Driver for thermal printer using serial communication like http://www.sparkfun.com/products/10438. This is port from arduino library available at http://bildr.org/2011/08/thermal-printer-arduino/
Dependents: ThermalSample mbedica_on_yehowshua mbedica
Thermal.cpp
00001 /* 00002 Copyright (c) 2010 bildr community 00003 Ported to mbed by mimilowns 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "Thermal.h" 00026 00027 00028 Thermal::Thermal(PinName tx, PinName rx, int baud) : Serial(tx, rx) { 00029 Serial::baud(baud); 00030 //setTimeout(50); 00031 00032 00033 int zero = 0; 00034 00035 00036 00037 heatTime = 80; // 80 is default from page 23 of datasheet. Controls speed of printing and darkness 00038 heatInterval = 2; // 2 is default from page 23 of datasheet. Controls speed of printing and darkness 00039 printDensity = 15; // Not sure what the defaut is. Testing shows the max helps darken text. From page 23. 00040 printBreakTime = 15; // Not sure what the defaut is. Testing shows the max helps darken text. From page 23. 00041 00042 00043 setHeatTime(heatTime); 00044 setPrintDensity(printDensity); 00045 00046 00047 setDefault(); 00048 } 00049 00050 void Thermal::setDefault() 00051 00052 { 00053 wake(); 00054 justify('L'); 00055 inverseOff(); 00056 doubleHeightOff(); 00057 setLineHeight(32); 00058 boldOff(); 00059 underlineOff(); 00060 setBarcodeHeight(50); 00061 setSize('s'); 00062 } 00063 00064 void Thermal::test() 00065 00066 { 00067 Serial::printf("Hello World!"); 00068 feed(2); 00069 } 00070 00071 00072 00073 void Thermal::setBarcodeHeight(int val) 00074 00075 { 00076 // default is 50 00077 writeBytes(29, 104, val); 00078 } 00079 00080 void Thermal::printBarcode(char * text){ 00081 writeBytes(29, 107, 0); // GS, K, m! 00082 00083 for(int i = 0; i < strlen(text); i ++){ 00084 write(text[i]); //Data 00085 } 00086 00087 write(zero); //Terminator 00088 00089 delay(3000); //For some reason we can't immediately have line feeds here 00090 feed(2); 00091 } 00092 00093 void Thermal::printFancyBarcode(char * text){ 00094 writeBytes(29, 107, 4); // GS, K, Fancy! 00095 00096 for(int i = 0; i < strlen(text); i ++){ 00097 write(text[i]); //Data 00098 } 00099 00100 write(zero); //Terminator 00101 00102 delay(3000); //For some reason we can't immediately have line feeds here 00103 feed(2); 00104 } 00105 00106 void Thermal::writeBytes(uint8_t a, uint8_t b) 00107 00108 { 00109 write(a); 00110 write(b); 00111 } 00112 00113 void Thermal::writeBytes(uint8_t a, uint8_t b, uint8_t c) 00114 00115 { 00116 write(a); 00117 write(b); 00118 write(c); 00119 } 00120 00121 void Thermal::writeBytes(uint8_t a, uint8_t b, uint8_t c, uint8_t d) 00122 00123 { 00124 write(a); 00125 write(b); 00126 write(c); 00127 write(d); 00128 } 00129 00130 void Thermal::write(uint8_t a) 00131 { 00132 putc(a); 00133 } 00134 00135 void Thermal::delay(uint8_t a) 00136 { 00137 wait(a); 00138 } 00139 00140 void Thermal::inverseOn() 00141 00142 { 00143 writeBytes(29, 'B', 1); 00144 } 00145 00146 void Thermal::inverseOff() 00147 00148 { 00149 writeBytes(29, 'B', 0, 10); 00150 } 00151 00152 void Thermal::doubleHeightOn() 00153 00154 { 00155 writeBytes(27, 14); 00156 } 00157 00158 void Thermal::doubleHeightOff() 00159 00160 { 00161 writeBytes(27, 20); 00162 } 00163 00164 void Thermal::boldOn() 00165 00166 { 00167 writeBytes(27, 69, 1); 00168 } 00169 00170 void Thermal::boldOff() 00171 00172 { 00173 writeBytes(27, 69, 0); 00174 if (linefeedneeded) 00175 feed(); 00176 00177 linefeedneeded = false; 00178 } 00179 00180 void Thermal::justify(char value) 00181 00182 { 00183 uint8_t pos = 0; 00184 00185 00186 if(value == 'l' || value == 'L') pos = 0; 00187 if(value == 'c' || value == 'C') pos = 1; 00188 if(value == 'r' || value == 'R') pos = 2; 00189 00190 writeBytes(0x1B, 0x61, pos); 00191 } 00192 00193 void Thermal::feed(uint8_t x) 00194 00195 { 00196 while (x--) 00197 write(10); 00198 } 00199 00200 void Thermal::setSize(char value) 00201 00202 { 00203 int size = 0; 00204 00205 00206 if(value == 's' || value == 'S') size = 0; 00207 if(value == 'm' || value == 'M') size = 10; 00208 if(value == 'l' || value == 'L') size = 25; 00209 00210 writeBytes(29, 33, size, 10); 00211 // if (linefeedneeded) 00212 // println("lfn"); //feed(); 00213 //linefeedneeded = false; 00214 } 00215 00216 void Thermal::underlineOff() 00217 00218 { 00219 writeBytes(27, 45, 0, 10); 00220 } 00221 00222 00223 void Thermal::underlineOn() 00224 00225 { 00226 writeBytes(27, 45, 1); 00227 } 00228 00229 void Thermal::printBitmap(uint8_t w, uint8_t h, const uint8_t *bitmap) 00230 00231 { 00232 writeBytes(18, 42, h, w/8); 00233 for (uint16_t i=0; i<(w/8) * h; i++) { 00234 //write(pgm_read_byte(bitmap + i)); 00235 write(*(bitmap + i)); 00236 } 00237 } 00238 00239 void Thermal::wake() 00240 00241 { 00242 writeBytes(27, 61, 1); 00243 } 00244 00245 void Thermal::sleep() 00246 00247 { 00248 writeBytes(27, 61, 0); 00249 } 00250 00251 ////////////////////// not working? 00252 void Thermal::tab() 00253 00254 { 00255 write(9); 00256 } 00257 00258 00259 void Thermal::setCharSpacing(int spacing) 00260 00261 { 00262 writeBytes(27, 32, 0, 10); 00263 } 00264 00265 00266 void Thermal::setLineHeight(int val) 00267 00268 { 00269 writeBytes(27, 51, val); // default is 32 00270 } 00271 00272 00273 00274 void Thermal::setHeatTime(int vHeatTime) 00275 00276 { 00277 00278 heatTime = vHeatTime; 00279 00280 write(27); 00281 00282 write(55); 00283 00284 write(7); //Default 64 dots = 8*('7'+1) 00285 00286 write(heatTime); //Default 80 or 800us 00287 00288 write(heatInterval); //Default 2 or 20us 00289 00290 } 00291 00292 00293 00294 void Thermal::setHeatInterval(int vHeatInterval) 00295 00296 { 00297 00298 heatInterval = vHeatInterval; 00299 00300 write(27); 00301 00302 write(55); 00303 00304 write(7); //Default 64 dots = 8*('7'+1) 00305 00306 write(heatTime); //Default 80 or 800us 00307 00308 write(heatInterval); //Default 2 or 20us 00309 00310 } 00311 00312 00313 00314 void Thermal::setPrintDensity(char vPrintDensity) 00315 00316 { 00317 00318 //Modify the print density and timeout 00319 00320 printDensity = vPrintDensity; 00321 00322 write(18); 00323 00324 write(35); 00325 00326 int printSetting = (printDensity<<4) | printBreakTime; 00327 00328 write(printSetting); //Combination of printDensity and printBreakTime 00329 00330 } 00331 00332 00333 00334 void Thermal::setPrintBreakTime(char vPrintBreakTime) 00335 00336 { 00337 00338 //Modify the print density and timeout 00339 00340 printBreakTime = vPrintBreakTime; 00341 00342 write(18); 00343 00344 write(35); 00345 00346 int printSetting = (printDensity<<4) | printBreakTime; 00347 00348 write(printSetting); //Combination of printDensity and printBreakTime 00349 00350 } 00351
Generated on Mon Jul 18 2022 10:36:05 by
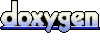