This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cIPv4Arp.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 * 00026 * Parts of this file were leveraged from uIP: 00027 * 00028 * Copyright (c) 2001-2003, Adam Dunkels. 00029 * All rights reserved. 00030 * 00031 * Redistribution and use in source and binary forms, with or without 00032 * modification, are permitted provided that the following conditions 00033 * are met: 00034 * 1. Redistributions of source code must retain the above copyright 00035 * notice, this list of conditions and the following disclaimer. 00036 * 2. Redistributions in binary form must reproduce the above copyright 00037 * notice, this list of conditions and the following disclaimer in the 00038 * documentation and/or other materials provided with the distribution. 00039 * 3. The name of the author may not be used to endorse or promote 00040 * products derived from this software without specific prior 00041 * written permission. 00042 * 00043 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS 00044 * OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00045 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00046 * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY 00047 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00048 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00049 * GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00050 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00051 * WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00052 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00053 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00054 */ 00055 #ifndef NYLPC_CIPV4ARP_H_ 00056 #define NYLPC_CIPV4ARP_H_ 00057 #include "../NyLPC_cIPv4Config.h" 00058 00059 #ifdef __cplusplus 00060 extern "C" { 00061 #endif /* __cplusplus */ 00062 00063 00064 struct NyLPC_TArpTableItem 00065 { 00066 struct NyLPC_TIPv4Addr ipaddr; 00067 struct NyLPC_TEthAddr ethaddr; 00068 NyLPC_TUInt8 time; 00069 }; 00070 00071 /********************************************************************** 00072 * 00073 * class NyLPC_TcIPv4Config 00074 * 00075 **********************************************************************/ 00076 00077 00078 #define NyLPC_TcIPv4Arp_ARPTAB_SIZE 8 00079 00080 00081 typedef struct NyLPC_TcIPv4Arp NyLPC_TcIPv4Arp_t; 00082 00083 /** 00084 * NyLPC_TcIPv4クラスの構造体です。 00085 */ 00086 struct NyLPC_TcIPv4Arp 00087 { 00088 const NyLPC_TcIPv4Config_t* _cfg; 00089 NyLPC_TUInt8 arptime; 00090 NyLPC_TUInt8 tmpage; 00091 struct NyLPC_TArpTableItem arp_table[NyLPC_TcIPv4Arp_ARPTAB_SIZE]; 00092 }; 00093 00094 void NyLPC_cIPv4Arp_initialize(NyLPC_TcIPv4Arp_t* i_inst,const NyLPC_TcIPv4Config_t* i_ref_config); 00095 #define NyLPC_cIPv4Arp_finalize(i_inst) 00096 void NyLPC_cIPv4Arp_periodic(NyLPC_TcIPv4Arp_t* i_inst); 00097 void NyLPC_cIPv4Arp_incomingIp(NyLPC_TcIPv4Arp_t* i_inst,const struct NyLPC_TEthernetIIHeader* i_eth,struct NyLPC_TIPv4Addr i_ip_src); 00098 /** 00099 * ARPメッセージを処理します。 00100 * @param o_tx_len 00101 * 戻り値がある場合そのサイズ 00102 * @return 00103 * 応答パケットを格納したcUipService_allocBufで確保したメモリ 00104 */ 00105 void* NyLPC_cIPv4Arp_rx(NyLPC_TcIPv4Arp_t* i_inst,const struct NyLPC_TArpHeader* i_arp, NyLPC_TUInt16 i_len, NyLPC_TUInt16* o_tx_len); 00106 const struct NyLPC_TEthAddr* NyLPC_cIPv4Arp_IPv4toEthAddr(NyLPC_TcIPv4Arp_t* i_inst,const struct NyLPC_TIPv4Addr i_ip_addr); 00107 00108 00109 #ifdef __cplusplus 00110 } 00111 #endif /* __cplusplus */ 00112 00113 00114 00115 #endif /* NYLPC_CIPV4ARP_H_ */ 00116
Generated on Tue Jul 12 2022 15:46:15 by
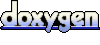