This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
NetConfig.cpp
00001 #include "NetConfig.h" 00002 #include "NyLPC_flash.h" 00003 #include "NyLPC_netif.h" 00004 #include "utils/PlatformInfo.h" 00005 #include <stdio.h> 00006 #include <stdlib.h> 00007 #include "mbed.h" 00008 00009 /** 00010 * Default UPnP Description 00011 */ 00012 const static char* UPNP_DEVICE_TYPE ="urn:schemas-upnp-org:device:Basic:1"; 00013 const static char* UPNP_DEFAULT_FRENDLY_NAME="MiMic Device"; 00014 const static char* UPNP_DEFAULT_MANUFACTUR_NAME="nyatla.jp"; 00015 const static char* UPNP_DEFAULT_MANUFACTUR_URL="http://nyatla.jp/"; 00016 const static char* UPNP_DEFAULT_MODEL_NAME="MiMic UPnP device."; 00017 const static char* STR_EMPTY=""; 00018 const static char* UPNP_DEFAULT_PRESENTATION_URL="/"; 00019 const static char* UPNP_DEFAULT_ICON_MIMETYPE="image/png"; 00020 const static char* UPNP_DEFAULT_ICON_URL="./icon.png"; 00021 00022 00023 /* 00024 setUPnPFriendlyName 00025 setUPnPManufactur(name,url,description) 00026 setUPnPModel(name,number,url) 00027 setUPnPPresentationUrl(name,number,url) 00028 */ 00029 00030 00031 //default mdns records 00032 const static char* MDNS_SRV_HTTP="_http._tcp\0"; 00033 //const static char* MDNS_NAME="LPC176x(MiMic)\0"; 00034 00035 extern "C" void mbed_mac_address(char *s); 00036 00037 00038 static void overrideMacAddrIfmbed(NyLPC_TcNetConfig& v) 00039 { 00040 mbed_mac_address((char*)(v.super.eth_mac.addr)); 00041 //update default hostname 00042 strcpy(v.hostname,"MiMic"); 00043 NyLPC_uitoa2(NyLPC_TcIPv4Config_getEtherMac000120203(&(v.super)),v.hostname+ 5,16,8); 00044 NyLPC_uitoa2((NyLPC_TcIPv4Config_getEtherMac0405xxxx(&(v.super))>>16)&0xffff,v.hostname+13,16,4); 00045 v.services.flags=NyLPC_TcNetConfig_SERVICE_FLAG_MDNS|NyLPC_TcNetConfig_SERVICE_FLAG_UPNP; 00046 } 00047 00048 static void updateOnchipConfig(NyLPC_TcNetConfig& v) 00049 { 00050 struct NyLPC_TMiMicConfigulation cfg_image; 00051 //パラメータ→ROMイメージ変換 00052 cfg_image.fast_boot=0xffffffff; 00053 cfg_image.mac_00_01_02_03=NyLPC_TcIPv4Config_getEtherMac000120203(&(v.super)); 00054 cfg_image.mac_04_05_xx_xx=NyLPC_TcIPv4Config_getEtherMac0405xxxx(&(v.super)); 00055 cfg_image.ipv4_addr_net =NyLPC_ntohl(v.super.ip_addr.v); 00056 cfg_image.ipv4_mask_net =NyLPC_ntohl(v.super.netmask.v); 00057 cfg_image.ipv4_drut_net =NyLPC_ntohl(v.super.dr_addr.v); 00058 //additional information 00059 cfg_image.ipv4_flags=v.tcp_mode; 00060 cfg_image.http_port=v.services.http_port; 00061 cfg_image.srv_flags=v.services.flags; 00062 strcpy(cfg_image.hostname,v.hostname); 00063 //FreeRTOSの停止 00064 NyLPC_cIsr_enterCritical(); 00065 //Flashへの書き込み 00066 NyLPC_cMiMicConfiglation_updateConfigulation(&cfg_image); 00067 //FreeRTOSの復帰 00068 NyLPC_cIsr_exitCritical(); 00069 } 00070 00071 00072 namespace MiMic 00073 { 00074 NetConfig::NetConfig(bool i_is_factory_default) 00075 { 00076 NyLPC_cNetConfig_initialize(&(this->_inst),i_is_factory_default); 00077 this->_ref_custom_dns_record=NULL; 00078 this->_ref_custom_upnp_desc=NULL; 00079 00080 //check mbed 00081 if(PlatformInfo::getPlatformType()==PlatformInfo::PF_MBED) { 00082 //mbedチップからMACアドレスを取得 00083 if(!NyLPC_cMiMicConfiglation_hasUserConfigulation()) { 00084 //is 1st read? 00085 //mbed override 00086 overrideMacAddrIfmbed((this->_inst)); 00087 //save 00088 updateOnchipConfig((this->_inst)); 00089 } else { 00090 //2nd read 00091 if(i_is_factory_default) { 00092 //mbed override 00093 overrideMacAddrIfmbed((this->_inst)); 00094 } else { 00095 //nothing to do 00096 } 00097 } 00098 } 00099 00100 //updateUUID 00101 this->setUPnPUdn(0xe29f7100,0x4ba2,0x01e0,0); 00102 this->_upnp_desc.device_type=UPNP_DEVICE_TYPE; 00103 this->_upnp_desc.frendly_name=UPNP_DEFAULT_FRENDLY_NAME; 00104 this->_upnp_desc.manufacturer=UPNP_DEFAULT_MANUFACTUR_NAME; 00105 this->_upnp_desc.manufacturer_url=UPNP_DEFAULT_MANUFACTUR_URL; 00106 this->_upnp_desc.model_descriprion=STR_EMPTY; 00107 this->_upnp_desc.model_name=UPNP_DEFAULT_MODEL_NAME; 00108 this->_upnp_desc.model_number=NULL; 00109 this->_upnp_desc.model_url=NULL; 00110 this->_upnp_desc.serial_number=STR_EMPTY; 00111 this->_upnp_desc.udn=this->_udn;//pointer 00112 this->_upnp_desc.upc=NULL; 00113 this->_upnp_icon.width=32; 00114 this->_upnp_icon.height=32; 00115 this->_upnp_icon.depth=8; 00116 this->_upnp_icon.mimetype=UPNP_DEFAULT_ICON_MIMETYPE; 00117 this->_upnp_icon.url=UPNP_DEFAULT_ICON_URL; 00118 this->_upnp_desc.icons=&this->_upnp_icon;//pointer 00119 this->_upnp_desc.presentation_url=UPNP_DEFAULT_PRESENTATION_URL; 00120 this->_upnp_desc.number_of_devices=0; 00121 this->_upnp_desc.number_of_service=0; 00122 this->_upnp_desc.number_of_icon=1; 00123 this->_upnp_desc.devices=NULL; 00124 this->_upnp_desc.services=NULL; 00125 //mdns 00126 this->_dns_record.name=UPNP_DEFAULT_FRENDLY_NAME; 00127 this->_dns_record.a=this->_inst.hostname;//pointer 00128 this->_dns_record.num_of_srv=1; 00129 this->_srv_record.protocol=MDNS_SRV_HTTP; 00130 this->_srv_record.port=this->_inst.services.http_port; 00131 this->_dns_record.srv=&(this->_srv_record); 00132 00133 } 00134 00135 NetConfig::~NetConfig() 00136 { 00137 NyLPC_cNetConfig_finalize(&(this->_inst)); 00138 } 00139 00140 const struct NyLPC_TUPnPDevDescDevice* NetConfig::refUPnPDevDesc()const 00141 { 00142 return this->_ref_custom_upnp_desc!=NULL?this->_ref_custom_upnp_desc:&this->_upnp_desc; 00143 } 00144 /** internal dns record*/ 00145 const struct NyLPC_TDnsRecord* NetConfig::refMdnsRecord()const 00146 { 00147 return this->_ref_custom_dns_record!=NULL?this->_ref_custom_dns_record:&this->_dns_record; 00148 } 00149 00150 00151 void NetConfig::setZeroconf(bool v) 00152 { 00153 this->_inst.tcp_mode=(v?NyLPC_TcNetConfig_IPV4_FLAG_MODE_APIPA:NyLPC_TcNetConfig_IPV4_FLAG_MODE_MANUAL); 00154 } 00155 /** 00156 * Set IPv4 ip address to instance. 00157 */ 00158 void NetConfig::setIpAddr(unsigned char ip1,unsigned char ip2,unsigned char ip3,unsigned char ip4) 00159 { 00160 NyLPC_TIPv4Addr_set(&(this->_inst.super.ip_addr),ip1,ip2,ip3,ip4); 00161 } 00162 00163 void NetConfig::setIpAddr(const IpAddr& i_addr) 00164 { 00165 this->_inst.super.ip_addr=i_addr.addr.v4; 00166 } 00167 00168 /** 00169 * Set IPv4 network mask value to instance. 00170 */ 00171 void NetConfig::setNetMask(unsigned char ip1,unsigned char ip2,unsigned char ip3,unsigned char ip4) 00172 { 00173 NyLPC_TIPv4Addr_set(&(this->_inst.super.netmask),ip1,ip2,ip3,ip4); 00174 } 00175 void NetConfig::setNetMask(const IpAddr& i_mask) 00176 { 00177 this->_inst.super.netmask=i_mask.addr.v4; 00178 } 00179 00180 /** 00181 * Set IPv4 default gateway address to instance. 00182 */ 00183 void NetConfig::setGateway(unsigned char ip1,unsigned char ip2,unsigned char ip3,unsigned char ip4) 00184 { 00185 NyLPC_TIPv4Addr_set(&(this->_inst.super.dr_addr),ip1,ip2,ip3,ip4); 00186 } 00187 void NetConfig::setGateway(const IpAddr& i_addr) 00188 { 00189 this->_inst.super.dr_addr=i_addr.addr.v4; 00190 } 00191 00192 /** 00193 * Set ethernet mac address to instance. 00194 */ 00195 void NetConfig::setEmac(unsigned char a1,unsigned char a2,unsigned char a3,unsigned char a4,unsigned char a5,unsigned char a6) 00196 { 00197 NyLPC_TEthAddr_set(&(this->_inst.super.eth_mac),a1,a2,a3,a4,a5,a6); 00198 //update only node field 00199 for(NyLPC_TInt16 i=0;i<6;i++){ 00200 NyLPC_uitoa2(this->_inst.super.eth_mac.addr[i],&this->_udn[5+24+i*2],16,2); 00201 } 00202 00203 } 00204 void NetConfig::setSrvHttpPort(unsigned short port) 00205 { 00206 this->_srv_record.port=this->_inst.services.http_port=port; 00207 } 00208 void NetConfig::setSrvMdns(bool i_enable) 00209 { 00210 if(i_enable) { 00211 this->_inst.services.flags|=NyLPC_TcNetConfig_SERVICE_FLAG_MDNS; 00212 } else { 00213 this->_inst.services.flags&=(~NyLPC_TcNetConfig_SERVICE_FLAG_MDNS); 00214 } 00215 } 00216 void NetConfig::setSrvUPnP(bool i_enable) 00217 { 00218 if(i_enable) { 00219 this->_inst.services.flags|=NyLPC_TcNetConfig_SERVICE_FLAG_UPNP; 00220 } else { 00221 this->_inst.services.flags&=(~NyLPC_TcNetConfig_SERVICE_FLAG_UPNP); 00222 } 00223 } 00224 00225 void NetConfig::setHostName(const char* i_hostname) 00226 { 00227 this->setHostName(i_hostname,strlen(i_hostname)); 00228 } 00229 void NetConfig::setHostName(const char* i_hostname,int len) 00230 { 00231 int l=(len>(NyLPC_TcNetConfig_HOSTNAME_LEN-1))?NyLPC_TcNetConfig_HOSTNAME_LEN-1:len; 00232 memcpy(this->_inst.hostname,i_hostname,l); 00233 *(this->_inst.hostname+l)='\0'; 00234 } 00235 const char* NetConfig::getHostName() 00236 { 00237 return this->_inst.hostname; 00238 } 00239 00240 void NetConfig::setFriendlyName(const char* i_name) 00241 { 00242 this->_dns_record.name=this->_upnp_desc.frendly_name=(i_name==NULL?STR_EMPTY:i_name); 00243 } 00244 void NetConfig::setUPnPManufactur(const char* i_name,const char* i_url) 00245 { 00246 this->_upnp_desc.manufacturer=i_name==NULL?STR_EMPTY:i_name; 00247 this->_upnp_desc.manufacturer_url=i_name==NULL?STR_EMPTY:i_url; 00248 } 00249 void NetConfig::setUPnPModel(const char* i_name,const char* i_number,const char* i_url,const char* i_description) 00250 { 00251 this->_upnp_desc.model_descriprion=i_description==NULL?STR_EMPTY:i_description; 00252 this->_upnp_desc.model_name=i_name==NULL?STR_EMPTY:i_name; 00253 this->_upnp_desc.model_number=i_number; 00254 this->_upnp_desc.model_url=i_url; 00255 } 00256 void NetConfig::setUPnPSerialNumber(const char* i_number) 00257 { 00258 this->_upnp_desc.serial_number=i_number; 00259 } 00260 void NetConfig::setUPnPUdn(unsigned long i_time_l,unsigned short i_time_m,unsigned short i_time_h,unsigned short i_sq) 00261 { 00262 NyLPC_TcUuid_t uuid; 00263 NyLPC_cUuid_initialize(&uuid); 00264 NyLPC_cUuid_setTimeBase(&uuid,i_time_l,(i_time_h<<16)|i_time_m,i_sq,&(this->_inst.super.eth_mac)); 00265 strcpy(this->_udn,"uuid:"); 00266 NyLPC_cUuid_toString(&uuid,this->_udn+5); 00267 NyLPC_cUuid_finalize(&uuid); 00268 } 00269 void NetConfig::setUPnPIcon(unsigned short i_width,unsigned short i_height,unsigned short i_depth,const char* i_mimetype,const char* i_url) 00270 { 00271 this->_upnp_icon.width=i_width; 00272 this->_upnp_icon.height=i_height; 00273 this->_upnp_icon.depth=i_depth; 00274 this->_upnp_icon.mimetype=i_mimetype; 00275 this->_upnp_icon.url=i_url; 00276 } 00277 00278 void NetConfig::setUPnPPresentationURL(const char* i_url) 00279 { 00280 this->_upnp_desc.presentation_url=i_url; 00281 } 00282 void NetConfig::setCustomUPnPDescription(const struct NyLPC_TUPnPDevDescDevice* i_ref_description) 00283 { 00284 this->_ref_custom_upnp_desc=i_ref_description; 00285 } 00286 void NetConfig::setCustomMdnsRecord(const struct NyLPC_TDnsRecord* i_ref_record) 00287 { 00288 this->_ref_custom_dns_record=i_ref_record; 00289 } 00290 00291 bool NetConfig::loadFromFile(const char* i_file) 00292 { 00293 #define NUMBER_OF_NAME 8 00294 const static char* tbl[]= { 00295 "macaddr", //0 00296 "ipaddr", //1 00297 "netmask", //2 00298 "gateway", //3 00299 "srv_http_port",//4 00300 "srv_mdns",//5 00301 "srv_upnp" //6 00302 "host",//7 00303 }; 00304 char tmp[32]; 00305 union { 00306 unsigned char u8[6]; 00307 NyLPC_TUInt32 u32; 00308 } v; 00309 const char* p;//pointer to read 00310 const char* key; 00311 const char* t; 00312 int l; 00313 FILE* fp = fopen(i_file,"r"); 00314 if(fp==NULL) { 00315 return false; 00316 } 00317 //read from values 00318 while(fgets(tmp,31,fp)) { 00319 p=NyLPC_cFormatTextReader_seekSpace(tmp)+tmp;//skip space 00320 l=NyLPC_cFormatTextReader_readWord(p,&key); 00321 for(int i=0; i<NUMBER_OF_NAME; i++) { 00322 if(l>=0 && NyLPC_strnicmp(key,tbl[i],l)==0) { 00323 p+=l;//skip keyname 00324 p=NyLPC_cFormatTextReader_seekSpace(p)+p;//skip space 00325 if(*p!='=') { 00326 break;//check equal 00327 } 00328 p++; 00329 //skip space 00330 p=NyLPC_cFormatTextReader_seekSpace(p)+p;//skip space 00331 switch(i) { 00332 case 0://macaddr 00333 if(NyLPC_cFormatTextReader_readMacAddr(p,v.u8)!=0) { 00334 this->setEmac(v.u8[0],v.u8[1],v.u8[2],v.u8[3],v.u8[4],v.u8[5]); 00335 } 00336 break; 00337 case 1://ipaddr 00338 if(NyLPC_cFormatTextReader_readIpAddr(p,v.u8)!=0) { 00339 this->setIpAddr(v.u8[0],v.u8[1],v.u8[2],v.u8[3]); 00340 this->setZeroconf(false); 00341 } else { 00342 if(NyLPC_cFormatTextReader_readWord(p,&t)==4) { 00343 if(NyLPC_strnicmp(t,"auto",4)==0) { 00344 this->setZeroconf(true); 00345 } 00346 } 00347 } 00348 break; 00349 case 2: 00350 if(NyLPC_cFormatTextReader_readIpAddr(p,v.u8)!=0) { 00351 this->setNetMask(v.u8[0],v.u8[1],v.u8[2],v.u8[3]); 00352 } 00353 break; 00354 case 3: 00355 if(NyLPC_cFormatTextReader_readIpAddr(p,v.u8)!=0) { 00356 this->setGateway(v.u8[0],v.u8[1],v.u8[2],v.u8[3]); 00357 } 00358 break; 00359 case 4: 00360 if(NyLPC_cFormatTextReader_readUInt(p,&(v.u32))!=0) { 00361 this->setSrvHttpPort((unsigned short)v.u32); 00362 } 00363 break; 00364 case 5: 00365 l=NyLPC_cFormatTextReader_readWord(p,&t); 00366 if((*t=='y' || *t=='Y')) { 00367 this->setSrvMdns(true); 00368 } else if((*t=='n' || *t=='N')) { 00369 this->setSrvMdns(false); 00370 } 00371 break; 00372 case 6: 00373 l=NyLPC_cFormatTextReader_readWord(p,&t); 00374 if((*t=='y' || *t=='Y')) { 00375 this->setSrvUPnP(true); 00376 } else if((*t=='n' || *t=='N')) { 00377 this->setSrvUPnP(false); 00378 } 00379 break; 00380 case 7: 00381 l=NyLPC_cFormatTextReader_readWord(p,&t); 00382 if(l>1) { 00383 this->setHostName(t,l); 00384 } 00385 break; 00386 default: 00387 break; 00388 } 00389 } 00390 } 00391 } 00392 fclose(fp); 00393 return true; 00394 } 00395 };
Generated on Tue Jul 12 2022 15:46:15 by
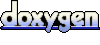