This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cHttpHeaderWriter.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #ifndef NYLPC_CHTTPHEADERWRITER_H_ 00027 #define NYLPC_CHTTPHEADERWRITER_H_ 00028 00029 #include "NyLPC_stdlib.h" 00030 #include "NyLPC_netif.h" 00031 #include "NyLPC_cHttpStream.h" 00032 #include "NyLPC_cHttpBasicHeaderParser.h" 00033 #include "NyLPC_cHttpdConfig.h" 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif /* __cplusplus */ 00038 00039 00040 typedef struct NyLPC_TcHttpHeaderWriter NyLPC_TcHttpHeaderWriter_t; 00041 struct NyLPC_TcHttpHeaderWriter 00042 { 00043 NyLPC_TUInt8 _is_chunked; 00044 NyLPC_TUInt8 _is_close; 00045 //異常状態をチェックするためのフラグ 00046 NyLPC_TUInt8 _is_error; 00047 00048 NyLPC_TUInt32 _content_length; 00049 NyLPC_TUInt32 _size_of_sent; 00050 00051 NyLPC_TiHttpPtrStream_t* _ref_stream; 00052 }; 00053 00054 /** 00055 * Httpリクエストヘッダに対応したHttpヘッダライタを構築します。 00056 * インスタンスは、正常/異常の2状態を持ちます。 00057 * <p>初期値について - 00058 * ConnectionCloseは有効です。 00059 * </p> 00060 */ 00061 NyLPC_TBool NyLPC_cHttpHeaderWriter_initialize(NyLPC_TcHttpHeaderWriter_t* i_inst,NyLPC_TiHttpPtrStream_t* i_ref_stream,const struct NyLPC_THttpBasicHeader* i_req_header); 00062 00063 #define NyLPC_cHttpHeaderWriter_finalize(i) 00064 NyLPC_TBool NyLPC_cHttpHeaderWriter_writeRequestHeader(NyLPC_TcHttpHeaderWriter_t* i_inst,NyLPC_THttpMethodType i_method,const struct NyLPC_TIPv4Addr* i_host,NyLPC_TUInt16 i_port,const NyLPC_TChar* i_path); 00065 NyLPC_TBool NyLPC_cHttpHeaderWriter_writeResponseHeader(NyLPC_TcHttpHeaderWriter_t* i_inst,NyLPC_TUInt16 i_status); 00066 NyLPC_TBool NyLPC_cHttpHeaderWriter_writeMessage(NyLPC_TcHttpHeaderWriter_t* i_inst,const NyLPC_TChar* i_name,const NyLPC_TChar* i_field); 00067 /** 00068 * \r\n区切りのメッセージをそのままヘッダに挿入します。i_additional_headerの終端は\r\nで閉じてください。 00069 */ 00070 NyLPC_TBool NyLPC_cHttpHeaderWriter_writeRawMessage(NyLPC_TcHttpHeaderWriter_t* i_inst,const NyLPC_TChar* i_additional_header); 00071 NyLPC_TBool NyLPC_cHttpHeaderWriter_close(NyLPC_TcHttpHeaderWriter_t* i_inst); 00072 void NyLPC_cHttpHeaderWriter_setContentLength(NyLPC_TcHttpHeaderWriter_t* i_inst,NyLPC_TUInt32 i_content_length); 00073 /** 00074 * ConnectionCloseのON/OFFを設定します。 00075 */ 00076 void NyLPC_cHttpHeaderWriter_setConnectionClose(NyLPC_TcHttpHeaderWriter_t* i_inst,NyLPC_TBool i_is_close); 00077 void NyLPC_cHttpHeaderWriter_setChunked(NyLPC_TcHttpHeaderWriter_t* i_inst); 00078 00079 #ifdef __cplusplus 00080 } 00081 #endif /* __cplusplus */ 00082 00083 #endif /* NYLPC_CHTTPHEADERWRITER_H_ */
Generated on Tue Jul 12 2022 15:46:15 by
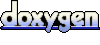