This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
HttpdConnection.h
00001 #pragma once 00002 //////////////////////////////////////////////////////////////////////////////// 00003 // HttpdConnection.h 00004 //////////////////////////////////////////////////////////////////////////////// 00005 00006 #include "NyLPC_net.h" 00007 namespace MiMic 00008 { 00009 class HttpdConnection 00010 { 00011 public: 00012 NyLPC_TcHttpdConnection* _ref_inst; 00013 HttpdConnection(NyLPC_TcHttpdConnection* i_ref_inst); 00014 /** 00015 * This function returns HTTP request type. 00016 * See Http::MT_xxx constant value. 00017 */ 00018 int getMethodType(); 00019 00020 /** 00021 * This function checks Method type if equal with i_method_type. 00022 */ 00023 bool isMethodType(int i_method_type); 00024 00025 /** 00026 * This function send HTTP response header to connection. 00027 * The function is useful for unknown length content. 00028 * @param i_additional_header 00029 * Additional header text. 00030 * The text which was divided in CRLF and closed by CRLF. 00031 */ 00032 bool sendHeader(unsigned short i_status_code,const char* i_content_type,const char* i_additional_header); 00033 00034 /** 00035 * This function send HTTP response header to connection. 00036 * The function is useful for known length content. 00037 */ 00038 bool sendHeader(unsigned short i_status_code,const char* i_content_type,const char* i_additional_header,unsigned int i_length); 00039 /** 00040 * This function send HTTP error response with empty body. 00041 */ 00042 bool sendError(unsigned short i_status_code); 00043 00044 /** 00045 * This function send formated text to response. 00046 * The function can be repeatedly called until the end of contents. 00047 */ 00048 bool sendBody(const void* i_data,NyLPC_TUInt32 i_size); 00049 00050 /** 00051 * The function send formated text to response. 00052 * The function can be repeatedly called until the end of contents. 00053 * @param i_fmt 00054 * printf like string 00055 * %% - '%' charactor ,%s - null terminated string ,%d - 32bit signed integer,%x - 32bit intager ,%u - 32bit unsigned integer ,%c - a charactor 00056 * @param ... 00057 * 00058 */ 00059 bool sendBodyF(const char* i_fmt,...); 00060 /** 00061 * The function gets httpd lock. 00062 * This lock is the only lock in the Httpd. 00063 * After call the function, must call unlock function by end of handler. 00064 */ 00065 void lockHttpd(); 00066 /** 00067 * The function releases the httpd lock. 00068 */ 00069 void unlockHttpd(); 00070 /** 00071 * The function breaks the persist connection if it enabled. 00072 */ 00073 void breakPersistentConnection(); 00074 00075 }; 00076 }
Generated on Tue Jul 12 2022 15:46:14 by
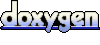