This is a port of the Adafruit driver and DateTime library for the DS1307 RTC controller
Dependents: espresso-machine-control SAT_OLED Mbedesclava Mbedmaestra ... more
DS1307.h
00001 // Code by JeeLabs http://news.jeelabs.org/code/ 00002 // Released to the public domain! Enjoy! 00003 00004 /* 00005 * Taken from https://github.com/adafruit/RTClib 00006 * and modified for LPC1768 by Neal Horman July 2012 00007 * Also add support for access to the 56 bytes of 00008 * user accessible battery backed ram. 00009 */ 00010 00011 #ifndef _DS1307_H_ 00012 #define _DS1307_H_ 00013 00014 #include "mbed.h" 00015 #include "DateTime.h" 00016 00017 // RTC based on the DS1307 chip connected via I2C 00018 class RtcDs1307 00019 { 00020 public: 00021 RtcDs1307(I2C &i2c); 00022 bool adjust(const DateTime& dt); 00023 bool isRunning(); 00024 DateTime now(); 00025 bool commit(); 00026 uint8_t &operator[](uint8_t i) { return mRam[(i<sizeof(mRam)-1 ? i+1 : 0)]; }; 00027 protected: 00028 I2C &mI2c; 00029 uint8_t mRam[1+56]; // device register address + 56 bytes 00030 }; 00031 00032 #endif
Generated on Fri Jul 15 2022 02:15:33 by
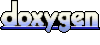