This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
HttpdConnection.cpp
00001 #include "HttpdConnection.h" 00002 namespace MiMic 00003 { 00004 00005 int HttpdConnection::getMethodType() 00006 { 00007 return NyLPC_cHttpdConnection_getMethod(this->_ref_inst); 00008 } 00009 00010 bool HttpdConnection::isMethodType(int i_method_type) 00011 { 00012 return NyLPC_cHttpdConnection_getMethod(this->_ref_inst)==i_method_type; 00013 } 00014 00015 HttpdConnection::HttpdConnection(NyLPC_TcHttpdConnection* i_ref_inst) 00016 { 00017 this->_ref_inst=i_ref_inst; 00018 } 00019 bool HttpdConnection::sendError(unsigned short i_status_code) 00020 { 00021 return NyLPC_TBool_TRUE==NyLPC_cHttpdConnection_sendResponseHeader2(this->_ref_inst,i_status_code,"text/html",0,NULL); 00022 } 00023 bool HttpdConnection::sendHeader(unsigned short i_status_code,const char* i_content_type,const char* i_additional_header) 00024 { 00025 return NyLPC_TBool_TRUE==NyLPC_cHttpdConnection_sendResponseHeader(this->_ref_inst,i_status_code,i_content_type,i_additional_header); 00026 } 00027 bool HttpdConnection::sendHeader(unsigned short i_status_code,const char* i_content_type,const char* i_additional_header,unsigned int i_length) 00028 { 00029 return NyLPC_TBool_TRUE==NyLPC_cHttpdConnection_sendResponseHeader2(this->_ref_inst,i_status_code,i_content_type,i_length,i_additional_header); 00030 } 00031 bool HttpdConnection::sendBody(const void* i_data,NyLPC_TUInt32 i_size) 00032 { 00033 return NyLPC_TBool_TRUE==NyLPC_cHttpdConnection_sendResponseBody(this->_ref_inst,i_data,i_size); 00034 } 00035 bool HttpdConnection::sendBodyF(const char* i_fmt,...) 00036 { 00037 va_list a; 00038 if(this->_ref_inst->_res_status!=NyLPC_cHttpdConnection_ResStatus_BODY) 00039 { 00040 NyLPC_OnErrorGoto(Error); 00041 } 00042 //Bodyの書込み 00043 va_start(a,i_fmt); 00044 if(!NyLPC_cHttpBodyWriter_formatV(&(this->_ref_inst->_body_writer),i_fmt,a)){ 00045 NyLPC_OnErrorGoto(Error_Send); 00046 } 00047 va_end(a); 00048 return true; 00049 Error_Send: 00050 va_end(a); 00051 NyLPC_cHttpBodyWriter_finalize(&(this->_ref_inst->_in_stream)); 00052 Error: 00053 this->_ref_inst->_res_status=NyLPC_cHttpdConnection_ResStatus_ERROR; 00054 return false; 00055 } 00056 void HttpdConnection::lockHttpd() 00057 { 00058 NyLPC_cHttpdConnection_lock(this->_ref_inst); 00059 } 00060 void HttpdConnection::unlockHttpd() 00061 { 00062 NyLPC_cHttpdConnection_unlock(this->_ref_inst); 00063 } 00064 void HttpdConnection::breakPersistentConnection() 00065 { 00066 NyLPC_cHttpdConnection_setConnectionMode(this->_ref_inst,NyLPC_TcHttpdConnection_CONNECTION_MODE_CLOSE); 00067 } 00068 }
Generated on Tue Jul 12 2022 15:46:14 by
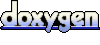