This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cHttpClient.h
00001 /* 00002 * NyLPC_cHttpClient.h 00003 * 00004 * Created on: 2013/08/24 00005 * Author: nyatla 00006 */ 00007 00008 #ifndef NYLPC_CHTTPCLIENT_H_ 00009 #define NYLPC_CHTTPCLIENT_H_ 00010 00011 #include "NyLPC_stdlib.h" 00012 #include "NyLPC_net.h" 00013 #include "NyLPC_http.h" 00014 #include "NyLPC_netif.h" 00015 00016 #ifdef __cplusplus 00017 extern "C" { 00018 #endif /* __cplusplus */ 00019 00020 typedef struct NyLPC_TcHttpClient NyLPC_TcHttpClient_t; 00021 00022 #define NyLPC_cHttpHeaderWriter_CONTENT_LENGTH_UNLIMITED 0xffffffff 00023 struct NyLPC_TcHttpClient 00024 { 00025 NyLPC_TUInt8 _state; 00026 NyLPC_TUInt8 _padding1; 00027 NyLPC_TiTcpSocket_t* _sock; 00028 NyLPC_TcHttpStream_t _stream; 00029 union{ 00030 NyLPC_TcHttpHeaderWriter_t head_writer; 00031 NyLPC_TcHttpBodyWriter_t body_writer; 00032 NyLPC_TcHttpBasicHeaderParser_t head_parser; 00033 NyLPC_TcHttpBodyParser_t body_parser; 00034 }pw; 00035 }; 00036 00037 00038 NyLPC_TBool NyLPC_cHttpClient_initialize(NyLPC_TcHttpClient_t* i_inst,void* i_rx_buf,NyLPC_TUInt16 i_rx_size); 00039 00040 void NyLPC_cHttpClient_finalize(NyLPC_TcHttpClient_t* i_inst); 00041 00042 /** 00043 * サーバとの接続を切断する。 00044 * ステータスはCLOSEDになる。 00045 */ 00046 void NyLPC_cHttpClient_close(NyLPC_TcHttpClient_t* i_inst); 00047 00048 00049 /** 00050 * サーバに接続する。 00051 * 関数はステータスをIDLEへ遷移する。 00052 * インスタンスのステータスは何でも構わない。 00053 * @return 00054 * TRUE - ステータスはIDLEへ遷移する。 00055 * FALSE - ステータスはCLOSEDへ遷移する。 00056 */ 00057 NyLPC_TBool NyLPC_cHttpClient_connect(NyLPC_TcHttpClient_t* i_inst,const struct NyLPC_TIPv4Addr* i_addr,NyLPC_TUInt16 i_port); 00058 00059 00060 00061 /** 00062 * POSTリクエストを送信する。 00063 * ステータスはIDLEである必要がある。 00064 * @param i_content_length 00065 * 送信bodyのサイズ。最大 0xfffffffe 00066 * NyLPC_cHttpHeaderWriter_CONTENT_LENGTH_UNLIMITEDの場合はChunked転送になる。 00067 * @return 00068 * 引き続き処理が可能かを返す。 00069 * TRUE - 成功。ステータスはSEND_REQ_BODYになる。write/getStatusを呼び出せる。 00070 * FALSE - 失敗。ステータスはCLOSEDになる。 00071 */ 00072 NyLPC_TBool NyLPC_cHttpClient_sendMethod( 00073 NyLPC_TcHttpClient_t* i_inst, 00074 NyLPC_THttpMethodType i_method, 00075 const NyLPC_TChar* i_path, 00076 NyLPC_TUInt32 i_content_length, 00077 const NyLPC_TChar* i_mime_type, 00078 const NyLPC_TChar* i_additional_header); 00079 00080 00081 /** 00082 * POSTリクエストのデータを送信する。 00083 * ステータスはSEND_REQ_BODYである必要がある。 00084 * @return 00085 * TRUE - 成功。 00086 * FALSE - 失敗。ステータスはCLOSEDになる。 00087 */ 00088 NyLPC_TBool NyLPC_cHttpClient_write(NyLPC_TcHttpClient_t* i_inst,const void* i_buf,NyLPC_TUInt32 i_buf_size); 00089 00090 /** 00091 * 書式文字列としてPOSTリクエストのデータを送信する。 00092 * ステータスはSEND_REQ_BODYである必要がある。 00093 * @param i_fmt 00094 * printfライクなフォーマット文字列 00095 * @return 00096 * TRUE - 成功。 00097 * FALSE - 失敗。ステータスはCLOSEDになる。 00098 */ 00099 NyLPC_TBool NyLPC_cHttpClient_writeFormat(NyLPC_TcHttpClient_t* i_inst,const NyLPC_TChar* i_fmt,...); 00100 NyLPC_TBool NyLPC_cHttpClient_writeFormatV(NyLPC_TcHttpClient_t* i_inst,const NyLPC_TChar* i_fmt,va_list i_args); 00101 00102 /** 00103 * ステータスコードを返す。 00104 * ステータスはSEND_REQ_BODYである必要がある。 00105 * @return 00106 * 0 - 失敗。ステータスはCLOSEDになる。 00107 * その他 - ステータスコード。ステータスはRECV_RES_BODYになる。 00108 */ 00109 NyLPC_TUInt16 NyLPC_cHttpClient_getStatus(NyLPC_TcHttpClient_t* i_inst); 00110 00111 00112 /** 00113 * GET/POSTリクエストで受信したデータを読み出す。 00114 * ステータスはRECV_RES_BODYである必要がある。 00115 * @param o_read_len 00116 * 戻り値TRUEの場合のみ有効。データ終端に達した場合は0になる。 00117 * @return 00118 * TRUE:正常読み出し。o_read_lenの値で終端判定 00119 * FALSE:失敗。コネクションはクローズされる。 00120 */ 00121 NyLPC_TBool NyLPC_cHttpClient_read(NyLPC_TcHttpClient_t* i_inst,void* i_buf,NyLPC_TUInt32 i_buf_size,NyLPC_TInt16* o_read_len); 00122 00123 00124 00125 00126 00127 00128 #ifdef __cplusplus 00129 } 00130 #endif /* __cplusplus */ 00131 00132 #endif /* NYLPC_CHTTPCLIENT_H_ */ 00133
Generated on Tue Jul 12 2022 15:46:15 by
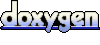