This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
K64F_IAP.h
00001 /** 00002 * Copyright 2014 MiMicProject 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 /** 00016 * This file based on https://mbed.org/users/Sissors/code/FreescaleIAP/ 00017 */ 00018 #ifndef K64F_IAP_h 00019 #define K64F_IAP_h 00020 #include "NyLPC_config.h" 00021 #if NyLPC_MCU==NyLPC_MCU_K64F 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif /* __cplusplus */ 00025 #define K64F_IAP_SECTOR_SIZE 4096 00026 00027 typedef int K64F_IAP_TIAPCode; 00028 00029 #define K64F_IAP_TIAPCode_BoundaryError -99 //Commands may not span several sectors 00030 #define K64F_IAP_TIAPCode_AlignError -98 //Data must be aligned on longword (two LSBs zero) 00031 #define K64F_IAP_TIAPCode_ProtectionError -97 //Flash sector is protected 00032 #define K64F_IAP_TIAPCode_AccessError -96 //Something went wrong 00033 #define K64F_IAP_TIAPCode_CollisionError -95 //During writing something tried to flash which was written to 00034 #define K64F_IAP_TIAPCode_LengthError -94 //The length must be multiples of 4 00035 #define K64F_IAP_TIAPCode_RuntimeError -93 00036 #define K64F_IAP_TIAPCode_EraseError -92 //The flash was not erased before writing to it 00037 #define K64F_IAP_TIAPCode_Success 0 00038 00039 00040 /** Erase a flash sector 00041 * 00042 * The size erased depends on the used device 00043 * 00044 * @param address address in the sector which needs to be erased 00045 * @param return Success if no errors were encountered, otherwise one of the error states 00046 */ 00047 K64F_IAP_TIAPCode K64F_IAP_erase_sector(int address); 00048 00049 /** Program flash 00050 * 00051 * Before programming the used area needs to be erased. The erase state is checked 00052 * before programming, and will return an error if not erased. 00053 * 00054 * @param address starting address where the data needs to be programmed (must be longword alligned: two LSBs must be zero) 00055 * @param data pointer to array with the data to program 00056 * @param length number of bytes to program (must be a multiple of 4. must be a multiple of 8 when K64F) 00057 * @param return Success if no errors were encountered, otherwise one of the error states 00058 */ 00059 K64F_IAP_TIAPCode K64F_IAP_program_flash(int address, char *data, unsigned int length); 00060 00061 /** 00062 * Returns size of flash memory 00063 * 00064 * This is the first address which is not flash 00065 * 00066 * @param return length of flash memory in bytes 00067 */ 00068 unsigned int K64F_IAP_flash_size(void); 00069 #ifdef __cplusplus 00070 } 00071 #endif /* __cplusplus */ 00072 #endif 00073 #endif
Generated on Tue Jul 12 2022 15:46:14 by
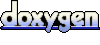