This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_NetIf_ip_types.h
00001 /********************************************************************************* 00002 * PROJECT: MiMic 00003 * -------------------------------------------------------------------------------- 00004 * 00005 * This file is part of MiMic 00006 * Copyright (C)2011 Ryo Iizuka 00007 * 00008 * MiMic is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU Lesser General Public License as published 00010 * by the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU Lesser General Public License 00019 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * For further information please contact. 00022 * http://nyatla.jp/ 00023 * <airmail(at)ebony.plala.or.jp> or <nyatla(at)nyatla.jp> 00024 * 00025 * 00026 * Parts of this file were leveraged from uIP: 00027 * 00028 * Copyright (c) 2001-2003, Adam Dunkels. 00029 * All rights reserved. 00030 * 00031 * Redistribution and use in source and binary forms, with or without 00032 * modification, are permitted provided that the following conditions 00033 * are met: 00034 * 1. Redistributions of source code must retain the above copyright 00035 * notice, this list of conditions and the following disclaimer. 00036 * 2. Redistributions in binary form must reproduce the above copyright 00037 * notice, this list of conditions and the following disclaimer in the 00038 * documentation and/or other materials provided with the distribution. 00039 * 3. The name of the author may not be used to endorse or promote 00040 * products derived from this software without specific prior 00041 * written permission. 00042 * 00043 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS 00044 * OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00045 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00046 * ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY 00047 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00048 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00049 * GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00050 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00051 * WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00052 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00053 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00054 */ 00055 #ifndef NyLPC_NETIF_IP_TYPES_h 00056 #define NyLPC_NETIF_IP_TYPES_h 00057 #include "NyLPC_config.h" 00058 #include "NyLPC_stdlib.h" 00059 #include "NyLPC_NetIf_ethernet_types.h" 00060 #include "NyLPC_NetIf_ip_types.h" 00061 00062 #ifdef __cplusplus 00063 extern "C" { 00064 #endif /* __cplusplus */ 00065 00066 #define UIP_PROTO_ICMP 1 00067 #define UIP_PROTO_TCP 6 00068 #define UIP_PROTO_UDP 17 00069 #define UIP_PROTO_ICMP6 58 00070 00071 #define ARP_REQUEST 1 00072 #define ARP_REPLY 2 00073 #define ARP_HWTYPE_ETH 1 00074 00075 /** イーサネットヘッダのサイズ値*/ 00076 #define UIP_ETHERHEADER_LEN 14 00077 #define UIP_TCPH_LEN 20 /* Size of TCP header */ 00078 #define UIP_IPH_LEN 20 /* Size of IP header */ 00079 00080 #ifndef PACK_STRUCT_END 00081 #define PACK_STRUCT_END __attribute((packed)) 00082 #endif 00083 00084 /********************************************************************** 00085 * 00086 * 00087 * 00088 **********************************************************************/ 00089 00090 /** 00091 * IPアドレスを格納します。 00092 * IPアドレスは、ネットワークオーダーで設定します。 00093 */ 00094 struct NyLPC_TIPv4Addr 00095 { 00096 NyLPC_TUInt32 v; 00097 }PACK_STRUCT_END; 00098 extern const struct NyLPC_TIPv4Addr NyLPC_TIPv4Addr_ZERO; 00099 extern const struct NyLPC_TIPv4Addr NyLPC_TIPv4Addr_ALL; 00100 extern const struct NyLPC_TIPv4Addr NyLPC_TIPv4Addr_BROADCAST; 00101 extern const struct NyLPC_TIPv4Addr NyLPC_TIPv4Addr_MULTICAST; 00102 extern const struct NyLPC_TIPv4Addr NyLPC_TIPv4Addr_MULTICAST_MASK; 00103 extern const struct NyLPC_TIPv4Addr NyLPC_TIPv4Addr_APIPA_MASK; 00104 00105 /** 00106 * addr1とaddr2が全く同じであるかをテストします。 00107 * \hideinitializer 00108 */ 00109 #define NyLPC_TIPv4Addr_isEqual(v1,v2) ((v1)->v==(v2)->v) 00110 /** 00111 * addr1とaddr2をmaskでマスクした結果を比較します。 00112 * \hideinitializer 00113 */ 00114 #define NyLPC_TIPv4Addr_isEqualWithMask(addr1, addr2, mask) ((((addr1)->v) & ((mask)->v))==(((addr2)->v) & ((mask)->v))) 00115 00116 /** 00117 * 変数にIP v4アドレスをセットします。 00118 * 次のように使います。 00119 \code 00120 NyLPC_TIPv4Addr ip; 00121 NyLPC_TIpv4Addr_set(&ip,1,2,3,4); 00122 \endcode 00123 */ 00124 #define NyLPC_TIPv4Addr_set(s,a0,a1,a2,a3) (s)->v=NyLPC_htonl((0xff000000&(((NyLPC_TUInt32)(a0))<<24))|(0x00ff0000&(((NyLPC_TUInt32)(a1))<<16))|(0x0000ff00&(((NyLPC_TUInt32)(a2))<<8))|(0x000000ff&((NyLPC_TUInt32)(a3)))) 00125 #define NyLPC_TIPv4Addr_pack(a0,a1,a2,a3) {NyLPC_HTONL((0xff000000&(((NyLPC_TUInt32)(a0))<<24))|(0x00ff0000&(((NyLPC_TUInt32)(a1))<<16))|(0x0000ff00&(((NyLPC_TUInt32)(a2))<<8))|(0x000000ff&((NyLPC_TUInt32)(a3))))} 00126 00127 /** 00128 * IPアドレスを文字列に変換して返します。 00129 */ 00130 NyLPC_TInt16 NyLPC_TIPv4Addr_toString(const struct NyLPC_TIPv4Addr* i_ip,NyLPC_TChar* i_buf); 00131 00132 00133 00134 00135 00136 struct uip_ip6addr 00137 { 00138 NyLPC_TUInt16 v[8]; 00139 }PACK_STRUCT_END; 00140 00141 typedef struct uip_ip6addr uip_ip6addr_t; 00142 00143 NyLPC_TUInt16 NyLPC_uip_chksum(NyLPC_TUInt16 sum, const NyLPC_TUInt8 *data, NyLPC_TUInt16 len); 00144 00145 00146 00147 00148 /** 00149 * TTL 00150 */ 00151 #define UIP_DEFAULT_IP_TTL 64 00152 00153 00154 00155 /** 00156 * The maximum number of times a segment should be retransmitted 00157 * before the connection should be aborted. 00158 * 00159 * This should not be changed. 00160 */ 00161 #define UIP_MAXRTX 8 00162 00163 00164 00165 00166 00167 # if UIP_BYTE_ORDER == NyLPC_ENDIAN_BIG 00168 # define NyLPC_HTONS(n) (n) 00169 # define NyLPC_htons(n) (n) 00170 # define NyLPC_ntohs(n) (n) 00171 # define NyLPC_htonl(n) (n) 00172 # define NyLPC_ntohl(n) (n) 00173 # define NyLPC_HTONS(n) (n) 00174 # define NyLPC_NTOHS(n) (n) 00175 # else 00176 # define NyLPC_htonl(n) NyLPC_TUInt32_bswap(n) 00177 # define NyLPC_ntohl(n) NyLPC_TUInt32_bswap(n) 00178 # define NyLPC_htons(n) NyLPC_TUInt16_bswap(n) 00179 # define NyLPC_ntohs(n) NyLPC_TUInt16_bswap(n) 00180 # define NyLPC_HTONS(n) NyLPC_TUInt16_BSWAP(n) 00181 # define NyLPC_NTOHS(n) NyLPC_TUInt16_BSWAP(n) 00182 # define NyLPC_NTOHL(n) NyLPC_TUInt32_BSWAP(n) 00183 # define NyLPC_HTONL(n) NyLPC_TUInt32_BSWAP(n) 00184 #endif /* NyLPC_HTONS */ 00185 00186 00187 00188 00189 /********************************************************************** 00190 * 00191 * struct NyLPC_TEthernetIIHeader 00192 * 00193 **********************************************************************/ 00194 00195 #define NyLPC_TEthernetIIHeader_TYPE_IP 0x0800 00196 #define NyLPC_TEthernetIIHeader_TYPE_ARP 0x0806 00197 #define NyLPC_TEthernetIIHeader_TYPE_IPV6 0x86DD 00198 //#define UIP_ETHTYPE_IP 0x0800 00199 //#define UIP_ETHTYPE_ARP 0x0806 00200 //#define UIP_ETHTYPE_IP6 0x86dd 00201 00202 struct NyLPC_TEthernetIIHeader 00203 { 00204 struct NyLPC_TEthAddr dest; 00205 struct NyLPC_TEthAddr src; 00206 NyLPC_TUInt16 type; 00207 }PACK_STRUCT_END; 00208 00209 00210 NyLPC_TUInt16 NyLPC_TEthernetIIHeader_setArpTx( 00211 struct NyLPC_TEthernetIIHeader* i_struct, 00212 const struct NyLPC_TEthAddr* i_my_eth_addr); 00213 00214 NyLPC_TUInt16 NyLPC_TEthernetIIHeader_setIPv4Tx( 00215 struct NyLPC_TEthernetIIHeader* i_eth, 00216 const struct NyLPC_TEthAddr* i_src_eth_addr, 00217 const struct NyLPC_TEthAddr* i_dest_eth_addr); 00218 /********************************************************************** 00219 * 00220 * struct NyLPC_TIPv4Header 00221 * 00222 **********************************************************************/ 00223 00224 00225 /** 00226 * IPパケットヘッダのメモリマップ構造体 00227 * 値はすべてネットワークオーダーです。 00228 */ 00229 struct NyLPC_TIPv4Header 00230 { 00231 NyLPC_TUInt8 vhl; 00232 NyLPC_TUInt8 tos; 00233 NyLPC_TUInt16 len16; 00234 NyLPC_TUInt16 ipid16; 00235 NyLPC_TUInt16 ipoffset; 00236 NyLPC_TUInt8 ttl; 00237 NyLPC_TUInt8 proto; 00238 NyLPC_TUInt16 ipchksum; 00239 struct NyLPC_TIPv4Addr srcipaddr; 00240 struct NyLPC_TIPv4Addr destipaddr; 00241 }PACK_STRUCT_END; 00242 00243 00244 00245 00246 NyLPC_TBool NyLPC_TIPv4Header_isCorrectIpCheckSum( 00247 const struct NyLPC_TIPv4Header* ip_header); 00248 00249 NyLPC_TBool NyLPC_cIPv4Packet_isCorrectTcpCheckSum( 00250 const struct NyLPC_TIPv4Header* ip_header); 00251 00252 NyLPC_TUInt16 NyLPC_TIPv4Header_makeIpChecksum(const struct NyLPC_TIPv4Header* ip_header); 00253 00254 00255 NyLPC_TUInt16 NyLPC_TIPv4Header_makeTcpChecksum( 00256 const struct NyLPC_TIPv4Header* i_iph); 00257 00258 #define NyLPC_TIPv4Header_isDestAddrEqual(i_struct,i_addr) ((i_struct)->destipaddr==(i_addr)) 00259 #define NyLPC_TIPv4Header_isSrcAddrEqual(i_struct,i_addr) ((i_struct)->srcipaddr==(i_addr)) 00260 00261 /** 00262 * IPヘッダの長さを返す。 00263 */ 00264 #define NyLPC_TIPv4Header_getHeaderLength(i_iph) (((i_iph)->vhl & 0x0f)*4) 00265 /** 00266 * IPパケット全体の長さを返す。 00267 */ 00268 #define NyLPC_TIPv4Header_getPacketLength(i_iph) (NyLPC_ntohs((i_iph)->len16)) 00269 00270 /** 00271 * IPヘッダを送信パケット用に設定する。 00272 * ipid16にはコールされるたびに新しい値を設定する。 00273 * ipcecksumには0を設定する。 00274 * この関数は、パケットサイズ,ローカルIP/リモートIPの設定はしない。 00275 */ 00276 void NyLPC_TIPv4Header_writeTxIpHeader( 00277 struct NyLPC_TIPv4Header* i_struct, 00278 NyLPC_TUInt8 i_proto); 00279 /********************************************************************** 00280 * 00281 * struct NyLPC_TIPv6Header 00282 * 00283 **********************************************************************/ 00284 00285 00286 struct NyLPC_TIPv6Header 00287 { 00288 /* IPv6 header. */ 00289 NyLPC_TUInt8 vtc; 00290 NyLPC_TUInt8 tcflow; 00291 NyLPC_TUInt16 flow; 00292 NyLPC_TUInt8 len16; 00293 NyLPC_TUInt8 proto, ttl; 00294 uip_ip6addr_t srcipaddr; 00295 uip_ip6addr_t destipaddr; 00296 }PACK_STRUCT_END; 00297 00298 void NyLPC_TIPv6Header_setSendHeader( 00299 struct NyLPC_TIPv6Header* i_iph, 00300 uip_ip6addr_t i_src, 00301 uip_ip6addr_t i_dest, 00302 NyLPC_TUInt8 i_proto, 00303 NyLPC_TUInt8 i_ttl, 00304 NyLPC_TUInt16 i_len); 00305 00306 NyLPC_TUInt16 NyLPC_TIPv6Header_makeTcpChecksum( 00307 struct NyLPC_TIPv6Header* i_iph, 00308 NyLPC_TUInt16 i_len); 00309 00310 #define NyLPC_TIPv6Header_isDestAddrEqual(i_struct,i_addr) (memcmp((i_struct)->destipaddr,(i_addr),sizeof(uip_ip6addr_t))) 00311 #define NyLPC_TIPv6Header_isSrcAddrEqual(i_struct,i_addr) (memcmp(i_struct)->srcipaddr,(i_addr),sizeof(uip_ip6addr_t))) 00312 /********************************************************************** 00313 * 00314 * struct NyLPC_TTcpHeader 00315 * 00316 **********************************************************************/ 00317 #define TCP_OPT_END 0 /* End of TCP options list */ 00318 #define TCP_OPT_NOOP 1 /* "No-operation" TCP option */ 00319 #define TCP_OPT_MSS 2 /* Maximum segment size TCP option */ 00320 #define TCP_OPT_MSS_LEN 4 /* Length of TCP MSS option. */ 00321 00322 /** 00323 * TCP/IPヘッダのメモリマップ構造体 00324 * マルチバイトの値は、全てネットワークオーダーです。 00325 */ 00326 struct NyLPC_TTcpHeader 00327 { 00328 //TCP header. 00329 NyLPC_TUInt16 srcport; 00330 NyLPC_TUInt16 destport; 00331 NyLPC_TUInt32 seqno32; 00332 NyLPC_TUInt32 ackno32; 00333 NyLPC_TUInt8 tcpoffset; 00334 NyLPC_TUInt8 flags; 00335 NyLPC_TUInt16 wnd16; 00336 NyLPC_TUInt16 tcpchksum; 00337 NyLPC_TUInt8 urgp[2]; 00338 // NyLPC_TUInt8 optdata[4]; 00339 } PACK_STRUCT_END; 00340 00341 00342 NyLPC_TUInt8* NyLPC_TTcpHeader_getTcpOptFragmentPtr( 00343 const struct NyLPC_TTcpHeader* i_struct, 00344 NyLPC_TUInt8 i_opt_id); 00345 00346 NyLPC_TBool NyLPC_TTcpHeader_getTcpMmsOpt( 00347 const struct NyLPC_TTcpHeader* i_struct,NyLPC_TUInt16* o_val); 00348 00349 /** 00350 * この関数は、TCPヘッダの長さを返します。ヘッダの長さは、i_structのフィールドから計算します。 00351 * @param i_struct 00352 * 構造体のアドレス。 00353 */ 00354 NyLPC_TUInt16 NyLPC_TTcpHeader_getHeaderLength(const struct NyLPC_TTcpHeader* i_struct); 00355 00356 /** 00357 * この関数は、指定したアドレスに、mmsオプション値を書き込みます。 00358 */ 00359 void NyLPC_TTcpHeader_setMmsOpt(NyLPC_TUInt8* i_opt_addr,NyLPC_TUInt16 i_mms); 00360 00361 00362 /********************************************************************** 00363 * 00364 * struct NyLPC_TUdpHeader 00365 * 00366 **********************************************************************/ 00367 00368 /** 00369 * UDP/IPヘッダのメモリマップ構造体 00370 */ 00371 struct NyLPC_TUdpHeader 00372 { 00373 NyLPC_TUInt16 srcport; 00374 NyLPC_TUInt16 destport; 00375 NyLPC_TUInt16 udplen; 00376 NyLPC_TUInt16 udpchksum; 00377 } PACK_STRUCT_END; 00378 00379 /** 00380 * UDPヘッダの長さを返す。 00381 */ 00382 #define NyLPC_TUdpHeader_getHeaderLength(i_struct) (8) 00383 00384 /********************************************************************** 00385 * 00386 * struct NyLPC_TIcmpipHeader 00387 * 00388 **********************************************************************/ 00389 00390 00391 struct NyLPC_TIcmpHeader 00392 { 00393 /* ICMP (echo) header. */ 00394 NyLPC_TUInt8 type, icode; 00395 NyLPC_TUInt16 icmpchksum; 00396 #if !UIP_CONF_IPV6 00397 NyLPC_TUInt16 id, seqno; 00398 #else /* !UIP_CONF_IPV6 */ 00399 NyLPC_TUInt8 flags, reserved1, reserved2, reserved3; 00400 NyLPC_TUInt8 icmp6data[16]; 00401 NyLPC_TUInt8 options[1]; 00402 #endif /* !UIP_CONF_IPV6 */ 00403 } PACK_STRUCT_END; 00404 00405 /********************************************************************** 00406 * 00407 * struct NyLPC_TIcmpipHeader 00408 * 00409 **********************************************************************/ 00410 00411 struct NyLPC_TArpHeader 00412 { 00413 NyLPC_TUInt16 hwtype; 00414 NyLPC_TUInt16 protocol; 00415 NyLPC_TUInt8 hwlen; 00416 NyLPC_TUInt8 protolen; 00417 NyLPC_TUInt16 opcode; 00418 struct NyLPC_TEthAddr shwaddr; 00419 struct NyLPC_TIPv4Addr sipaddr; 00420 struct NyLPC_TEthAddr dhwaddr; 00421 struct NyLPC_TIPv4Addr dipaddr; 00422 } PACK_STRUCT_END; 00423 00424 00425 /** 00426 * i_req_addrを問い合わせるARP_REQUESTを生成します。 00427 */ 00428 void NyLPC_TArpHeader_setArpRequest( 00429 struct NyLPC_TArpHeader* i_struct, 00430 const struct NyLPC_TIPv4Addr i_saddr, 00431 const struct NyLPC_TEthAddr* i_srceth, 00432 const struct NyLPC_TIPv4Addr* i_req_addr); 00433 00434 00435 typedef struct NyLPC_TcEthernetIIPayload NyLPC_TcEthernetIIPayload_t; 00436 00437 00438 00439 00440 /********************************************************************** 00441 * 00442 * NyLPC_TIPv6Payload 00443 * 00444 **********************************************************************/ 00445 00446 00447 struct NyLPC_TIPv6Payload 00448 { 00449 struct NyLPC_TIPv6Header* header; 00450 union{ 00451 void* rawbuf; 00452 void* tcp; 00453 void* udp; 00454 void* icmp6; 00455 }payload; 00456 }; 00457 00458 struct NyLPC_TcEthernetIIPayload 00459 { 00460 struct NyLPC_TEthernetIIHeader* header; 00461 union{ 00462 void* rawbuf; 00463 struct NyLPC_TArpHeader* arp; 00464 struct NyLPC_TIPv4Payload* ipv4; 00465 struct NyLPC_TIPv6Payload* ipv6; 00466 }payload; 00467 NyLPC_TUInt16 len; 00468 }; 00469 00470 00471 00472 00473 00474 #ifdef __cplusplus 00475 } 00476 #endif /* __cplusplus */ 00477 00478 00479 #endif 00480
Generated on Tue Jul 12 2022 15:46:17 by
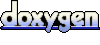