This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NyLPC_IEthernetDevice.h
00001 /* 00002 * NyLPC_IEthernetDevice.h 00003 * 00004 * Created: 2011/12/06 00005 * MiMicのイーサネットドライバインタフェイスを定義する。 00006 */ 00007 #ifndef NyLPC_IEthernetDevice_h 00008 #define NyLPC_IEthernetDevice_h 00009 #include "NyLPC_stdlib.h" 00010 #include "../../netif/NyLPC_NetIf_ip_types.h" 00011 00012 00013 #ifdef __cplusplus 00014 extern "C" { 00015 #endif /* __cplusplus */ 00016 00017 00018 00019 typedef struct NyLPC_TiEthernetDevice NyLPC_TiEthernetDevice_t; 00020 00021 /**非同期イベントのメッセージタイプ*/ 00022 typedef unsigned int NyLPC_TiEthernetDevice_EVENT; 00023 /**TXが到達した*/ 00024 #define NyLPC_TiEthernetDevice_EVENT_ON_TX 1 00025 /**RXが到達した*/ 00026 #define NyLPC_TiEthernetDevice_EVENT_ON_RX 2 00027 00028 /** 00029 * ヒント値。NyLPC_TcEthernetMM_HINT_CTRL_PACKETと同じ。 00030 */ 00031 #define NyLPC_TcEthernetMM_HINT_CTRL_PACKET 0 00032 00033 typedef void (*NyLPC_TiEthernetDevice_onEvent)(void* i_param,NyLPC_TiEthernetDevice_EVENT i_type); 00034 00035 00036 00037 /** 00038 * 受信キューの先頭にあるデータを返す。 00039 * 関数は、受信キューのポインタを操作しない。続けて読み出したとしても、同じポインターを返す。 00040 * 次のデータを得るには、nextRxEthFrameを呼び出す。 00041 * #制限として、返却したポインタの内容は、一時的に書き換え可としてください。(この制限は将来削除します。) 00042 * @return 00043 * 成功した場合、受信データを格納したバッファポインタ。返却値は、nextRxEthFrameをコールするまで有効である。 00044 * 存在しない場合NULL。 00045 */ 00046 #define NyLPC_iEthernetDevice_getRxEthFrame(i,p) (i)->getRxEthFrame(p) 00047 typedef void* (*NyLPC_TiEthernetDevice_getRxEthFrame)(unsigned short* o_len_of_data); 00048 00049 00050 00051 /** 00052 * getRxEthFrameで得たメモリを破棄して、次のRXデータを準備する。 00053 * getRxEthFrameで返したメモリの内容の有効期間はここで終了するので注意すること。 00054 */ 00055 #define NyLPC_iEthernetDevice_nextRxEthFrame(i) (i)->nextRxEthFrame() 00056 typedef void (*NyLPC_TiEthernetDevice_nextRxEthFrame)(void); 00057 00058 00059 /** 00060 * 送信バッファを得る。 00061 * 関数は、i_hintで示されるサイズのメモリブロックを確保しようとするが、実際にはそれよりも小さいことがある。 00062 * @param i_hint 00063 * 確保してほしいメモリサイズ 00064 * @param o_size 00065 * 実際に割り当てたメモリのサイズ 00066 * @return 00067 * 割り当てたメモリブロックのヘッダ。 00068 */ 00069 #define NyLPC_iEthernetDevice_allocTxBuf(i,h,s) (i)->allocTxBuf((h),(s)) 00070 typedef void* (*NyLPC_TiEthernetDevice_allocTxBuf)(NyLPC_TUInt16 i_hint,NyLPC_TUInt16* o_size); 00071 00072 00073 /** 00074 * alloc_TxBufで得た送信バッファを開放する。 00075 * 関数は、メモリブロックの参照カウンタを1減算する。 00076 */ 00077 #define NyLPC_iEthernetDevice_releaseTxBuf(i,b) (i)->releaseTxBuf(b) 00078 typedef void (*NyLPC_TiEthernetDevice_releaseTxBuf)(void* i_buf); 00079 00080 00081 00082 /** 00083 * イーサネットパケット構造体i_bufの内容を送信する。 00084 * @param i_buf 00085 * allocTxBufで得たメモリか、初期化したNyLPC_TTxBufferHeaderメモリブロックを指定する。 00086 * 送信が終わるまでの間、メモリを開放してはならない。 00087 * @oaram i_size 00088 * i_bufの後ろに連結されているデータメモリの長さ 00089 */ 00090 #define NyLPC_iEthernetDevice_sendTxEthFrame(i,b,s) (i)->sendTxEthFrame((b),(s)) 00091 typedef void (*NyLPC_TiEthernetDevice_sendTxEthFrame)(void* i_buf,unsigned short i_size); 00092 00093 00094 /** 00095 * この関数は、送信キューの状態を進行させるタイミングを与える。 00096 * 外部関数が、送信キューにセットしたパケットの送信待ちをするときに呼び出す。 00097 */ 00098 #define NyLPC_iEthernetDevice_processTx(i) (i)->processTx() 00099 typedef void (*NyLPC_TiEthernetDevice_processTx)(void); 00100 00101 00102 00103 /** 00104 * この関数は、ドライバを開始します。 00105 * @param i_eth_addr 00106 * イーサネットアドレス 00107 * @param i_handler 00108 * 通知ハンドラ 00109 * @param i_param 00110 * 通知ハンドラに渡るパラメータ 00111 */ 00112 #define NyLPC_iEthernetDevice_start(i,a,h,p) (i)->start((a),(h),(p)) 00113 typedef NyLPC_TBool(*NyLPC_TiEthernetDevice_start)(const struct NyLPC_TEthAddr* i_eth_addr,NyLPC_TiEthernetDevice_onEvent i_handler,void* i_param); 00114 00115 00116 /** 00117 * この関数はドライバを停止します。 00118 */ 00119 #define NyLPC_iEthernetDevice_stop(i) (i)->stop() 00120 typedef void(*NyLPC_TiEthernetDevice_stop)(void); 00121 00122 00123 /** 00124 * デバイス名を返します。 00125 */ 00126 #define NyLPC_iEthernetDevice_getDevicName(i) ((i)->_device_name) 00127 00128 /** 00129 * イーサネットデバイスのアクセスインターフェイス 00130 * イーサネットデバイスの管理するメモリブロック、ペリフェラル制御のインタフェイスを提供する。 00131 * 00132 */ 00133 struct TiEthernetDevice 00134 { 00135 /** NyLPC_iEthernetDevice_getDevicNameで返却する値*/ 00136 const char* _device_name;//pointer to device name. 00137 NyLPC_TiEthernetDevice_start start; 00138 NyLPC_TiEthernetDevice_stop stop; 00139 NyLPC_TiEthernetDevice_getRxEthFrame getRxEthFrame; 00140 NyLPC_TiEthernetDevice_nextRxEthFrame nextRxEthFrame; //nextRxEthFrame; 00141 NyLPC_TiEthernetDevice_allocTxBuf allocTxBuf; 00142 NyLPC_TiEthernetDevice_releaseTxBuf releaseTxBuf; 00143 NyLPC_TiEthernetDevice_sendTxEthFrame sendTxEthFrame; 00144 NyLPC_TiEthernetDevice_processTx processTx; 00145 }; 00146 00147 00148 00149 00150 #ifdef __cplusplus 00151 } 00152 #endif /* __cplusplus */ 00153 00154 #endif
Generated on Tue Jul 12 2022 15:46:17 by
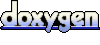