This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
ModWebSocket.h
00001 #pragma once 00002 #include "NyLPC_net.h" 00003 #include "ModBaseClass.h" 00004 #include "HttpdConnection.h" 00005 #include "Httpd.h" 00006 #include "Net.h" 00007 00008 namespace MiMic 00009 { 00010 class HttpdConnection; 00011 00012 /** 00013 * This class is Websocket module. 00014 * The class provides 3 services. 00015 * <ul> 00016 * <li>d.xml - a device description.</li> 00017 * <li>control/xx - soap handler</li> 00018 * <li>event/xx -event handler.</li> 00019 * </ul> 00020 */ 00021 class ModWebSocket:ModBaseClass 00022 { 00023 private: 00024 protected: 00025 NyLPC_TcModWebSocket_t* _mod; 00026 public: 00027 ModWebSocket(); 00028 ModWebSocket(const char* i_path); 00029 virtual ~ModWebSocket(); 00030 void setParam(const char* i_path); 00031 bool isStarted(); 00032 /** 00033 * This function executes websocket negotiation to the current connection. 00034 * Should be handle websocket session if function successful. 00035 * @return 00036 * true if negotiation successful;otherwishe false. 00037 */ 00038 bool execute(HttpdConnection& i_connection); 00039 /** 00040 * Write data to websocket stream. 00041 * @return 00042 * true if successful;otherwishe false and connection closed. 00043 */ 00044 bool write(const void* i_tx_buf,int i_tx_size); 00045 /** 00046 * This function sends data to websocket stream. 00047 * @param i_fmt 00048 * printf like format text. 00049 * @param ... 00050 * argument list for i_fmt 00051 */ 00052 bool writeFormat(const char* i_fmt,...); 00053 00054 /** 00055 * This function receives data from websocket stream. 00056 * @return 00057 * <ul> 00058 * <li>r<0 Error. The socket already closed. 00059 * <li>r==0 Timeout. The connection is continued. 00060 * <li>r>0 Success. Received data size. 00061 * </ul> 00062 * If an Error found, application should be call close and exit handler. 00063 */ 00064 int read(void* i_rx_buf,int i_rx_size); 00065 /** 00066 * This function terminates websocket connection. 00067 * Should be called if execute function successful. 00068 */ 00069 void close(); 00070 /** 00071 * This function returns read function status. 00072 * This is to confirm that "read" can call without blocking. 00073 * @return 00074 * true if can be call "read" with no-wait. otherwise, "read" might be wait. 00075 */ 00076 bool canRead(); 00077 00078 00079 }; 00080 }
Generated on Tue Jul 12 2022 15:46:15 by
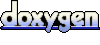