IC Eurobot 2012 Program published from Shuto's account
Dependents: Eurobot2012_Primary
RtosTimer.h
00001 /* Copyright (c) 2012 mbed.org */ 00002 #ifndef TIMER_H 00003 #define TIMER_H 00004 00005 #include <stdint.h> 00006 #include "cmsis_os.h" 00007 00008 namespace rtos { 00009 00010 /*! The RtosTimer class allow creating and and controlling of timer functions in the system. 00011 A timer function is called when a time period expires whereby both on-shot and 00012 periodic timers are possible. A timer can be started, restarted, or stopped. 00013 00014 Timers are handled in the thread osTimerThread. 00015 Callback functions run under control of this thread and may use CMSIS-RTOS API calls. 00016 */ 00017 class RtosTimer { 00018 public: 00019 /*! Create and Start timer. 00020 \param task name of the timer call back function. 00021 \param type osTimerOnce for one-shot or osTimerPeriodic for periodic behaviour. (default: osTimerPeriodic) 00022 \param argument argument to the timer call back function. (default: NULL) 00023 */ 00024 RtosTimer (void (*task)(void const *argument), 00025 os_timer_type type=osTimerPeriodic, 00026 void *argument=NULL); 00027 00028 /*! Stop the timer. 00029 \return status code that indicates the execution status of the function. 00030 */ 00031 osStatus stop (void); 00032 00033 /*! start a timer. 00034 \param millisec time delay value of the timer. 00035 \return status code that indicates the execution status of the function. 00036 */ 00037 osStatus start (uint32_t millisec); 00038 00039 private: 00040 osTimerId _timer_id; 00041 osTimerDef_t _timer; 00042 #ifdef CMSIS_OS_RTX 00043 uint32_t _timer_data[5]; 00044 #endif 00045 }; 00046 00047 } 00048 00049 #endif
Generated on Tue Jul 12 2022 21:26:54 by
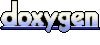