This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cJsonRpcParser.h
00001 #ifndef NYLPC_CJSONRPCPARSER_H_ 00002 #define NYLPC_CJSONRPCPARSER_H_ 00003 #ifdef __cplusplus 00004 extern "C" { 00005 #endif 00006 00007 #include "NyLPC_stdlib.h" 00008 00009 00010 #define NyLPC_TJsonRpcErrorCode_PARSE_ERROR (-32700) 00011 #define NyLPC_TJsonRpcErrorCode_INVALID_REQUEST (-32600) 00012 #define NyLPC_TJsonRpcErrorCode_METHOD_NOT_FOUND (-32601) 00013 #define NyLPC_TJsonRpcErrorCode_INVALID_PARAMS (-32602) 00014 #define NyLPC_TJsonRpcErrorCode_INTERNAL_ERROR (-32603) 00015 #define NyLPC_TJsonRpcErrorCode_SERVER_ERROR_BASE (-32000) 00016 00017 00018 /** 型定義*/ 00019 union NyLPC_TJsonRpcParserResult; 00020 00021 /** 00022 * RPC関数ハンドラ型です。 00023 * @param 00024 */ 00025 typedef NyLPC_TBool (*NyLPC_TJsonRpcHandler)(const union NyLPC_TJsonRpcParserResult* i_rpc,void* i_param); 00026 00027 /** 00028 * JSON RPC定義テーブルの一要素。 00029 * メソッド名とパラメータパターンのセットを定義します。 00030 * この構造体は配列としてNyLPC_TJsonRpcFunctionTableから参照されます。 00031 */ 00032 struct NyLPC_TJsonRpcMethodDef 00033 { 00034 /** 00035 * 関数名 00036 */ 00037 const char* name; 00038 /** 00039 * パラメータパターン 00040 * <ul> 00041 * <li>s - 文字列</li> 00042 * <li>i - signed int32</li> 00043 * <li>u - unsigned int32</li> 00044 * <li>b - unsigned int8</li> 00045 * <li>B - BSTR (string ByteArray)</li> 00046 * </ul> 00047 */ 00048 const char* param_patt; 00049 /** 00050 * 外部のDispatch関数で使われるRPC関数ハンドラ。 00051 */ 00052 NyLPC_TJsonRpcHandler handler; 00053 }; 00054 00055 00056 /** 00057 * JsonRPCクラスの定義テーブル。 00058 * JsonRPCは、 [namespace]::[] 00059 */ 00060 struct NyLPC_TJsonRpcClassDef 00061 { 00062 const char* names_pace; 00063 const char* class_name; 00064 const struct NyLPC_TJsonRpcMethodDef* functions; 00065 }; 00066 00067 00068 00069 00070 00071 #define NyLPC_TJsonRpcParserResult_TYPE_UNKNOWN 0 00072 #define NyLPC_TJsonRpcParserResult_TYPE_RESULT 1 00073 #define NyLPC_TJsonRpcParserResult_TYPE_METHOD 2 00074 00075 /** NyLPC_TJsonRpcParserResultが格納できる引数の最大数。パラメータの最大数に等しくなります。 */ 00076 #define NyLPC_TJsonRpcParserResult_NUMBER_OF_PARAM_INDEX 32 00077 /** 00078 * NyLPC_TJsonRpcParserResultが格納できるパラメータの総バイト数。 00079 * (文字数+1)+(uint32|int32)*4+uint8の合計値です。 00080 */ 00081 #define NyLPC_TJsonRpcParserResult_PARAM_BUF 256 00082 00083 /** 00084 * JSONRPC構文のパース結果を格納します。 00085 * 開発メモ 00086 * 更新する場合は、param_indexまでの構造体のレイアウトを破壊しないようにしてください。 00087 */ 00088 union NyLPC_TJsonRpcParserResult 00089 { 00090 NyLPC_TUInt8 type; //タイプ 00091 /** この構造体は type==NyLPC_TJsonRpcParserResult_TYPE_METHODのときに有効です。 */ 00092 struct{ 00093 NyLPC_TUInt8 _type; //タイプ 00094 NyLPC_TUInt8 func_number; //確定した関数番号。 00095 NyLPC_TUInt8 _padding[2]; //パディング 00096 NyLPC_TInt32 id; //idパラメータの値 00097 NyLPC_TChar param_buf[NyLPC_TJsonRpcParserResult_PARAM_BUF]; 00098 NyLPC_TUInt8 param_index[NyLPC_TJsonRpcParserResult_NUMBER_OF_PARAM_INDEX]; 00099 /** 関数の含まれるクラスへのポインタ。 */ 00100 const struct NyLPC_TJsonRpcClassDef* class_def; 00101 }method; 00102 struct{ 00103 NyLPC_TUInt8 _type; //タイプ 00104 NyLPC_TUInt8 _padding[3]; //パディング 00105 NyLPC_TInt32 id; //idパラメータの値 00106 NyLPC_TChar param_buf[NyLPC_TJsonRpcParserResult_PARAM_BUF]; 00107 NyLPC_TUInt8 param_index[NyLPC_TJsonRpcParserResult_NUMBER_OF_PARAM_INDEX]; 00108 }result; 00109 struct{ 00110 NyLPC_TUInt8 _type; //タイプ 00111 NyLPC_TInt8 _padding[3];// 00112 NyLPC_TInt32 _id; //idパラメータの値 00113 NyLPC_TChar param_buf[NyLPC_TJsonRpcParserResult_PARAM_BUF]; 00114 NyLPC_TUInt8 param_index[NyLPC_TJsonRpcParserResult_NUMBER_OF_PARAM_INDEX]; 00115 }raw; 00116 }; 00117 /** 00118 * Resultに格納される結果がハンドラを持っているかを返します。 00119 */ 00120 #define NyLPC_TJsonRpcParserResult_hasMethodHandler(i_struct) ((i_struct)->method.class_def->functions[(i_struct)->method.func_number].handler!=NULL) 00121 /** 00122 * Resultに含まれるハンドラを呼び出します。 00123 * @param i_struct 00124 * Result構造体 00125 * @param i_param 00126 * ハンドラに引き渡すパラメータ 00127 * @return 00128 * FALSEの場合、ループを終了してください。 00129 */ 00130 #define NyLPC_TJsonRpcParserResult_callMethodHandler(i_struct,i_param) (i_struct)->method.class_def->functions[(i_struct)->method.func_number].handler((i_struct),(i_param)) 00131 00132 /** 00133 * i_idx番目のパラメータをuint32としてo_valへ取り出します。 00134 */ 00135 NyLPC_TBool NyLPC_TJsonRpcParserResult_getUInt32(const union NyLPC_TJsonRpcParserResult* i_struct, NyLPC_TInt16 i_idx, NyLPC_TUInt32* o_val); 00136 /** 00137 * i_idx番目のパラメータをint32としてo_valへ取り出します。 00138 */ 00139 NyLPC_TBool NyLPC_TJsonRpcParserResult_getInt32(const union NyLPC_TJsonRpcParserResult* i_struct, NyLPC_TInt16 i_idx, NyLPC_TInt32* o_val); 00140 /** 00141 * i_idx番目のパラメータをchar[]としてo_valへ取り出します。 00142 */ 00143 NyLPC_TBool NyLPC_TJsonRpcParserResult_getStr(const union NyLPC_TJsonRpcParserResult* i_struct, NyLPC_TInt16 i_idx, const NyLPC_TChar** o_val, NyLPC_TUInt8* o_len); 00144 /** 00145 * i_idx番目のパラメータをuchar[]としてo_valへ取り出します。 00146 */ 00147 NyLPC_TBool NyLPC_TJsonRpcParserResult_getByteArray(const union NyLPC_TJsonRpcParserResult* i_struct, NyLPC_TInt16 i_idx, const NyLPC_TUInt8** o_val, NyLPC_TUInt8* o_len); 00148 00149 /** 00150 * i_idx番目のパラメータをuint8としてo_valへ取り出します。 00151 */ 00152 NyLPC_TBool NyLPC_TJsonRpcParserResult_getByte(const union NyLPC_TJsonRpcParserResult* i_struct, NyLPC_TInt16 i_idx, NyLPC_TUInt8* o_val); 00153 00154 /******************************************************************************** 00155 * 00156 * NyLPC_TcJsonRpcParser 00157 * 00158 ********************************************************************************/ 00159 /** 00160 * JSONRPCの型定義定数です。 00161 */ 00162 #define NyLPC_cJsonRpcParser_TYPE_INT32 'd' 00163 #define NyLPC_cJsonRpcParser_TYPE_UINT32 'u' 00164 #define NyLPC_cJsonRpcParser_TYPE_STRING 's' 00165 #define NyLPC_cJsonRpcParser_TYPE_BYTE 'b' 00166 #define NyLPC_cJsonRpcParser_TYPE_BSTRING 'B' 00167 00168 /** 00169 * JsonRPCメッセージをパースします。パース出来るメッセージは以下の通りです。 00170 * クラスは、RPC関数定義テーブルに従ってメッセージを分析し、テーブルに存在する関数のみを返却することができます。 00171 * <p> 00172 * メッセージ形式 00173 * <pre> 00174 * METHOD: 00175 * {"method":METHOD,"version":VERSION,"params":PARAMS,"id":ID} 00176 * METHOD VERSION as string 00177 * ID as uint32 00178 * PARAMS as Array of (string|uint32|int32) 00179 * </pre> 00180 * </p> 00181 */ 00182 typedef struct NyLPC_TcJsonRpcParser NyLPC_TcJsonRpcParser_t; 00183 00184 00185 00186 00187 /** パーサの状態値*/ 00188 typedef NyLPC_TUInt8 NyLPC_TcJsonRpcParser_TStatus; 00189 00190 #define NyLPC_TcJsonRpcParser_ST_START 0x01 //開始ブランケット受信待ち 00191 #define NyLPC_TcJsonRpcParser_ST_END 0x02 //終了受信済 00192 #define NyLPC_TcJsonRpcParser_ST_ERROR 0x03 //エラー発生 00193 #define NyLPC_TcJsonRpcParser_ST_NAME_Q 0x04 //名前クオート受信待ち 00194 #define NyLPC_TcJsonRpcParser_ST_NAME_STR 0x05 //名前受信中 00195 #define NyLPC_TcJsonRpcParser_ST_NV_SEP 0x06 //名前と値のセパレータ待ち 00196 #define NyLPC_TcJsonRpcParser_ST_VAL 0x07 //値開始待ち 00197 #define NyLPC_TcJsonRpcParser_ST_VAL_STR 0x08 //文字列受信 00198 #define NyLPC_TcJsonRpcParser_ST_VAL_UINT 0x09 //UINT受信中 00199 #define NyLPC_TcJsonRpcParser_ST_VAL_INT 0x10 //INT受信中 00200 #define NyLPC_TcJsonRpcParser_ST_NEXT 0x11 //次のNAMEもしくは終了ブランケット 00201 #define NyLPC_TcJsonRpcParser_ST_PARAMS 0x12 //PARAM要素パース中 00202 00203 00204 00205 /** NyLPC_TcJsonRpcParserの定数値です。 字句解析ワークメモリの長さ。256未満8*n-4の数を指定してください。 */ 00206 #define NyLPC_TcJsonRpcParser_WORK_MAX (48-4) 00207 00208 /** 00209 * クラス構造体です。 00210 */ 00211 struct NyLPC_TcJsonRpcParser 00212 { 00213 const struct NyLPC_TJsonRpcClassDef** _class_def; 00214 union{ 00215 struct{ 00216 NyLPC_TChar buf[NyLPC_TcJsonRpcParser_WORK_MAX]; //文字解析メモリ 00217 NyLPC_TUInt8 n; //字句解析の文字数 00218 NyLPC_TUInt8 st; //字句解析のサブステータス 00219 NyLPC_TUInt8 vt; //数字エスケープ解釈のテンポラリ 00220 NyLPC_TUInt8 _padding; // 00221 }str; 00222 struct{ 00223 NyLPC_TInt32 v; 00224 NyLPC_TInt8 s; 00225 }int32; 00226 NyLPC_TUInt32 uint32; 00227 }_work; 00228 NyLPC_TcJsonRpcParser_TStatus _st; //パーサステータス 00229 NyLPC_TUInt8 _pst; //PARAMS解析ステータス 00230 NyLPC_TUInt8 _name_id; //解析中のNAME_ID 00231 NyLPC_TUInt8 _pcounter; //パラメタ解析に使うワークカウンタ 00232 union NyLPC_TJsonRpcParserResult* _result;//出力格納先 00233 }; 00234 00235 00236 00237 00238 00239 00240 /** 00241 * インスタンスを初期化します。 00242 * @param i_class_def 00243 * クラステーブルの配列です。NULLで終端します。 00244 */ 00245 void NyLPC_cJsonRpcParser_initialize( 00246 NyLPC_TcJsonRpcParser_t* i_inst, 00247 const struct NyLPC_TJsonRpcClassDef** i_class_def); 00248 00249 #define NyLPC_cJsonRpcParser_finalize(i) 00250 /** 00251 * パーサの状態を初期化します。 00252 * @param i_result 00253 * パース結果の出力先構造体のアドレスです。 00254 */ 00255 void NyLPC_cJsonRpcParser_initParser(NyLPC_TcJsonRpcParser_t* i_inst, union NyLPC_TJsonRpcParserResult* i_result); 00256 /** 00257 * パーサに文字列を入力します。入力後は、NyLPC_cJsonRpcParser_getStatusでパーサの状態をチェックしてください。 00258 */ 00259 void NyLPC_cJsonRpcParser_putChar(NyLPC_TcJsonRpcParser_t* i_inst, char i_c); 00260 00261 #define NyLPC_cJsonRpcParser_getStatus(i) ((i)->_st) 00262 /** クラス定義テーブルを返します。*/ 00263 #define NyLPC_cJsonRpcParser_getClassDef(i) ((i)->_class_def) 00264 00265 00266 #ifdef __cplusplus 00267 } 00268 #endif /* __cplusplus */ 00269 00270 #endif /* NYLPC_CJSONRPCPARSER4_H_ */
Generated on Tue Jul 12 2022 15:46:16 by
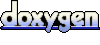