This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_cApipa.c
00001 #include "NyLPC_cApipa.h" 00002 #include "../NyLPC_cNet.h" 00003 #include <stdio.h> 00004 #include <string.h> 00005 00006 00007 /** 00008 * ARPテーブルに指定IPが現れるまで待ちます。 00009 */ 00010 static NyLPC_TBool waitForArpResponse(const struct NyLPC_TIPv4Addr* i_ip,NyLPC_TUInt32 i_wait_in_ms) 00011 { 00012 NyLPC_TcStopwatch_t sw; 00013 NyLPC_cStopwatch_initialize(&sw); 00014 NyLPC_cStopwatch_startExpire(&sw,i_wait_in_ms); 00015 while(!NyLPC_cStopwatch_isExpired(&sw)){ 00016 NyLPC_cThread_yield(); 00017 if(NyLPC_cNet_hasArpInfo(i_ip)){ 00018 return NyLPC_TBool_TRUE; 00019 } 00020 } 00021 NyLPC_cStopwatch_finalize(&sw); 00022 return NyLPC_TBool_FALSE; 00023 } 00024 static void makeIP(NyLPC_TcApipa_t* i_inst,struct NyLPC_TIPv4Addr* i_ip) 00025 { 00026 // NyLPC_TIPv4Addr_set(i_ip,192,168,128,206);//for conflict test! 00027 NyLPC_TIPv4Addr_set(i_ip,169,254,(i_inst->_seed>>8)&0xff,i_inst->_seed & 0xff); 00028 } 00029 static void updateSeed(NyLPC_TcApipa_t* i_inst) 00030 { 00031 do{ 00032 i_inst->_seed=(391*i_inst->_seed+392); 00033 }while(((i_inst->_seed & 0xff)==0) || ((i_inst->_seed & 0xff00)==0)); 00034 } 00035 00036 00037 00038 void NyLPC_cApipa_initialize(NyLPC_TcApipa_t* i_inst) 00039 { 00040 i_inst->_seed=0; 00041 } 00042 00043 00044 /** 00045 * この関数はuipを操作します。 00046 * cNetは停止中である必要があります。 00047 */ 00048 NyLPC_TBool NyLPC_cApipa_requestAddr(NyLPC_TcApipa_t* i_inst,NyLPC_TcIPv4Config_t* i_cfg,NyLPC_TInt16 i_repeat) 00049 { 00050 int i; 00051 NyLPC_TcIPv4Config_t cfg; 00052 struct NyLPC_TIPv4Addr caip; 00053 00054 //ゼロコンフィギュレーション用のIPを設定 00055 NyLPC_cIPv4Config_initialzeCopy(&cfg,i_cfg); 00056 //seedを更新 00057 for(i=0;i<6;i++) 00058 { 00059 i_inst->_seed+=i_cfg->eth_mac.addr[i]; 00060 } 00061 NyLPC_cIPv4Config_setDefaultRoute(&cfg,&NyLPC_TIPv4Addr_ZERO); 00062 for(i=i_repeat-1;i>=0;i--){ 00063 NyLPC_cIPv4Config_setIp(&cfg,&NyLPC_TIPv4Addr_ZERO,&NyLPC_TIPv4Addr_ZERO); 00064 updateSeed(i_inst); 00065 makeIP(i_inst,&caip); 00066 //startInterface 00067 NyLPC_cNet_start(&cfg); 00068 NyLPC_cNet_sendArpRequest(&caip); 00069 //テーブル更新待ち 00070 if(waitForArpResponse(&caip,512+(i_inst->_seed % 256))){ 00071 NyLPC_cNet_stop(); 00072 continue; 00073 } 00074 NyLPC_cNet_stop(); 00075 //IPのコンフリクトテスト 00076 NyLPC_cIPv4Config_setIp(&cfg,&caip,&NyLPC_TIPv4Addr_APIPA_MASK); 00077 NyLPC_cNet_start(&cfg); 00078 //!ARP送信 00079 NyLPC_cNet_sendArpRequest(&caip); 00080 if(waitForArpResponse(&caip,512+(256-(i_inst->_seed % 256)))){ 00081 //応答があったらエラー 00082 NyLPC_cNet_stop(); 00083 continue; 00084 } 00085 //OK 00086 NyLPC_cNet_stop(); 00087 NyLPC_cIPv4Config_setIp(i_cfg,&cfg.ip_addr,&cfg.netmask); 00088 return NyLPC_TBool_TRUE; 00089 } 00090 return NyLPC_TBool_FALSE; 00091 }
Generated on Tue Jul 12 2022 15:46:15 by
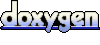