This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
LPC17xx_IAP.c
00001 /* 00002 * LPC17xx_IAP.c 00003 * 00004 * Created on: 2011/10/17 00005 * Author: nyatla 00006 */ 00007 #include "NyLPC_config.h" 00008 #if NyLPC_MCU==NyLPC_MCU_LPC4088 || NyLPC_MCU==NyLPC_MCU_LPC17xx 00009 00010 #include "LPC17xx_IAP.h" 00011 /** 00012 * IPAに通知するCPUクロック。 00013 * ここで指定したクロック以上で動作させないでください。 00014 */ 00015 #define IPA_CPU_FREQ_IN_HZ ( ( unsigned long ) 100000000 ) 00016 #define LPC17xx_FLASH_SECTOR_ADDR_16 ((void*)0x00010000) 00017 00018 //Define data structure or pointers to pass IAP command table and result table to the IAP 00019 #define IAP_LOCATION 0x1FFF1FF1; 00020 /** 00021 * @param command 00022 * 00023 * @param result 00024 */ 00025 typedef void (*_LPCXpresso_IAP_FUNC)(unsigned long command[],unsigned long result[]); 00026 /** 00027 * 00028 */ 00029 _LPCXpresso_IAP_FUNC LPCXpresso_iap_entry=(_LPCXpresso_IAP_FUNC) IAP_LOCATION; 00030 00031 00032 /** 00033 * アドレスをフラッシュメモリのセクタ番号へ変換する。 00034 */ 00035 int LPC17xx_IAP_addr2Sector(const void* addr,unsigned long* o_sector) 00036 { 00037 unsigned long t; 00038 if(addr>=LPC17xx_FLASH_SECTOR_ADDR_16){ 00039 t=((((unsigned long)addr)-((unsigned long)LPC17xx_FLASH_SECTOR_ADDR_16))/0x8000)+16; 00040 if(t>29){ 00041 return LPC17xx_IAP_FALSE;//Error 00042 } 00043 *o_sector=t; 00044 }else{ 00045 *o_sector=((unsigned long)addr)/0x1000; 00046 } 00047 return LPC17xx_IAP_TRUE;//Error 00048 } 00049 /** 00050 * セクタ番号をフラッシュメモリのアドレスへ変換する。 00051 */ 00052 int LPC17xx_IAP_sector2Addr(unsigned int i_sector,void** o_addr) 00053 { 00054 if(i_sector<16){ 00055 *o_addr=(void*)(0x1000*i_sector); 00056 }else if(i_sector>29){ 00057 return LPC17xx_IAP_FALSE; 00058 }else{ 00059 *o_addr=(void*)(((unsigned int)LPC17xx_FLASH_SECTOR_ADDR_16)+(i_sector-16)*0x8000); 00060 } 00061 return LPC17xx_IAP_TRUE; 00062 } 00063 00064 00065 unsigned long LPC17xx_IAP_getSectorSize(unsigned int i_sector) 00066 { 00067 if(i_sector<16){ 00068 return 0x1000; 00069 }else if(i_sector>29){ 00070 return 0; 00071 }else{ 00072 return 0x8000; 00073 } 00074 } 00075 /** 00076 * IAPのprepareコマンドを実行 00077 */ 00078 unsigned long LPC17xx_IAP_prepare(unsigned long i_start,unsigned long i_end) 00079 { 00080 unsigned long b[8]; 00081 unsigned long c[5]; 00082 unsigned long r[5]; 00083 c[0]=50; 00084 c[1]=i_start; 00085 c[2]=i_end; 00086 memcpy(b,(void*)0x10000000,32); 00087 LPCXpresso_iap_entry(c,r); 00088 memcpy((void*)0x10000000,b,32); 00089 return r[0]; 00090 } 00091 /** 00092 * IAPのcopy ram to flashコマンドを実行。 00093 */ 00094 unsigned long LPC17xx_IAP_copyRam2Flash(const void* i_flash_addr,const void* i_src_addr,unsigned long i_size) 00095 { 00096 unsigned long b[8]; 00097 unsigned long c[5]; 00098 unsigned long r[5]; 00099 c[0]=51; 00100 c[1]=(unsigned long)i_flash_addr; 00101 c[2]=(unsigned long)i_src_addr; 00102 c[3]=i_size; 00103 c[4]=IPA_CPU_FREQ_IN_HZ/1000; 00104 memcpy(b,(void*)0x10000000,32); 00105 LPCXpresso_iap_entry(c,r); 00106 memcpy((void*)0x10000000,b,32); 00107 return r[0]; 00108 } 00109 /** 00110 * IAPのeraseコマンドを実行 00111 */ 00112 unsigned long LPC17xx_IAP_erase(unsigned long i_start,unsigned long i_end) 00113 { 00114 unsigned long b[8]; 00115 unsigned long c[5]; 00116 unsigned long r[5]; 00117 c[0]=52; 00118 c[1]=i_start; 00119 c[2]=i_end; 00120 c[3]=IPA_CPU_FREQ_IN_HZ/1000; 00121 memcpy(b,(void*)0x10000000,32); 00122 LPCXpresso_iap_entry(c,r); 00123 memcpy((void*)0x10000000,b,32); 00124 return r[0]; 00125 } 00126 #endif
Generated on Tue Jul 12 2022 15:46:14 by
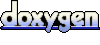