
see http://mbed.org/users/no2chem/notebook/mbed-clock-control--benchmarks/
Embed:
(wiki syntax)
Show/hide line numbers
coremark.h
00001 /* 00002 Author : Shay Gal-On, EEMBC 00003 00004 This file is part of EEMBC(R) and CoreMark(TM), which are Copyright (C) 2009 00005 All rights reserved. 00006 00007 EEMBC CoreMark Software is a product of EEMBC and is provided under the terms of the 00008 CoreMark License that is distributed with the official EEMBC COREMARK Software release. 00009 If you received this EEMBC CoreMark Software without the accompanying CoreMark License, 00010 you must discontinue use and download the official release from www.coremark.org. 00011 00012 Also, if you are publicly displaying scores generated from the EEMBC CoreMark software, 00013 make sure that you are in compliance with Run and Reporting rules specified in the accompanying readme.txt file. 00014 00015 EEMBC 00016 4354 Town Center Blvd. Suite 114-200 00017 El Dorado Hills, CA, 95762 00018 */ 00019 /* Topic: Description 00020 This file contains declarations of the various benchmark functions. 00021 */ 00022 00023 /* Configuration: TOTAL_DATA_SIZE 00024 Define total size for data algorithms will operate on 00025 */ 00026 #ifndef TOTAL_DATA_SIZE 00027 #define TOTAL_DATA_SIZE 2*1000 00028 #endif 00029 00030 #define SEED_ARG 0 00031 #define SEED_FUNC 1 00032 #define SEED_VOLATILE 2 00033 00034 #define MEM_STATIC 0 00035 #define MEM_MALLOC 1 00036 #define MEM_STACK 2 00037 00038 #include "core_portme.h" 00039 00040 #if HAS_STDIO 00041 #include <stdio.h> 00042 #endif 00043 #if HAS_PRINTF 00044 #define ee_printf printf 00045 #endif 00046 00047 /* Actual benchmark execution in iterate */ 00048 void *iterate(void *pres); 00049 00050 /* Typedef: secs_ret 00051 For machines that have floating point support, get number of seconds as a double. 00052 Otherwise an unsigned int. 00053 */ 00054 #if HAS_FLOAT 00055 typedef double secs_ret; 00056 #else 00057 typedef ee_u32 secs_ret; 00058 #endif 00059 00060 #if MAIN_HAS_NORETURN 00061 #define MAIN_RETURN_VAL 00062 #define MAIN_RETURN_TYPE void 00063 #else 00064 #define MAIN_RETURN_VAL 0 00065 #define MAIN_RETURN_TYPE int 00066 #endif 00067 00068 void start_time(void); 00069 void stop_time(void); 00070 CORE_TICKS get_time(void); 00071 secs_ret time_in_secs(CORE_TICKS ticks); 00072 00073 /* Misc useful functions */ 00074 ee_u16 crcu8(ee_u8 data, ee_u16 crc); 00075 ee_u16 crc16(ee_s16 newval, ee_u16 crc); 00076 ee_u16 crcu16(ee_u16 newval, ee_u16 crc); 00077 ee_u16 crcu32(ee_u32 newval, ee_u16 crc); 00078 ee_u8 check_data_types(); 00079 void *portable_malloc(ee_size_t size); 00080 void portable_free(void *p); 00081 ee_s32 parseval(char *valstring); 00082 00083 /* Algorithm IDS */ 00084 #define ID_LIST (1<<0) 00085 #define ID_MATRIX (1<<1) 00086 #define ID_STATE (1<<2) 00087 #define ALL_ALGORITHMS_MASK (ID_LIST|ID_MATRIX|ID_STATE) 00088 #define NUM_ALGORITHMS 3 00089 00090 /* list data structures */ 00091 typedef struct list_data_s { 00092 ee_s16 data16; 00093 ee_s16 idx; 00094 } list_data; 00095 00096 typedef struct list_head_s { 00097 struct list_head_s *next; 00098 struct list_data_s *info; 00099 } list_head; 00100 00101 00102 /*matrix benchmark related stuff */ 00103 #define MATDAT_INT 1 00104 #if MATDAT_INT 00105 typedef ee_s16 MATDAT; 00106 typedef ee_s32 MATRES; 00107 #else 00108 typedef ee_f16 MATDAT; 00109 typedef ee_f32 MATRES; 00110 #endif 00111 00112 typedef struct MAT_PARAMS_S { 00113 int N; 00114 MATDAT *A; 00115 MATDAT *B; 00116 MATRES *C; 00117 } mat_params; 00118 00119 /* state machine related stuff */ 00120 /* List of all the possible states for the FSM */ 00121 typedef enum CORE_STATE { 00122 CORE_START=0, 00123 CORE_INVALID, 00124 CORE_S1, 00125 CORE_S2, 00126 CORE_INT, 00127 CORE_FLOAT, 00128 CORE_EXPONENT, 00129 CORE_SCIENTIFIC, 00130 NUM_CORE_STATES 00131 } core_state_e ; 00132 00133 00134 /* Helper structure to hold results */ 00135 typedef struct RESULTS_S { 00136 /* inputs */ 00137 ee_s16 seed1; /* Initializing seed */ 00138 ee_s16 seed2; /* Initializing seed */ 00139 ee_s16 seed3; /* Initializing seed */ 00140 void *memblock[4]; /* Pointer to safe memory location */ 00141 ee_u32 size; /* Size of the data */ 00142 ee_u32 iterations; /* Number of iterations to execute */ 00143 ee_u32 execs; /* Bitmask of operations to execute */ 00144 struct list_head_s *list; 00145 mat_params mat; 00146 /* outputs */ 00147 ee_u16 crc; 00148 ee_u16 crclist; 00149 ee_u16 crcmatrix; 00150 ee_u16 crcstate; 00151 ee_s16 err; 00152 /* ultithread specific */ 00153 core_portable port; 00154 } core_results; 00155 00156 /* Multicore execution handling */ 00157 #if (MULTITHREAD>1) 00158 ee_u8 core_start_parallel(core_results *res); 00159 ee_u8 core_stop_parallel(core_results *res); 00160 #endif 00161 00162 /* list benchmark functions */ 00163 list_head *core_list_init(ee_u32 blksize, list_head *memblock, ee_s16 seed); 00164 ee_u16 core_bench_list(core_results *res, ee_s16 finder_idx); 00165 00166 /* state benchmark functions */ 00167 void core_init_state(ee_u32 size, ee_s16 seed, ee_u8 *p); 00168 ee_u16 core_bench_state(ee_u32 blksize, ee_u8 *memblock, 00169 ee_s16 seed1, ee_s16 seed2, ee_s16 step, ee_u16 crc); 00170 00171 /* matrix benchmark functions */ 00172 ee_u32 core_init_matrix(ee_u32 blksize, void *memblk, ee_s32 seed, mat_params *p); 00173 ee_u16 core_bench_matrix(mat_params *p, ee_s16 seed, ee_u16 crc); 00174
Generated on Fri Jul 15 2022 12:36:20 by
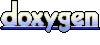