This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cFormatTextReader.c
00001 /** 00002 * @file 00003 * NyLPC_cFormatTextReader.c 00004 * このクラスは、書式テキスト読み出し関数を集約します。 00005 * Created on: 2013/04/18 00006 * Author: nyatla 00007 */ 00008 #include <ctype.h> 00009 #include <stdlib.h> 00010 #include <string.h> 00011 #include "NyLPC_cFormatTextReader.h" 00012 /** 00013 * [a-zA-Z0-9_-]で構成されるワードを取得します。 00014 * This function peek a word from string. 00015 * @return 00016 * size of seeked. 00017 */ 00018 NyLPC_TInt32 NyLPC_cFormatTextReader_readWord(const NyLPC_TChar* buf,const NyLPC_TChar** top) 00019 { 00020 const NyLPC_TChar* p=buf; 00021 *top=p; 00022 for(;*p!='\0' && (isalnum(*p)|| (strchr("_-",*p)!=NULL));p++);//skip words 00023 return p-(*top); 00024 } 00025 00026 /** 00027 * 文字列からIPアドレスを取得します。 00028 * [:number:]\.[:number:]\.[:number:]\.[:number:] 00029 * [:number:]は0-255までに制限されます。 00030 * @param v 00031 * uint8[4] 00032 * @return 00033 * next pointer 00034 */ 00035 NyLPC_TInt32 NyLPC_cFormatTextReader_readIpAddr(const NyLPC_TChar* buf,NyLPC_TUInt8* v) 00036 { 00037 NyLPC_TInt32 t; 00038 const NyLPC_TChar* p=buf; 00039 NyLPC_TInt32 i; 00040 for(i=0;i<4;i++){ 00041 t=0; 00042 for(;isdigit(*p);p++){ 00043 t=t*10+NyLPC_ctoi(*p); 00044 if(t>255){ 00045 return 0; 00046 } 00047 } 00048 v[i]=t; 00049 if(i<3){ 00050 if(*p!='.'){ 00051 return 0; 00052 } 00053 p++; 00054 }else if(!isspace(*p) && *p!='\0'){ 00055 return 0; 00056 } 00057 } 00058 return (p-buf); 00059 } 00060 /** 00061 * 文字列から10進数の数値を読み出します。 00062 * @return 00063 * 読み出した文字数 00064 */ 00065 NyLPC_TInt32 NyLPC_cFormatTextReader_readUInt(const NyLPC_TChar* buf,NyLPC_TUInt32* v) 00066 { 00067 NyLPC_TUInt32 t; 00068 const NyLPC_TChar* p=buf; 00069 t=0; 00070 for(;isdigit(*p);p++){ 00071 t=t*10+NyLPC_ctoi(*p); 00072 } 00073 *v=t; 00074 return (p-buf); 00075 } 00076 00077 /** 00078 * 文字列からMACアドレスを取得します。 00079 * [:hex:]:[:hex:]:[:hex:]:[:hex:] 00080 * @param v 00081 * uint8[6] 00082 */ 00083 NyLPC_TInt32 NyLPC_cFormatTextReader_readMacAddr(const NyLPC_TChar* buf,NyLPC_TUInt8* v) 00084 { 00085 NyLPC_TInt32 t,i; 00086 const NyLPC_TChar* p=buf; 00087 for(i=0;i<6;i++){ 00088 t=0; 00089 for(;isxdigit(*p);p++){ 00090 t=t*16+NyLPC_ctox(*p); 00091 if(t>255){ 00092 return 0; 00093 } 00094 } 00095 v[i]=t; 00096 if(i<5){ 00097 if(*p!=':'){ 00098 return 0; 00099 } 00100 p++; 00101 }else if(!isspace(*p) && *p!='\0'){ 00102 return 0; 00103 } 00104 } 00105 return (p-buf); 00106 } 00107 /** 00108 * 連続するスペースを読み飛ばします。 00109 * @return 00110 * 読み飛ばしたスペース 00111 */ 00112 NyLPC_TInt32 NyLPC_cFormatTextReader_seekSpace(const NyLPC_TChar* s) 00113 { 00114 const NyLPC_TChar* p=s; 00115 for(;*p!='\0' && isspace(*p);p++); 00116 return p-s; 00117 } 00118 00119 00120
Generated on Tue Jul 12 2022 15:46:15 by
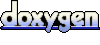