcMiMicVM_OP_TYPE_XOR,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00277 {"AM",NyLPC_TcMiMicVM_OP_TYPE_NOT,NyLPC_TcMiMicVM_OPR_TYPE_WM}, 00278 00279 {"BA",NyLPC_TcMiMicVM_OP_TYPE_SHL,NyLPC_TcMiMicVM_OPR_TYPE_WM_H08}, 00280 {"BB",NyLPC_TcMiMicVM_OP_TYPE_SHL,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00281 {"BE",NyLPC_TcMiMicVM_OP_TYPE_SHR,NyLPC_TcMiMicVM_OPR_TYPE_WM_H08}, 00282 {"BF",NyLPC_TcMiMicVM_OP_TYPE_SHR,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00283 00284 {"CA",NyLPC_TcMiMicVM_OP_TYPE_ADD,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00285 {"CB",NyLPC_TcMiMicVM_OP_TYPE_ADD,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00286 {"CE",NyLPC_TcMiMicVM_OP_TYPE_SUB,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00287 {"CF",NyLPC_TcMiMicVM_OP_TYPE_SUB,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00288 {"CI",NyLPC_TcMiMicVM_OP_TYPE_MUL,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00289 {"CJ",NyLPC_TcMiMicVM_OP_TYPE_MUL,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00290 00291 {"DA",NyLPC_TcMiMicVM_OP_TYPE_MGET,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00292 {"DB",NyLPC_TcMiMicVM_OP_TYPE_MGET,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00293 {"DE",NyLPC_TcMiMicVM_OP_TYPE_MPUT,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00294 {"DF",NyLPC_TcMiMicVM_OP_TYPE_MPUT,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00295 00296 {"EA",NyLPC_TcMiMicVM_OP_TYPE_SGET,NyLPC_TcMiMicVM_OPR_TYPE_WM}, 00297 {"EE",NyLPC_TcMiMicVM_OP_TYPE_SPUT,NyLPC_TcMiMicVM_OPR_TYPE_WM}, 00298 {"EF",NyLPC_TcMiMicVM_OP_TYPE_SPUT,NyLPC_TcMiMicVM_OPR_TYPE_H32}, 00299 00300 {"FA",NyLPC_TcMiMicVM_OP_TYPE_LD,NyLPC_TcMiMicVM_OPR_TYPE_WM_WM}, 00301 {"FB",NyLPC_TcMiMicVM_OP_TYPE_LD,NyLPC_TcMiMicVM_OPR_TYPE_WM_H32}, 00302 00303 {"ZA",NyLPC_TcMiMicVM_OP_TYPE_NOP,NyLPC_TcMiMicVM_OPR_TYPE_NONE}, 00304 {"ZB",NyLPC_TcMiMicVM_OP_TYPE_NOP,NyLPC_TcMiMicVM_OPR_TYPE_H08}, 00305 00306 {"ZE",NyLPC_TcMiMicVM_OP_TYPE_CALL,NyLPC_TcMiMicVM_OPR_TYPE_WM}, 00307 {"ZF",NyLPC_TcMiMicVM_OP_TYPE_CALL,NyLPC_TcMiMicVM_OPR_TYPE_H32}, 00308 00309 00310 {"ZZ",NyLPC_TcMiMicVM_OP_TYPE_EXIT,NyLPC_TcMiMicVM_OPR_TYPE_NONE}, 00311 {NULL} 00312 }; 00313 int i; 00314 //ここ早くできますよね。 00315 for(i=0;_bc_type_tbl[i].bcopcode!=NULL;i++){ 00316 //2バイト一致? 00317 if((*i_char==_bc_type_tbl[i].bcopcode[0])&&(*(i_char+1)==_bc_type_tbl[i].bcopcode[1])){ 00318 *o_opc=_bc_type_tbl[i].opid; 00319 *o_opt=_bc_type_tbl[i].optype; 00320 return NyLPC_TBool_TRUE; 00321 } 00322 } 00323 return NyLPC_TBool_FALSE; 00324 } 00325 00326 00327 /** 00328 * 長さi_numの16進数文字列を数値に変換する。アルファベットは小文字であること。 00329 * @param i_num 00330 * 変換する文字数 00331 * @param out 00332 * 00333 */ 00334 static NyLPC_TBool txt2UInt(const NyLPC_TChar* i_txt,NyLPC_TUInt8 i_num,void* out) 00335 { 00336 NyLPC_TUInt32 ret=0; 00337 NyLPC_TChar c; 00338 int i; 00339 00340 for(i=0;i<i_num;i++){ 00341 c=(*(i_txt+i)); 00342 if('f'>=c && c>='a'){ 00343 c=c-(NyLPC_TUInt8)'a'+10; 00344 }else if('9'>=c && c>='0'){ 00345 c-=(NyLPC_TUInt8)'0'; 00346 }else{ 00347 return NyLPC_TBool_FALSE; 00348 } 00349 ret=(ret<<4)|c; 00350 } 00351 //2,4,8だけ。 00352 switch(i_num){ 00353 case 2: 00354 *((NyLPC_TUInt8*)out)=(NyLPC_TUInt8)ret; 00355 break; 00356 case 4: 00357 *((NyLPC_TUInt16*)out)=(NyLPC_TUInt16)ret; 00358 break; 00359 case 8: 00360 *((NyLPC_TUInt32*)out)=(NyLPC_TUInt32)ret; 00361 break; 00362 default: 00363 return NyLPC_TBool_FALSE; 00364 } 00365 return NyLPC_TBool_TRUE; 00366 } 00367 /** 00368 * テキストデータをWMIDに変換する。WMIDは、VMの使用の影響を受ける。 00369 */ 00370 static NyLPC_TBool txt2WMId(const NyLPC_TChar* i_txt,NyLPC_TUInt8* out) 00371 { 00372 if(txt2UInt(i_txt,2,out)){ 00373 if(*out<=NyLPC_TcMiMicVM_NUMBER_OF_WM){ 00374 return NyLPC_TBool_TRUE; 00375 } 00376 } 00377 return NyLPC_TBool_FALSE; 00378 } 00379 /** 00380 * オペランドタイプからオペランドのBC長と、インストラクションサイズを計算 00381 */ 00382 static void NyLPC_TcMiMicVM_OPR_TYPE_getOpInfo(NyLPC_TcMiMicVM_OPR_TYPE i_type,NyLPC_TUInt8* oprbc_len,NyLPC_TUInt8* o_istlen) 00383 { 00384 const struct{ 00385 NyLPC_TcMiMicVM_OPR_TYPE t; 00386 NyLPC_TUInt8 oprbc_len; 00387 NyLPC_TUInt8 ist_len; 00388 }_tbl[]={ 00389 {NyLPC_TcMiMicVM_OPR_TYPE_NONE, 0, 1}, 00390 {NyLPC_TcMiMicVM_OPR_TYPE_WM_WM, (1+1)*2, 1}, 00391 {NyLPC_TcMiMicVM_OPR_TYPE_WM_H08, (1+1)*2, 1}, 00392 {NyLPC_TcMiMicVM_OPR_TYPE_WM_H16, (1+2)*2, 2}, 00393 {NyLPC_TcMiMicVM_OPR_TYPE_WM_H32, (1+4)*2, 2}, 00394 {NyLPC_TcMiMicVM_OPR_TYPE_WM, (1)*2, 1}, 00395 {NyLPC_TcMiMicVM_OPR_TYPE_H08, (1)*2, 1}, 00396 {NyLPC_TcMiMicVM_OPR_TYPE_H16, (2)*2, 1}, 00397 {NyLPC_TcMiMicVM_OPR_TYPE_H32, (4)*2, 2}, 00398 {0,0,0} 00399 }; 00400 int i; 00401 for(i=0;_tbl[i].t!=0;i++){ 00402 if(_tbl[i].t==i_type){ 00403 *oprbc_len=_tbl[i].oprbc_len; 00404 *o_istlen=_tbl[i].ist_len; 00405 return; 00406 } 00407 } 00408 NyLPC_Abort(); 00409 return; 00410 } 00411 00412 #define TEST 00413 #ifndef TEST 00414 void main(void) 00415 { 00416 struct NyLPC_TCharArrayPtr bc; 00417 const char* BC="AA0102AB0100000001AE0203AF0200000003AI0304AJ0300000004AM07BA0505BE0607CA0304CB0300000005CE0304CF0300000005CI0304CJ0300000005DA0400000000DE0400000000EA04EE04EF00000000ZAZZ.E"; 00418 NyLPC_TcMiMicTxtCompiler_t inst; 00419 struct NyLPC_TUInt32ArrayPtr bin; 00420 00421 NyLPC_TUInt16 l,bl; 00422 NyLPC_TUInt32 obuf[1024]; 00423 NyLPC_cMiMicBcCompiler_initialize(&inst); 00424 bc.ptr=(char* )BC; 00425 bc.len=strlen(BC); 00426 bin.ptr=obuf; 00427 bin.len=5; 00428 00429 for(;;){ 00430 00431 switch(NyLPC_cMiMicBcCompiler_compileFragment(&inst,&bc,&bin,&bl,&l)) 00432 { 00433 case NyLPC_TcMiMicTxtCompiler_RET_OK: 00434 //命令確定。 00435 NyLPC_TUInt32ArrayPtr_seek(&bin,bl); 00436 NyLPC_TCharArrayPtr_seek(&bc,l); 00437 break; 00438 case NyLPC_TcMiMicTxtCompiler_RET_OK_END: 00439 //命令終端 00440 printf("OK"); 00441 break; 00442 case NyLPC_TcMiMicTxtCompiler_RET_CONTINUE: 00443 //蓄積中。 00444 NyLPC_TCharArrayPtr_seek(&bc,l); 00445 break; 00446 case NyLPC_TcMiMicTxtCompiler_RET_NG: 00447 printf("エラー"); 00448 return; 00449 default: 00450 break; 00451 } 00452 } 00453 } 00454 #endif
Generated on Tue Jul 12 2022 15:46:16 by
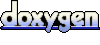