This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cHttpdConnection.h
00001 /* 00002 * NyLPC_cHttpdConnection.h 00003 * 00004 * Created on: 2013/02/07 00005 * Author: nyatla 00006 */ 00007 00008 #ifndef NYLPC_CHTTPDCONNECTION_H_ 00009 #define NYLPC_CHTTPDCONNECTION_H_ 00010 #include "NyLPC_stdlib.h" 00011 #include "NyLPC_cHttpRequestPrefixParser.h" 00012 00013 #ifdef __cplusplus 00014 extern "C" { 00015 #endif /* __cplusplus */ 00016 00017 #ifndef DEFINE_NyLPC_TcHttpd_t 00018 typedef struct NyLPC_TcHttpd NyLPC_TcHttpd_t; 00019 #define DEFINE_NyLPC_TcHttpd_t 00020 #endif 00021 00022 00023 typedef NyLPC_TUInt8 NyLPC_TcHttpdConnection_Status; 00024 00025 /** リクエストプレフィクスを読み出した状態*/ 00026 #define NyLPC_cHttpdConnection_ReqStatus_LISTEN 0 00027 /** ACCEPT待ち*/ 00028 #define NyLPC_cHttpdConnection_ReqStatus_ACCEPT 1 00029 /** コネクションは接続済*/ 00030 #define NyLPC_cHttpdConnection_ReqStatus_PREFETCH 2 00031 /** リクエストパース待ち*/ 00032 #define NyLPC_cHttpdConnection_ReqStatus_REQPARSE 3 00033 /** リクエストパース済*/ 00034 #define NyLPC_cHttpdConnection_ReqStatus_END 4 00035 #define NyLPC_cHttpdConnection_ReqStatus_BODYPARSE 5 00036 00037 00038 00039 00040 /** レスポンスヘッダ送付済*/ 00041 #define NyLPC_cHttpdConnection_ResStatus_HEAD 1 00042 /** レスポンスヘッダ送付済*/ 00043 #define NyLPC_cHttpdConnection_ResStatus_BODY 2 00044 /** レスポンスBODY送付済*/ 00045 #define NyLPC_cHttpdConnection_ResStatus_CLOSED 3 00046 /** エラーが発生した。*/ 00047 #define NyLPC_cHttpdConnection_ResStatus_ERROR 4 00048 00049 00050 00051 /** 00052 * Httpdのハンドラが引き渡す、HTTPDコネクションクラス。 00053 * 00054 */ 00055 #ifndef DEFINE_NyLPC_TcHttpdConnection_t 00056 typedef struct NyLPC_TcHttpdConnection NyLPC_TcHttpdConnection_t; 00057 #define DEFINE_NyLPC_TcHttpdConnection_t 00058 #endif 00059 struct NyLPC_TcHttpdConnection 00060 { 00061 NyLPC_TUInt8 _req_status;//リクエストステータス 00062 NyLPC_TUInt8 _res_status;//レスポンスステータス 00063 NyLPC_TUInt8 _connection_message_mode;//COnnection:closeをヘッダに書き込むかのフラグ 00064 NyLPC_TcHttpd_t* _parent_httpd; //NyLPC_cHttpd 00065 NyLPC_TiTcpSocket_t* _socket; 00066 NyLPC_TcHttpStream_t _in_stream; 00067 NyLPC_TcHttpRequestPrefixParser_t _pparser; 00068 union{ 00069 NyLPC_TcHttpBodyWriter_t _body_writer; 00070 NyLPC_TcHttpHeaderWriter_t _head_writer; 00071 }; 00072 }; 00073 00074 /** 00075 * @param i_parent_httpd 00076 * 00077 */ 00078 NyLPC_TBool NyLPC_cHttpdConnection_initialize(NyLPC_TcHttpdConnection_t* i_inst,NyLPC_TcHttpd_t* i_parent_httpd); 00079 void NyLPC_cHttpdConnection_finalize(NyLPC_TcHttpdConnection_t* i_inst); 00080 00081 /** 00082 * 100 Continueをストリームに送信する。 00083 * HTTP/1.1でPOSTメッセージを受け付けた場合にコールすること。 00084 * この関数はステータスがNyLPC_cHttpdConnection_ResStatus_HEADの時だけ実行できる。 00085 */ 00086 NyLPC_TBool NyLPC_cHttpdConnection_send100Continue(NyLPC_TcHttpdConnection_t* i_inst); 00087 00088 00089 /** 00090 * レスポンスヘッダを送信します。 00091 * BodyはChunkedエンコーディングで送信します。 00092 * @param i_additional_header 00093 * メッセージフィールドに追加する文字列です。 00094 * \r\nで終端下文字列を指定して下さい。 00095 */ 00096 NyLPC_TBool NyLPC_cHttpdConnection_sendResponseHeader(NyLPC_TcHttpdConnection_t* i_inst,NyLPC_TUInt16 i_response_code,const NyLPC_TChar* i_content_type,const NyLPC_TChar* i_additional_header); 00097 /** 00098 * レスポンスヘッダを送信します。 00099 * BodyはContentLengthを伴って送信します。Body送信時にサイズチェックは行いません。 00100 * @param i_content_length 00101 * 最大で0x0fffffffを指定できます。 00102 * @param i_additional_header 00103 * メッセージフィールドに追加する文字列です。 00104 * \r\nで終端下文字列を指定して下さい。 00105 */ 00106 NyLPC_TBool NyLPC_cHttpdConnection_sendResponseHeader2(NyLPC_TcHttpdConnection_t* i_inst,NyLPC_TUInt16 i_response_code,const NyLPC_TChar* i_content_type,NyLPC_TUInt32 i_content_length,const NyLPC_TChar* i_additional_header); 00107 /** 00108 * レスポンスBodyを送信します。 00109 * 関数を実行後、_res_statusはBODYかERRORに遷移します。 00110 */ 00111 NyLPC_TBool NyLPC_cHttpdConnection_sendResponseBody(NyLPC_TcHttpdConnection_t* i_inst,const void* i_data,NyLPC_TUInt32 i_size); 00112 00113 /** 00114 * レスポンスBodyを書式出力して送信します。 00115 * 関数を実行後、_res_statusはBODYかERRORに遷移します。 00116 */ 00117 NyLPC_TBool NyLPC_cHttpdConnection_sendResponseBodyF(NyLPC_TcHttpdConnection_t* i_inst,const char* i_fmt,...); 00118 00119 00120 /** 00121 * Httpd全体で唯一のロックを取得する。 00122 */ 00123 void NyLPC_cHttpdConnection_lock(NyLPC_TcHttpdConnection_t* i_inst); 00124 00125 /** 00126 * Httpd全体で唯一のロックを開放する。 00127 */ 00128 void NyLPC_cHttpdConnection_unlock(NyLPC_TcHttpdConnection_t* i_inst); 00129 00130 00131 /** 00132 * コネクションのStreamを返します。 00133 */ 00134 #define NyLPC_cHttpdConnection_refStream(i_inst) (&(i_inst->_in_stream.super)) 00135 00136 #define NyLPC_cHttpdConnection_getMethod(i_inst) ((i_inst)->_pparser.method) 00137 #define NyLPC_cHttpdConnection_getReqStatus(i_inst) ((i_inst)->_req_status) 00138 00139 #ifdef __cplusplus 00140 } 00141 #endif /* __cplusplus */ 00142 00143 #endif /* NYLPC_CHTTPDCONNECTION_H_ */
Generated on Tue Jul 12 2022 15:46:15 by
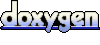