This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
ModFileIo.cpp
00001 #include <ModFileIo.h> 00002 #include <stdio.h> 00003 #include "mbed.h" 00004 using namespace MiMic; 00005 /* 00006 * FileIO class 00007 */ 00008 typedef struct TcModFileIoDumy 00009 { 00010 NyLPC_TcModFileIoBaseClass_t super; 00011 }TcFileIoDumy_t; 00012 00013 const static char* STR_TEXT_HTML="text/html"; 00014 #define SIZE_OF_FBUF 256 00015 00016 00017 00018 static NyLPC_TBool upload_handler(NyLPC_TcHttpdConnection_t* i_connection,const NyLPC_TChar* i_fname,NyLPC_TcHttpBodyParser_t* i_body_parser) 00019 { 00020 char* buf=Httpd::_shared_buf;// This handler called with lock! 00021 00022 //save to new file 00023 FILE *fp; 00024 fp = fopen(i_fname, "w"); 00025 if(fp==NULL){ 00026 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,500); 00027 return NyLPC_TBool_FALSE; 00028 } 00029 NyLPC_TInt16 l; 00030 if(NyLPC_cHttpBodyParser_parseStream(i_body_parser,NyLPC_cHttpdConnection_refStream(i_connection),buf,SIZE_OF_FBUF,&l)){ 00031 while(l>0){ 00032 fwrite(buf,1,l,fp); 00033 if(!NyLPC_cHttpBodyParser_parseStream(i_body_parser,NyLPC_cHttpdConnection_refStream(i_connection),buf,SIZE_OF_FBUF,&l)){ 00034 NyLPC_OnErrorGoto(Error_FILE); 00035 } 00036 } 00037 } 00038 fclose(fp); 00039 //write response 00040 NyLPC_cHttpdConnection_sendResponseHeader2(i_connection,200,STR_TEXT_HTML,0,NULL); 00041 return NyLPC_TBool_TRUE; 00042 Error_FILE: 00043 fclose(fp); 00044 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,500); 00045 return NyLPC_TBool_FALSE; 00046 } 00047 00048 static NyLPC_TBool create_handler(NyLPC_TcHttpdConnection_t* i_connection,const NyLPC_TChar* i_fname) 00049 { 00050 FILE *fp; 00051 fp = fopen(i_fname, "w"); 00052 if(fp!=NULL){ 00053 fclose(fp); 00054 NyLPC_cHttpdConnection_sendResponseHeader2(i_connection,200,STR_TEXT_HTML,0,NULL); 00055 return NyLPC_TBool_TRUE; 00056 } 00057 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,500); 00058 return NyLPC_TBool_FALSE; 00059 } 00060 00061 static NyLPC_TBool remove_handler(NyLPC_TcHttpdConnection_t* i_connection,const NyLPC_TChar* i_fname) 00062 { 00063 if(remove(i_fname)==0){ 00064 NyLPC_cHttpdConnection_sendResponseHeader2(i_connection,200,STR_TEXT_HTML,0,NULL); 00065 return NyLPC_TBool_TRUE; 00066 } 00067 //500ERROR 00068 NyLPC_cHttpdUtils_sendErrorResponse(i_connection,500); 00069 return NyLPC_TBool_FALSE; 00070 } 00071 00072 00073 00074 static void cModFileIoDumy_initialize(TcFileIoDumy_t* i_inst,const char* i_root_path) 00075 { 00076 NyLPC_cModFileIoBaseClass_initialize(&(i_inst->super),i_root_path); 00077 i_inst->super._abstruct_function.upload=upload_handler; 00078 i_inst->super._abstruct_function.create=create_handler; 00079 i_inst->super._abstruct_function.remove=remove_handler; 00080 } 00081 #define cModFileIoDumy_finalize(i_inst) NyLPC_cModFileIoBaseClass_finalize(&(i_inst)->super) 00082 #define cModFileIoDumy_canHandle(i_inst,i_connection) NyLPC_cModFileIoBaseClass_canHandle(&(i_inst)->super,i_connection) 00083 #define cModFileIoDumy_execute(i_inst,i_connection) NyLPC_cModFileIoBaseClass_execute(&(i_inst)->super,i_connection) 00084 00085 00086 00087 00088 00089 00090 00091 namespace MiMic 00092 { 00093 00094 00095 ModFileIo::ModFileIo(const char* i_path):ModBaseClass(i_path) 00096 { 00097 } 00098 ModFileIo::ModFileIo() 00099 { 00100 } 00101 ModFileIo::~ModFileIo() 00102 { 00103 } 00104 void ModFileIo::setParam(const char* i_path) 00105 { 00106 ModBaseClass::setParam(i_path); 00107 } 00108 bool ModFileIo::execute(HttpdConnection& i_connection) 00109 { 00110 //check parametor 00111 if(this->_path==NULL){ 00112 return false; 00113 } 00114 TcModFileIoDumy mod; 00115 cModFileIoDumy_initialize(&mod,this->_path); 00116 if(cModFileIoDumy_canHandle(&mod,i_connection._ref_inst)){ 00117 cModFileIoDumy_execute(&mod,i_connection._ref_inst); 00118 cModFileIoDumy_finalize(&mod); 00119 return true; 00120 } 00121 cModFileIoDumy_finalize(&mod); 00122 return false; 00123 } 00124 00125 00126 00127 00128 }
Generated on Tue Jul 12 2022 15:46:14 by
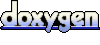