Myserial Library extends RawSerial
Embed:
(wiki syntax)
Show/hide line numbers
MySerial.h
00001 /** mbed Serial Library extend RawSerial 00002 * Copyright (c) 2014 Naoki Okino 00003 * 00004 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00005 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00006 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00007 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00008 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00009 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00010 * THE SOFTWARE. 00011 */ 00012 #ifndef MBED_MYSERIAL_H 00013 #define MBED_MYSERIAL_H 00014 00015 #include "mbed.h" 00016 00017 /** MySerial control class, based on a RawSerial 00018 * 00019 * Example: 00020 * @code 00021 * #include "mbed.h" 00022 * #include "MySerial.h" 00023 * 00024 * MySerial pc(USBTX,USBRX); //instance of MySerial Class 00025 * char cWord[16]; //receive chars or cWord[256], cWord[1024] etc.. 00026 * int readSize = 15; 00027 * 00028 * void readbuf() 00029 * { 00030 * if (readSize >= sizeof(cWord)) { readSize = sizeof(cWord)-1; } 00031 * // int iRtn = pc.GetString(readSize,&cWord); //Serial received chars by pointer cWord 00032 * int iRtn = pc.GetString(readSize,cWord); //Serial received chars byref of cWord 00033 * } 00034 * int main() { 00035 * pc.baud(9600); //set baud rate 00036 * pc.format(8, MySerial::None, 1);//set bits for a byte, parity bit, stop bit 00037 * pc.SetRxWait(0.01, 0.001); //set wait getting chars after interrupted, each char 00038 * pc.attach( readbuf, MySerial::RxIrq ); //Set Interrupt by Serial receive 00039 * } 00040 * @endcode 00041 */ 00042 class MySerial : public Serial{ 00043 00044 public: 00045 00046 /** constructor to get chars received by serial 00047 * 00048 * @param PinName tx 00049 * @param PinName rx 00050 */ 00051 MySerial(PinName tx, PinName rx); 00052 00053 /** set wait getting chars after interrupted 00054 * 00055 * @param float _fRxStartWait wait getting a 1st char after interrupted 00056 * @param float _fRxEachWait wait getting each char 00057 */ 00058 void SetRxWait(float _fRxStartWait, float _fRxEachWait); 00059 00060 /** function to get chars after received chars by serial 00061 * 00062 * @param int size for get chars 00063 * @param *cWord returns got chars by pointer 00064 * @param returns success by 0 00065 */ 00066 virtual int GetString(int size, char *cWord); 00067 00068 /** overload function to get chars after received chars by serial 00069 * 00070 * @param int size for get chars 00071 * @param cWord returns got chars by ref 00072 * @param returns success by 0 00073 */ 00074 template <class X> int GetString(int size, X cWord) 00075 { 00076 return GetString(size, &cWord); 00077 } 00078 00079 protected: 00080 float fRxStartWait; 00081 float fRxEachWait; 00082 00083 }; /* class MySerial */ 00084 00085 #endif
Generated on Thu Jul 14 2022 03:52:59 by
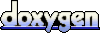