This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cFifoBuffer.c
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 *********************************************************************************/ 00026 #include "NyLPC_cFifoBuffer.h" 00027 #include <string.h> 00028 00029 /** 00030 * See Header file. 00031 */ 00032 void NyLPC_cFifoBuffer_initialize(NyLPC_TcFifoBuffer_t* i_inst,void* i_buf,NyLPC_TUInt16 i_buf_size) 00033 { 00034 i_inst->len=0; 00035 i_inst->size=i_buf_size; 00036 i_inst->buf=i_buf; 00037 } 00038 00039 00040 /** 00041 * See Header file. 00042 */ 00043 void NyLPC_cFifoBuffer_push(NyLPC_TcFifoBuffer_t* i_inst,const void* i_data,NyLPC_TUInt16 i_data_len) 00044 { 00045 NyLPC_TUInt8* wp; 00046 NyLPC_ArgAssert(NyLPC_cFifoBuffer_getSpace(i_inst)>=i_data_len); 00047 00048 wp=((NyLPC_TUInt8*)(i_inst->buf))+i_inst->len; 00049 memcpy(wp,i_data,i_data_len); 00050 i_inst->len+=i_data_len; 00051 } 00052 void* NyLPC_cFifoBuffer_prePush(NyLPC_TcFifoBuffer_t* i_inst,NyLPC_TUInt16 i_data_len) 00053 { 00054 NyLPC_TUInt8* wp; 00055 NyLPC_ArgAssert(NyLPC_cFifoBuffer_getSpace(i_inst)>=i_data_len); 00056 wp=((NyLPC_TUInt8*)(i_inst->buf))+i_inst->len; 00057 i_inst->len+=i_data_len; 00058 return wp; 00059 } 00060 00061 /** 00062 * See Header file. 00063 */ 00064 void NyLPC_cFifoBuffer_pop(NyLPC_TcFifoBuffer_t* i_inst,NyLPC_TUInt16 i_len) 00065 { 00066 NyLPC_TUInt8* wp; 00067 NyLPC_TUInt8* rp; 00068 NyLPC_ArgAssert(NyLPC_cFifoBuffer_getLength(i_inst)>=i_len); 00069 wp=(NyLPC_TUInt8*)i_inst->buf; 00070 rp=wp+i_len; 00071 i_inst->len-=i_len; 00072 memmove(wp,rp,i_inst->len); 00073 } 00074 00075 /** 00076 * See Header file. 00077 */ 00078 void* NyLPC_cFifoBuffer_getPtr(const NyLPC_TcFifoBuffer_t* i_inst) 00079 { 00080 return (void*)(i_inst->buf); 00081 } 00082 /** 00083 * See Header file. 00084 */ 00085 NyLPC_TUInt16 NyLPC_cFifoBuffer_getLength(const NyLPC_TcFifoBuffer_t* i_inst) 00086 { 00087 return i_inst->len; 00088 } 00089 /** 00090 * See Header file. 00091 */ 00092 NyLPC_TUInt16 NyLPC_cFifoBuffer_getSpace(const NyLPC_TcFifoBuffer_t* i_inst) 00093 { 00094 return i_inst->size-i_inst->len; 00095 }
Generated on Tue Jul 12 2022 15:46:15 by
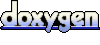