This is Webservice SDK for mbed. LPCXpresso1769/LPC1768/FRDM-K64F/LPC4088
Dependents: MbedFileServer_1768MiniDK2 RedWireBridge IssueDebug_gcc MiMicRemoteMCU-for-Mbed ... more
HttpClient.cpp
00001 #include "HttpClient.h" 00002 00003 namespace MiMic 00004 { 00005 HttpClient::HttpClient() 00006 { 00007 this->_private_tcp_rx_buf=malloc(256); 00008 NyLPC_cHttpClient_initialize(&this->_inst,this->_private_tcp_rx_buf,256); 00009 } 00010 HttpClient::~HttpClient() 00011 { 00012 NyLPC_cHttpClient_finalize(&this->_inst); 00013 if(this->_private_tcp_rx_buf!=NULL){ 00014 free(this->_private_tcp_rx_buf); 00015 } 00016 } 00017 bool HttpClient::connect(const IpAddr& i_host,unsigned short i_port) 00018 { 00019 return NyLPC_cHttpClient_connect(&this->_inst,&i_host.addr.v4,i_port)?true:false; 00020 } 00021 /** 00022 * This function sends a request to server and prevent to accept status code. 00023 * Must call getStatus after successful. 00024 * If request has content body(i_content_length!=0), call writeX function to send request body in before to call getStatus 00025 * @return 00026 * true if successful, 00027 * otherwise error. Connection is closed. 00028 * @example 00029 * <code> 00030 * //GET 00031 * </code> 00032 * <code> 00033 * //POST 00034 * 00035 * </code> 00036 */ 00037 bool HttpClient::sendMethod(NyLPC_THttpMethodType i_method,const char* i_path,int i_content_length,const char* i_mimetype,const char* i_additional_header) 00038 { 00039 return NyLPC_cHttpClient_sendMethod(&this->_inst,i_method,i_path,i_content_length,i_mimetype,i_additional_header)?true:false; 00040 } 00041 /** 00042 * This function returns status code. 00043 * Must call after the sendMethod was successful. 00044 * @return 00045 * Error:0,otherwise HTTP status code. 00046 */ 00047 int HttpClient::getStatus() 00048 { 00049 return NyLPC_cHttpClient_getStatus(&this->_inst); 00050 } 00051 /** 00052 * Close current connection. 00053 */ 00054 void HttpClient::close() 00055 { 00056 NyLPC_cHttpClient_close(&this->_inst); 00057 } 00058 /** 00059 * Read request body from http stream. 00060 * @param i_rx_buf 00061 * A buffer which accepts received data. 00062 * @param i_rx_buf_len 00063 * size of i_rx_buf in byte. 00064 * @param i_read_len 00065 * pointer to variable which accept received data size in byte. 00066 * It is enabled in retrurn true. 00067 * n>0 is datasize. n==0 is end of stream. 00068 * @return 00069 * true if successful,otherwise false 00070 */ 00071 bool HttpClient::read(void* i_rx_buf,int i_rx_buf_len,short &i_read_len) 00072 { 00073 return NyLPC_cHttpClient_read(&this->_inst,i_rx_buf,i_rx_buf_len,&i_read_len)?true:false; 00074 } 00075 bool HttpClient::write(const void* i_tx_buf,int i_tx_len) 00076 { 00077 return NyLPC_cHttpClient_write(&this->_inst,i_tx_buf,i_tx_len)?true:false; 00078 } 00079 00080 }
Generated on Tue Jul 12 2022 15:46:14 by
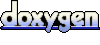