This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cHttpdConnection_protected.h
00001 /* 00002 * NyLPC_cHttpdConnection.h 00003 * 00004 * Created on: 2013/02/07 00005 * Author: nyatla 00006 */ 00007 00008 #ifndef NYLPC_CHTTPDCONNECTION_PROTECTED_H_ 00009 #define NYLPC_CHTTPDCONNECTION_PROTECTED_H_ 00010 #include "NyLPC_netif.h" 00011 #include "NyLPC_stdlib.h" 00012 #include "NyLPC_cHttpdConnection.h" 00013 #include "NyLPC_cHttpdUtils.h" 00014 00015 #ifdef __cplusplus 00016 extern "C" { 00017 #endif /* __cplusplus */ 00018 00019 /** 00020 * コネクションモード 00021 */ 00022 typedef NyLPC_TUInt8 NyLPC_TcHttpdConnection_CONNECTION_MODE; 00023 /** 00024 * 持続性接続 00025 */ 00026 #define NyLPC_TcHttpdConnection_CONNECTION_MODE_CONTINUE 1 00027 /** 00028 * 持続性接続キャンセル 00029 */ 00030 #define NyLPC_TcHttpdConnection_CONNECTION_MODE_CLOSE 2 00031 00032 /** 00033 * URL Prefixを返却します。 00034 */ 00035 const NyLPC_TChar* NyLPC_cHttpdConnection_getUrlPrefix(const NyLPC_TcHttpdConnection_t* i_inst); 00036 00037 /** 00038 * コネクションステータスをパース済みに設定します。 00039 * HttpModで処理を行った後にコールします。 00040 */ 00041 void NyLPC_cHttpdConnection_setReqStatusParsed(NyLPC_TcHttpdConnection_t* i_inst); 00042 00043 00044 /** 00045 * コネクションをHTTPレベルで閉じます。 00046 * ResponseステータスはCLOSEDになります。Requestステータスは変化しません。 00047 */ 00048 NyLPC_TBool NyLPC_cHttpdConnection_closeResponse(NyLPC_TcHttpdConnection_t* i_inst); 00049 00050 00051 /** 00052 * ソケットをlistenします。LISTEN状態のソケットに使えます。 00053 */ 00054 NyLPC_TBool NyLPC_cHttpdConnection_listenSocket(NyLPC_TcHttpdConnection_t* i_inst,NyLPC_TiTcpListener_t* i_listener); 00055 00056 /** 00057 * コネクションのソケットをacceptします。 00058 * listenSocketが成功したソケットにだけ使えます。 00059 * 関数が成功すると、REQStatusがHEADに遷移します。 00060 */ 00061 NyLPC_TBool NyLPC_cHttpdConnection_acceptSocket(NyLPC_TcHttpdConnection_t* i_inst); 00062 00063 /** 00064 * コネクションのソケットを閉じます。 00065 * ResponseステータスはCLOSEDになり、RequestステータスはLISTENになります。 00066 */ 00067 void NyLPC_cHttpdConnection_closeSocket(NyLPC_TcHttpdConnection_t* i_inst); 00068 00069 /** 00070 * ヘッダをprefetchします。 00071 */ 00072 NyLPC_TBool NyLPC_cHttpdConnection_prefetch(NyLPC_TcHttpdConnection_t* i_inst); 00073 00074 /** 00075 * コネクションのプリフェッチデータをヘッダパーサへpushします。 00076 */ 00077 NyLPC_TBool NyLPC_cHttpdConnection_pushPrefetchInfo(NyLPC_TcHttpdConnection_t* i_inst,NyLPC_TcHttpBasicHeaderParser_t* i_header_parser,struct NyLPC_THttpBasicHeader* o_out); 00078 00079 00080 /** 00081 * 次のプリフェッチを準備する。 00082 */ 00083 NyLPC_TBool NyLPC_cHttpdConnection_prevNextPrefetch(NyLPC_TcHttpdConnection_t* i_inst); 00084 00085 /** 00086 * リクエストのパースを完了する。 00087 */ 00088 void NyLPC_cHttpdConnection_requestParsed(NyLPC_TcHttpdConnection_t* i_inst); 00089 00090 00091 /** 00092 * コネクションのモードをセットする。 00093 * この関数はhttpdハンドラのコールするモジュールが呼び出すことが有る。 00094 * HTTPリクエストのバージョンが1.1かつconnectionがCLOSEで無い場合、CONTINUEを指定することで持続性接続に設定できる。 00095 * 関数は、NyLPC_cHttpdConnection_sendResponseHeaderをコールする前に実行すること。 00096 */ 00097 #define NyLPC_cHttpdConnection_setConnectionMode(i_inst,i_mode) (i_inst)->_connection_message_mode=(i_mode) 00098 00099 NyLPC_TUInt16 NyLPC_cHttpd_incNumOfConnection(NyLPC_TcHttpd_t* i_inst); 00100 NyLPC_TUInt16 NyLPC_cHttpd_decNumOfConnection(NyLPC_TcHttpd_t* i_inst); 00101 00102 #ifdef __cplusplus 00103 } 00104 #endif /* __cplusplus */ 00105 #endif /* NYLPC_CHTTPDCONNECTION_H_ */
Generated on Tue Jul 12 2022 15:46:15 by
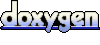